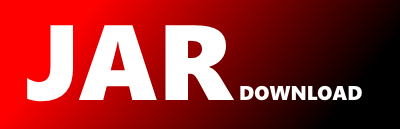
org.ow2.frascati.remote.introspection.RemoteScaDomainImpl Maven / Gradle / Ivy
The newest version!
/***
* OW2 FraSCAti Introspection
* Copyright (C) 2008-2011 INRIA, University of Lille 1
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*
* Authors: Christophe Demarey
*/
package org.ow2.frascati.remote.introspection;
import java.io.BufferedOutputStream;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.Reader;
import java.io.StringReader;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Set;
import java.util.StringTokenizer;
import java.util.logging.Level;
import java.util.logging.Logger;
import javax.script.ScriptException;
import org.apache.cxf.common.util.Base64Exception;
import org.apache.cxf.common.util.Base64Utility;
import org.objectweb.fractal.api.NoSuchInterfaceException;
import org.objectweb.fractal.api.control.ContentController;
import org.objectweb.fractal.fscript.jsr223.InvocableScriptEngine;
import org.objectweb.fractal.util.ContentControllerHelper;
import org.objectweb.fractal.util.Fractal;
import org.osoa.sca.annotations.Reference;
import org.osoa.sca.annotations.Scope;
import org.ow2.frascati.assembly.factory.api.CompositeManager;
import org.ow2.frascati.assembly.factory.api.ManagerException;
import org.ow2.frascati.fscript.model.ScaComponentNode;
import org.ow2.frascati.fscript.model.ScaReferenceNode;
import org.ow2.frascati.fscript.model.ScaServiceNode;
import org.ow2.frascati.remote.introspection.resources.Component;
import org.ow2.frascati.remote.introspection.resources.Node;
import org.ow2.frascati.remote.introspection.resources.Port;
/**
* This SCA component is used to expose / introspect an SCA domain
* remotely.
*/
@Scope("COMPOSITE")
public class RemoteScaDomainImpl implements RemoteScaDomain, Reconfiguration, Deployment {
/** A reference to the FraSCAti Domain */
@Reference(name="frascati-domain")
protected CompositeManager compositeManager;
/** A reference to the FraSCAti FScript engine */
@Reference(name="fscript-engine")
protected InvocableScriptEngine engine;
/** The logger */
public final static Logger log = Logger.getLogger("org.ow2.frascati.remote.introspection.RemoteScaDomain");
/** Default constructor. */
public RemoteScaDomainImpl() { }
// Private methods
/**
* Get a reference to the fractal component designated by id.
*
* @param id The full component id (ex: /a/b/d)
* @return the reference to the fractal component designated by id or null if not found.
*/
protected org.objectweb.fractal.api.Component getFractalComponent(String id) {
StringTokenizer st = new StringTokenizer(id, "/");
org.objectweb.fractal.api.Component current = null;
if (st.hasMoreTokens()) {
String parentName = st.nextToken();
log.info("Trying to get a reference to the '" + parentName + "' component");
try {
current = compositeManager.getComposite(parentName);
} catch (ManagerException e) {
log.warning("Cannot retrieve the '" + parentName + "' component");
return null;
}
while ( st.hasMoreTokens() && (current != null) ) {
String name = st.nextToken();
current = ContentControllerHelper.getSubComponentByName(current, name);
}
}
return current;
}
/**
* Map FraSCAti Script nodes to serializable resources.
*
* @param obj The result of an FScript evaluation : nothing, a node or a list of nodes
* @return A serializable resource if the type is known, else the parameter itself.
*/
protected Node getRessoureFor(Object obj) {
if (obj instanceof ScaComponentNode) {
return ResourceUtil.getFullComponentResource( ((ScaComponentNode) obj).getComponent() );
} if (obj instanceof ScaServiceNode) {
return ResourceUtil.getPortResource( ((ScaServiceNode) obj).getInterface() );
} if (obj instanceof ScaReferenceNode) {
return ResourceUtil.getPortResource( ((ScaReferenceNode) obj).getInterface() );
}
Node node = new Node();
if (obj != null)
node.setName( obj.toString() );
return node;
}
// Public Methods
// Implementation of the RemoteScaDomain interface
/**
* @see RemoteScaDomain#getComposites()
*/
public Collection getDomainComposites() {
Collection components = new ArrayList();
for (org.objectweb.fractal.api.Component composite : compositeManager.getComposites() ) {
components.add( ResourceUtil.getFullComponentResource(composite) );
}
return components;
}
/**
* @see RemoteScaDomain#getComponent(String)
*/
public Component getComponent(String id) {
org.objectweb.fractal.api.Component comp = getFractalComponent(id);
return ResourceUtil.getFullComponentResource(comp);
}
/**
* @see RemoteScaDomain#getInterface(String)
*/
public Port getInterface(String id) {
String itfName = id.substring(id.lastIndexOf('/') + 1);
String compId = id.substring(0, id.lastIndexOf('/'));
org.objectweb.fractal.api.Component comp = getFractalComponent(compId);
org.objectweb.fractal.api.Interface itf = null;
try {
itf = (org.objectweb.fractal.api.Interface) comp.getFcInterface(itfName);
} catch (NoSuchInterfaceException e) {
return null;
}
return ResourceUtil.getPortResource(itf);
}
/**
* @see RemoteScaDomain#getSubComponents(String)
*/
public Collection getSubComponents(String id) {
Collection children = new ArrayList();
org.objectweb.fractal.api.Component parent = null;
ContentController cc = null;
parent = getFractalComponent(id);
try {
cc = Fractal.getContentController(parent);
} catch (NoSuchInterfaceException e) {
log.warning("Cannot find a content controller on " + id);
return children;
}
for (org.objectweb.fractal.api.Component child: cc.getFcSubComponents()) {
children.add( ResourceUtil.getComponentResource(child) );
}
return children;
}
// Implementation of the Reconfiguration interface
/**
* Evaluate a FraSCAti FScript statement.
*
* @param script A FraSCAti FScript statement.
* @return a serializable resource, else an FScript node (or nodes list).
*/
public Collection eval(String script) throws ScriptException {
Object evalResults = engine.eval(script);
Collection results = new ArrayList();
if (evalResults instanceof Collection) {
Collection> objects = (Collection>) evalResults;
for (Object obj : objects) {
Node resource = getRessoureFor(obj);
results.add(resource);
}
} else {
Node resource = getRessoureFor(evalResults);
results.add(resource);
}
return results;
}
/**
* Register a new script into the FraSCAti FScript engine.
*/
public String register(String script) throws ScriptException {
Reader reader = new StringReader( script.trim() );
@SuppressWarnings("unchecked")
Set loadedProc = (Set) engine.eval(reader);
return loadedProc.toString();
}
// Implementation of the RemoteScaDomain interface
/**
* See {@link Deployment#deploy(byte[])}
*/
public int deploy(String encodedContribution) {
File destFile = null;
BufferedOutputStream bos = null;
byte[] contribution = null;
try {
contribution = Base64Utility.decode(encodedContribution);
} catch (Base64Exception b64e) {
log.log(Level.SEVERE, "Cannot Base64 encode the file", b64e);
return -1;
}
try {
destFile = File.createTempFile("frascati-remote", "contribution");
} catch (IOException e) {
log.log(Level.SEVERE, "Cannot create a temporary file", e);
return -1;
}
try {
log.finer("Writing Contribution file");
bos = new BufferedOutputStream( new FileOutputStream(destFile) );
bos.write(contribution);
log.finer("Contribution file written");
} catch(IOException ioe) {
log.log(Level.SEVERE, "Error while writing file", ioe);
return -1;
} finally {
if(bos != null) {
try {
//flush the BufferedOutputStream
bos.flush();
bos.close();
} catch(Exception e){
log.log(Level.SEVERE, "Cannot flush the contribution file", e);
return -1;
}
}
}
try {
compositeManager.getContribution(destFile.getAbsolutePath());
} catch (ManagerException e) {
log.log(Level.SEVERE, "Cannot load the contribution", e);
return -1;
} finally {
destFile.delete();
}
return 0;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy