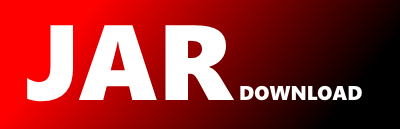
org.ow2.jasmine.jade.reflex.util.ReflexJulia Maven / Gradle / Ivy
/***
* Reflex-Fractal
*
* Copyright (C) 2007 : INRIA - Domaine de Voluceau, Rocquencourt, B.P. 105,
* 78153 Le Chesnay Cedex - France
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*
* Contact: jade inrialpes fr
*
* Author: SARDES project - http://sardes.inrialpes.fr
*
*/
package org.ow2.jasmine.jade.reflex.util;
import java.util.HashMap;
import java.util.Map;
import org.objectweb.fractal.api.Component;
import org.objectweb.fractal.api.Type;
import org.objectweb.fractal.api.factory.Factory;
import org.objectweb.fractal.api.factory.GenericFactory;
import org.objectweb.fractal.api.factory.InstantiationException;
import org.objectweb.fractal.api.type.ComponentType;
import org.objectweb.fractal.api.type.InterfaceType;
import org.objectweb.fractal.julia.factory.BasicGenericFactoryMixin;
import org.objectweb.fractal.julia.factory.ChainedInstantiationException;
import org.objectweb.fractal.julia.loader.Loader;
import org.objectweb.fractal.julia.type.BasicTypeFactoryMixin;
import org.ow2.jasmine.jade.fractal.julia.factory.JadeGenericFactoryMixin;
/**
* Provides access to a Julia bootstrap component which operates in reflexive mode.
* (the components created by this bootstrap are reflexive components, which means
* that they are associated to a dual component created by a dual bootstrap).
*
* The name and the level (execution / meta) of the bootstrap are given
* by the reflex-fractal.name and reflex-fractal.level system properties.
*
* At instanciation time, the bootstrap registrates itself under the naming service and
* automatically get the reference of its dual bootstrap if these has already been started.
*
* @author Fabienne Boyer
*
*/
public class ReflexJulia implements Factory, GenericFactory {
/**
* The bootstrap component.
*/
private static Component bootstrapComponent;
/**
* The bootstrap description name defined in Julia configuration file
*/
private static final String bootstrapName = "reflex-bootstrap-with-name";
// -------------------------------------------------------------------------
// Implementation of the Factory interface
// -------------------------------------------------------------------------
/**
* @return null.
*/
public Type getFcInstanceType() {
return null;
}
/**
* @return null.
*/
public Object getFcControllerDesc() {
return null;
}
/**
* @return null.
*/
public Object getFcContentDesc() {
return null;
}
// -------------------------------------------------------------------------
// Implementation of GenericFactory interface
// -------------------------------------------------------------------------
/*
* (non-Javadoc)
*
* @see org.objectweb.fractal.api.factory.Factory#newFcInstance()
*/
public Component newFcInstance() throws InstantiationException {
return newFcInstance(new HashMap());
}
public Component newFcInstance(Type type, Object contentDesc,
Object controllerDesc) throws InstantiationException {
Map context;
if (contentDesc instanceof Map) {
context = (Map) contentDesc;
} else {
context = new HashMap();
}
return newFcInstance(context);
}
// -------------------------------------------------------------------------
// Implementation of Factory interface
// -------------------------------------------------------------------------
/**
* Returns a reflexive Julia bootstrap component. If this component does not
* exists yet, it is created as follows:
*
* - a pre bootstrap component is created by assembling a {@link Loader}
* object, a {@link BasicTypeFactoryMixin} object, and a {@link
* BasicGenericFactoryMixin} object. The loader object is created by
* instantiating the class specified in the "julia.loader" system property.
* - the pre bootstrap component is used to create the real bootstrap
* component, by calling the newFcInstance method of the
* GenericFactory interface of the pre bootstrap component, with
* the "reflex-bootstrap" string as controller descriptor.
*
*
* @return the {@link Component} interface of the component instantiated
* from this factory.
* @throws InstantiationException
* if the component cannot be created.
*/
private Component newFcInstance(final Map context)
throws InstantiationException {
String boot = null;
if (bootstrapComponent == null) {
boot = System.getProperty("julia.loader");
if (boot == null) {
throw new InstantiationException(
"The julia.loader [system] property is not defined");
}
// creates the pre bootstrap controller components
Loader loader;
try {
loader = (Loader) _forName(boot).newInstance();
loader.init(new HashMap());
} catch (Exception e) {
throw new InstantiationException(
"Cannot find or instantiate the '"
+ boot
+ "' class specified in the julia.loader [system] property");
}
BasicTypeFactoryMixin typeFactory = new BasicTypeFactoryMixin();
JadeGenericFactoryMixin genericFactory = new JadeGenericFactoryMixin();
genericFactory._this_weaveableL = loader;
genericFactory._this_weaveableTF = typeFactory;
// use the pre bootstrap component to create the real bootstrap
// component
ComponentType t = typeFactory.createFcType(new InterfaceType[0]);
try {
bootstrapComponent = genericFactory.newFcInstance(t,bootstrapName, null);
((Loader) bootstrapComponent.getFcInterface("loader"))
.init(new HashMap());
} catch (Exception e) {
Logger.println(DebugReflex.on,
"[ReflexJulia] error when creating " + bootstrapName
+ " : " + e);
if (DebugReflex.on)
e.printStackTrace();
throw new ChainedInstantiationException(e, null,
"Cannot create the bootstrap component");
}
}
return bootstrapComponent;
}
// -------------------------------------------------------------------------
// Private methods
// -------------------------------------------------------------------------
/**
* Convenient method used for J2ME conversion (ClassLoader not available in
* CDLC)
*
* @param name
* @return
* @throws ClassNotFoundException
*/
private Class _forName(final String name) throws ClassNotFoundException {
return getClass().getClassLoader().loadClass(name);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy