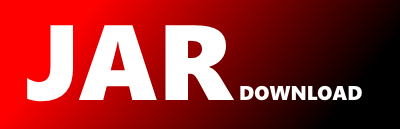
org.ow2.jasmine.jade.reflex.util.Reflex Maven / Gradle / Ivy
/***
* Reflex-Fractal
*
* Copyright (C) 2007 : INRIA - Domaine de Voluceau, Rocquencourt, B.P. 105,
* 78153 Le Chesnay Cedex - France
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*
* Contact: jade inrialpes fr
*
* Author: SARDES project - http://sardes.inrialpes.fr
*
*/
package org.ow2.jasmine.jade.reflex.util;
import org.objectweb.fractal.api.Component;
import org.objectweb.fractal.api.Interface;
import org.objectweb.fractal.api.NoSuchInterfaceException;
import org.objectweb.fractal.api.control.ContentController;
import org.ow2.jasmine.jade.reflex.api.control.BindingNotificationController;
import org.ow2.jasmine.jade.reflex.api.control.ContentNotificationController;
import org.ow2.jasmine.jade.reflex.api.control.GenericAttributeNotificationController;
import org.ow2.jasmine.jade.reflex.api.control.LifeCycleNotificationController;
import org.ow2.jasmine.jade.reflex.api.control.NameNotificationController;
import org.ow2.jasmine.jade.reflex.api.control.ReflexController;
import org.ow2.jasmine.jade.reflex.api.control.SpecificAttributeController;
import org.ow2.jasmine.jade.reflex.api.factory.GenericFactoryNotification;
import org.ow2.jasmine.jade.reflex.api.factory.GenericInstallingFactoryNotification;
/**
* Provides utility methods to deal with reflexive components.
*
* @author Fabienne Boyer
*
*/
public class Reflex {
/**
* prefix for controllers names in Julia configuration file
*/
public static String controllersPrefix = "reflex-";
/**
* Returns the {@link ReflexController} interface of the given component.
*
* @param component
* a component.
* @return the {@link ReflexController} interface of the given component.
* @throws NoSuchInterfaceException
* if there is no such interface.
*/
public static ReflexController getReflexController(final Component component)
throws NoSuchInterfaceException {
return (ReflexController) component.getFcInterface("reflex-controller");
}
/**
* Returns the {@link BindingNotificationController} interface of the given
* component.
*
* @param component
* a component.
* @return the {@link BindingNotificationController} interface of the given
* component.
* @throws NoSuchInterfaceException
* if there is no such interface.
*/
public static BindingNotificationController getBindingNotificationController(
final Component component) throws NoSuchInterfaceException {
return (BindingNotificationController) component
.getFcInterface("binding-notification-controller");
}
/**
* Returns the {@link ContentNotificationController} interface of the given
* component.
*
* @param component
* a component.
* @return the {@link ContentNotificationController} interface of the given
* component.
* @throws NoSuchInterfaceException
* if there is no such interface.
*/
public static ContentNotificationController getContentNotificationController(
final Component component) throws NoSuchInterfaceException {
return (ContentNotificationController) component
.getFcInterface("content-notification-controller");
}
/**
* Returns the {@link LifeCycleNotificationController} interface of the
* given component.
*
* @param component
* a component.
* @return the {@link LifeCycleNotificationController} interface of the
* given component.
* @throws NoSuchInterfaceException
* if there is no such interface.
*/
public static LifeCycleNotificationController getLifeCycleNotificationController(
final Component component) throws NoSuchInterfaceException {
return (LifeCycleNotificationController) component
.getFcInterface("lifecycle-notification-controller");
}
/**
* Returns the {@link NameNotificationController} interface of the given
* component.
*
* @param component
* a component.
* @return the {@link NameNotificationController} interface of the given
* component.
* @throws NoSuchInterfaceException
* if there is no such interface.
*/
public static NameNotificationController getNameNotificationController(
final Component component) throws NoSuchInterfaceException {
return (NameNotificationController) component
.getFcInterface("name-notification-controller");
}
/**
* Returns the {@link GenericAttributeNotificationController} interface of
* the given component.
*
* @param component
* a component.
* @return the {@link GenericAttributeNotificationController} interface
* of the given component.
* @throws NoSuchInterfaceException
* if there is no such interface.
*/
public static GenericAttributeNotificationController getGenericAttributeNotificationController(
final Component component) throws NoSuchInterfaceException {
return (GenericAttributeNotificationController) component
.getFcInterface("generic-attribute-notification-controller");
}
/**
* Returns the {@link SpecificAttributeController} interface of the given
* component.
*
* @param component
* a component.
* @return the {@link SpecificAttributeController} interface of the given
* component.
* @throws NoSuchInterfaceException
* if there is no such interface.
*/
public static SpecificAttributeController getSpecificAttributeController(
final Component component) throws NoSuchInterfaceException {
return (SpecificAttributeController) component
.getFcInterface("specific-attribute-controller");
}
/**
* Returns the {@link GenericFactoryNotification} interface of the given
* component.
*
* @param component
* a component.
* @return the {@link GenericFactoryNotification} interface of the given
* component.
* @throws NoSuchInterfaceException
* if there is no such interface.
*/
public static GenericFactoryNotification getGenericFactoryNotification(
final Component component) throws NoSuchInterfaceException {
return (GenericFactoryNotification) component
.getFcInterface("generic-factory-notification");
}
/**
* Returns the {@link GenericFactoryNotification} interface of the given
* component.
*
* @param component
* a component.
* @return the {@link GenericFactoryNotification} interface of the given
* component.
* @throws NoSuchInterfaceException
* if there is no such interface.
*/
public static GenericInstallingFactoryNotification getGenericInstallingFactoryNotification(
final Component component) throws NoSuchInterfaceException {
return (GenericInstallingFactoryNotification) component
.getFcInterface("generic-installing-factory-notification");
}
/**
* Returns the {@link Component} interface of the given component.
*
* @param component
* a component.
* @return the {@link Component} interface of the given component.
* @throws NoSuchInterfaceException
* if there is no such interface.
*/
public static Component getComponent(final Component component)
throws NoSuchInterfaceException {
return (Component) component.getFcInterface("component");
}
/**
* Get the dual componant of a given component
*
* @param o
* the component reference
* @return the dual componant reference null if the conversion is impossible
*/
public static Component getDualComponent(Component c)
throws NoSuchInterfaceException {
return ((ReflexController) c.getFcInterface("reflex-controller"))
.getCmpRef();
}
/**
* Get the dual controller of a given controller
*
* @param comp
* the controller's component
* @param name
* the controller's interface name
* @return the dual controller reference null if the conversion is
* impossible
*/
public static Interface getDualController(Component comp, String name)
throws NoSuchInterfaceException {
Component dualcomp = getDualComponent(comp);
return (Interface) (dualcomp.getFcInterface(name));
}
/**
* Get the dual interface of a given interface
*
* @param i
* the interface reference
* @return the dual interface reference null if the conversion is impossible
*/
public static Interface getDualInterface(Interface itf)
throws NoSuchInterfaceException {
String itfname = ((Interface) itf).getFcItfName();
Component dualcomp = getDualComponent(((Interface) itf).getFcItfOwner());
if (((Interface) itf).isFcInternalItf()) {
ContentController dualcc = (ContentController) (dualcomp
.getFcInterface("content-controller"));
return (Interface) (dualcc.getFcInternalInterface(itfname));
} else {
return (Interface) (dualcomp.getFcInterface(itfname));
}
}
/**
* Convert the reference
*
* @param o
* the ref to be converted
* @return the ref converted if necessary null if the conversion is
* impossible
*/
public static Object getDual(Object o) throws NoSuchInterfaceException {
if (o instanceof Interface) {
return getDualInterface((Interface) o);
} else if (o instanceof Component) {
return getDualComponent((Component) o);
} else
// nothing2do
Logger.println("Reflex.getDual : error");
return o;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy