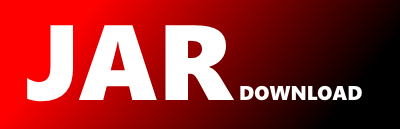
org.ow2.frascati.remote.introspection.ResourceUtil Maven / Gradle / Ivy
The newest version!
/***
* OW2 FraSCAti Introspection
* Copyright (C) 2008-2010 INRIA, University of Lille 1
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*
* Authors: Christophe Demarey
*/
package org.ow2.frascati.remote.introspection;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Hashtable;
import java.util.List;
import java.util.logging.Logger;
import org.objectweb.fractal.api.Interface;
import org.objectweb.fractal.api.NoSuchInterfaceException;
import org.objectweb.fractal.api.control.AttributeController;
import org.objectweb.fractal.api.control.BindingController;
import org.objectweb.fractal.api.control.ContentController;
import org.objectweb.fractal.api.control.LifeCycleController;
import org.objectweb.fractal.api.type.InterfaceType;
import org.objectweb.fractal.bf.AbstractSkeleton;
import org.objectweb.fractal.bf.connectors.jsonrpc.JsonRpcSkeletonContentAttributes;
import org.objectweb.fractal.bf.connectors.jsonrpc.JsonRpcStubContentAttributes;
import org.objectweb.fractal.bf.connectors.rest.RestSkeletonContentAttributes;
import org.objectweb.fractal.bf.connectors.rest.RestStubContentAttributes;
import org.objectweb.fractal.bf.connectors.ws.WsSkeletonContentAttributes;
import org.objectweb.fractal.bf.connectors.ws.WsStubContentAttributes;
import org.objectweb.fractal.util.AttributesHelper;
import org.objectweb.fractal.util.Fractal;
import org.ow2.frascati.remote.introspection.resources.Attribute;
import org.ow2.frascati.remote.introspection.resources.Binding;
import org.ow2.frascati.remote.introspection.resources.BindingKind;
import org.ow2.frascati.remote.introspection.resources.Component;
import org.ow2.frascati.remote.introspection.resources.ComponentStatus;
import org.ow2.frascati.remote.introspection.resources.Port;
import org.ow2.frascati.remote.introspection.resources.Property;
import org.ow2.frascati.tinfi.api.control.SCAPropertyController;
/**
* Utility class used to convert objects from Fractal API
* to Rest (SCA) resources.
*
* @author Christophe Demarey
*/
public class ResourceUtil {
/** The logger */
public static Logger log = Logger.getLogger("org.ow2.frascati.introspection.ResourceUtil");
// Private methods
/**
* Tells if this component owns SCA properties or not.
*
* @param pc The SCA Property Controller of this component.
* @return True if this component has at least one property.
*/
final private static boolean hasProperties(SCAPropertyController pc) {
return pc.getPropertyNames().length > 0;
}
// Public methods
/**
* Get a Component resource from a fractal Component.
*
* @param comp The fractal component to map.
* @return A Component Resource or null if comp cannot be introspect.
*/
public static Component getComponentResource(org.objectweb.fractal.api.Component comp) {
try {
String name = Fractal.getNameController(comp).getFcName();
String status = Fractal.getLifeCycleController(comp).getFcState();
Component compResource = new Component();
compResource.setName(name);
if ( status.compareTo(LifeCycleController.STARTED) == 0)
compResource.setStatus(ComponentStatus.STARTED);
else
compResource.setStatus(ComponentStatus.STOPPED);
return compResource;
} catch (NoSuchInterfaceException e) {
log.warning("Cannot find a name/status controller on " + comp);
}
return null;
}
/**
* Get full information on a fractal Component.
*
* @param comp A fractal component to introspect.
* @return A Component Resource or null if comp cannot be introspect.
*/
public static Component getFullComponentResource(org.objectweb.fractal.api.Component comp) {
Component compResource = null;
if (comp != null) {
try {
String name = Fractal.getNameController(comp).getFcName();
String status = Fractal.getLifeCycleController(comp).getFcState();
compResource = new Component();
compResource.setName(name);
if ( status.compareTo(LifeCycleController.STARTED) == 0)
compResource.setStatus(ComponentStatus.STARTED);
else
compResource.setStatus(ComponentStatus.STOPPED);
} catch (NoSuchInterfaceException e) {
log.warning("Cannot find a name/status controller on " + comp);
}
// Sub components => use RemoteScaDomainImpl.getSubComponents ?
try {
ContentController cc = Fractal.getContentController(comp);
for(org.objectweb.fractal.api.Component child : cc.getFcSubComponents()) {
compResource.getComponents().add( getComponentResource(child) );
}
} catch (NoSuchInterfaceException e) {
// No sub-components
}
// Get SCA Services // Get SCA References
getScaInterfaces(comp, compResource);
// Get SCA properties
addProperties(comp, compResource.getProperties());
}
return compResource;
}
public static void getScaInterfaces(org.objectweb.fractal.api.Component comp, Component compResource) {
Hashtable collections = new Hashtable();
List entries = new ArrayList();
// Prepare the collection interfaces.
/* TODO
ComponentType compType = (ComponentType) comp.getFcType();
for (InterfaceType itfType : compType.getFcInterfaceTypes() ) {
if(itfType.isFcCollectionItf()) {
ContextContainer cc ;
if (itfType.isFcClientItf() )
cc = new ClientCollectionInterfaceContainer(itfType, comp);
else
cc = new ServerCollectionInterfaceContainer(itfType, comp);
collections.put(itfType.getFcItfName(),cc);
}
} */
// Introspect component interfaces.
for (Object itfObject : comp.getFcInterfaces()) {
if (!comp.equals(itfObject)) {
Interface itf = (Interface) itfObject;
InterfaceType itfType = (InterfaceType) itf.getFcItfType();
/* TODO
if (FcExplorer.isCollection(itf)) {
InterfaceType iType = (InterfaceType)itf.getFcItfType();
String name = FcExplorer.getName(itf);
Object context;
if (FcExplorer.isClient(itf))
context = new ClientInterfaceWrapper(itf);
else
context = itf;
((ContextContainer)collections.get(iType.getFcItfName())).addEntry(name, context);
} else*/
if (itfType.isFcClientItf()) {
compResource.getReferences().add( getPortResource(itf) );
} else {
if ( !itf.getFcItfName().endsWith("controller") )
compResource.getServices().add( getPortResource(itf) );
}
}
}
// Add the collection interfaces
/* TODO Enumeration keys,elements;
keys = collections.keys();
elements = collections.elements();
for (int i = 0 ; i < collections.size() ; i++) {
entries.add(new DefaultEntry(keys.nextElement(),elements.nextElement()));
}*/
}
public static Port getPortResource(org.objectweb.fractal.api.Interface itf) {
Port port = new Port();
port.setName( itf.getFcItfName() );
// port.implement = new org.ow2.frascati.introspection.resources.Interface( itf.getFcItfType().getClass().toString() );
// SCA bindings
addBindingsResource(itf, port.getBindings());
// SCA intents
return port;
}
/**
* Get SCA bindings defined on a SCA service or reference.
*
* @param itf - The interface to search bindings on.
* @param bindings - The collection of bindings to populate.
*/
public static void addBindingsResource( org.objectweb.fractal.api.Interface itf, Collection bindings) {
org.objectweb.fractal.api.Interface boundInterface = null;
org.objectweb.fractal.api.Component owner = itf.getFcItfOwner();
Binding binding = new Binding();
try {
BindingController bc = Fractal.getBindingController(owner);
boundInterface = (org.objectweb.fractal.api.Interface) bc.lookupFc( itf.getFcItfName() );
} catch(Exception e) {
// Nothing to do.
}
if (boundInterface != null) {
org.objectweb.fractal.api.Component boundInterfaceOwner = boundInterface.getFcItfOwner();
try {
AttributeController ac = Fractal.getAttributeController( boundInterfaceOwner );
// WS binding
if ( (ac instanceof WsSkeletonContentAttributes)
|| (ac instanceof WsStubContentAttributes) ) {
binding.setKind(BindingKind.WS);
}
// RESTful binding
if ( (ac instanceof RestSkeletonContentAttributes)
|| (ac instanceof RestStubContentAttributes) ) {
binding.setKind(BindingKind.REST);
}
// JSON-RPC binding
if ( (ac instanceof JsonRpcSkeletonContentAttributes)
|| (ac instanceof JsonRpcStubContentAttributes) ) {
binding.setKind(BindingKind.JSON_RPC);
}
// RMI binding
/* TODO: the rmi connector in the BF should be refactored to provide the AC on the composite
if ( (ac instanceof RmiStubAttributes)
|| (ac instanceof RmiSkeletonAttributes) ) {
entries.add( new DefaultEntry(FcExplorer.getPrefixedName(boundInterface),
new RmiBindingWrapper(boundInterface)) );
} */
// For all bindings
addAttributesResource(boundInterfaceOwner, binding.getAttributes());
bindings.add(binding);
} catch (NoSuchInterfaceException e1) {
// Nothing to do
}
}
// TODO get bindings on services : see org.ow2.frascati.explorer.context.ServiceContext#getServiceBindingEntries()
try {
org.objectweb.fractal.api.Component[] parents = Fractal.getSuperController(owner).getFcSuperComponents();
for (org.objectweb.fractal.api.Component c : parents) {
ContentController cc = Fractal.getContentController(c);
org.objectweb.fractal.api.Component[] children = cc.getFcSubComponents();
for (org.objectweb.fractal.api.Component child : children) {
String childName = Fractal.getNameController(child).getFcName();
if ( childName.endsWith("-skeleton") ) {
// check that the skeleton is bound to owner
BindingController bc = Fractal.getBindingController(child);
try {
Interface servant = (Interface) bc.lookupFc(AbstractSkeleton.SERVANT_CLIENT_ITF_NAME);
if (servant == itf) {
Interface servantItf = (Interface) child.getFcInterface(AbstractSkeleton.SERVANT_CLIENT_ITF_NAME);
// Check the binding kind
if( childName.endsWith("-ws-skeleton") )
binding.setKind(BindingKind.WS);
else if ( childName.endsWith("-RESTful-skeleton") )
binding.setKind(BindingKind.REST);
else if ( childName.endsWith("-JSON-RPC-skeleton") )
binding.setKind(BindingKind.JSON_RPC);
if ( binding.getKind() != null ) {
addAttributesResource(child, binding.getAttributes());
bindings.add(binding);
}
}
} catch (NoSuchInterfaceException e) {
// RMI skeleton has no servant
// Nothing to do, entry is added in ScaInterfaceContext.getRmiBindingEntriesFromComponentController()
if ( childName.endsWith("-rmi-skeleton") ) {
// check that the rmi skeleton is bound to owner
Interface delegate = (Interface) bc.lookupFc(AbstractSkeleton.DELEGATE_CLIENT_ITF_NAME);
String delegateName = Fractal.getNameController(delegate.getFcItfOwner()).getFcName();
if ( delegateName.equals( Fractal.getNameController(owner).getFcName() )) {
// Interface delegateItf = (Interface) child.getFcInterface(AbstractSkeleton.DELEGATE_CLIENT_ITF_NAME);
binding.setKind(BindingKind.RMI);
bindings.add(binding);
}
}
}
}
}
}
} catch (NoSuchInterfaceException e) {
e.printStackTrace();
}
}
/**
* Get attributes defined on a Fractal Component. This method is used to retrieve SCA bindings
* attributes such as uri, port, etc.
*
* @param comp A Fractal binding component.
* @param attributes The collection of attributes to populate
*/
public static void addAttributesResource(org.objectweb.fractal.api.Component comp, Collection attributes) {
AttributesHelper attrHelper = new AttributesHelper(comp);
for (Object attrName : attrHelper.getAttributesNames()) {
Attribute att = new Attribute();
String name = (String) attrName;
att.setName(name);
att.setType( attrHelper.getAttributeType(name).toString() );
att.setValue( attrHelper.getAttribute(name).toString() );
attributes.add(att);
}
}
/**
* Get the list of properties defined on an SCA component
*
* @param comp The SCA component to introspect
* @param props The collection of properties to populate
*/
public static void addProperties(org.objectweb.fractal.api.Component comp, Collection props) {
try {
SCAPropertyController propCtl = (SCAPropertyController) comp.getFcInterface(SCAPropertyController.NAME);
for ( String propName : propCtl.getPropertyNames() ) {
Property prop = new Property();
prop.setName(propName);
prop.setValue( propCtl.getValue(propName).toString() );
prop.setType( propCtl.getType(propName).toString() );
props.add(prop);
}
} catch (NoSuchInterfaceException e) {
// No properties
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy