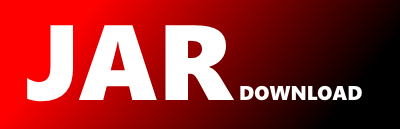
org.ow2.jasmine.jade.reflex.api.ReflexFractal Maven / Gradle / Ivy
/***
* Reflex-Fractal
*
* Copyright (C) 2007 : INRIA - Domaine de Voluceau, Rocquencourt, B.P. 105,
* 78153 Le Chesnay Cedex - France
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*
* Contact: jade inrialpes fr
*
* Author: SARDES project - http://sardes.inrialpes.fr
*
*/
package org.ow2.jasmine.jade.reflex.api;
import java.util.Map;
import org.objectweb.fractal.api.Component;
import org.objectweb.fractal.api.factory.Factory;
import org.objectweb.fractal.api.factory.GenericFactory;
import org.objectweb.fractal.api.factory.InstantiationException;
/**
* Provides a static method to get a Fractal bootstrap component which operates in
* reflexive mode (the components created by this bootstrap are reflexive components,
* which means that they are associated to a dual component created by a dual bootstrap).
*
* The fractal.provider system property should be defined, as it specifies
* which Fractal implementation to use.
*
* The reflex-fractal.name and reflex-fractal.level system properties
* respectively indicate the name and the reflexive level (execution / meta) of the
* returned Fractal bootstrap component.
*
* @author Fabienne Boyer
*/
public class ReflexFractal {
static Component bootstrapComponent = null;
/**
* Private constructor (uninstantiable class).
*/
private ReflexFractal() {
}
/**
* Returns a reflexive bootstrap component (i.e. a component which allows to
* create other components in reflexive mode). This method more precisely
* creates an instance of the class whose name is prefixed by
* "org.ow2.jasmine.jade.reflex.util.Reflex" followed by the fractal.provider
* system property. For instance, if the fractal.provider system
* property is equal to org.objectweb.fractal.julia.Julia, this method
* instanciate the class org.ow2.jasmine.jade.reflex.util.ReflexJulia. This class must
* implement the {@link Factory} interface.
*
* @return a bootstrap component to create other components. This component
* provides at least two interfaces named type-factory
* and generic-factory to create component types and
* components. The type of the generic-factory interface
* is {@link org.objectweb.fractal.api.factory.GenericFactory}, or
* a sub type of this type, but the type of the
* type-factory interface is not necessarily
* {@link org.objectweb.fractal.api.type.TypeFactory} (or a sub type
* of this type).
* @throws InstantiationException
* if the bootstrap component cannot be created.
*/
public static Component getBootstrapComponent()
throws InstantiationException {
if (bootstrapComponent != null)
return bootstrapComponent;
String bootTmplClassName = System.getProperty("fractal.provider");
if (bootTmplClassName == null) {
throw new InstantiationException(
"The fractal.provider system property is not defined");
}
Factory bootTmpl;
try {
/*
* TODO build the bootTmpClassName from configuration file Class
* bootTmplClass = Class.forName("Reflex" + bootTmplClassName);
*/
Class bootTmplClass = Class
.forName("org.ow2.jasmine.jade.reflex.util.ReflexJulia");
bootTmpl = (Factory) bootTmplClass.newInstance();
} catch (Exception e) {
throw new InstantiationException(
"Cannot find or instantiate the 'Reflex"
+ bootTmplClassName
+ "' class specified in the fractal.provider system property");
}
bootstrapComponent = bootTmpl.newFcInstance();
return bootstrapComponent;
}
/**
* Returns a bootstrap component to create other components. This method
* creates an instance of the class whose name is associated to the
* "fractal.provider" key, which much implement the {@link Factory} or
* {@link GenericFactory} interface, and returns the component instantiated
* by this factory.
*
* @param hints
* a map which must associate a value to the "fractal.provider"
* key, and which may associate a ClassLoader to the
* "classloader" key. This class loader will be used to load the
* bootstrap component.
* @return a bootstrap component to create other components.
* @throws InstantiationException
* if the bootstrap component cannot be created.
*/
public static Component getBootstrapComponent(final Map hints)
throws InstantiationException {
String bootTmplClassName = (String) hints.get("fractal.provider");
if (bootTmplClassName == null) {
bootTmplClassName = System.getProperty("fractal.provider");
}
// TODO build the bootTmpClassName from configuration file
System.out
.println("ReflexFractal.getBootstrapComponent(hints) NOT YET IMPLEMENTED");
if (bootTmplClassName == null) {
throw new InstantiationException(
"The fractal.provider value is not defined");
}
Object bootTmpl;
try {
ClassLoader cl = (ClassLoader) hints.get("classloader");
if (cl == null) {
cl = new ReflexFractal().getClass().getClassLoader();
}
Class bootTmplClass = cl.loadClass(bootTmplClassName);
bootTmpl = bootTmplClass.newInstance();
} catch (Exception e) {
throw new InstantiationException("Cannot find or instantiate the '"
+ bootTmplClassName
+ "' class associated to the fractal.provider key");
}
if (bootTmpl instanceof GenericFactory) {
return ((GenericFactory) bootTmpl).newFcInstance(null, null, hints);
} else {
return ((Factory) bootTmpl).newFcInstance();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy