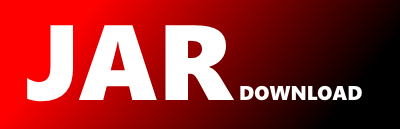
org.ow2.jonas.autostart.utility.Utility Maven / Gradle / Ivy
/**
* JOnAS: Java(TM) Open Application Server
* Copyright (C) 2010 Bull S.A.S.
* Contact: [email protected]
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307
* USA
*
* --------------------------------------------------------------------------
* $Id: Utility.java 20844 2011-02-07 14:14:54Z benoitf $
* --------------------------------------------------------------------------
*/
package org.ow2.jonas.autostart.utility;
import java.awt.Image;
import java.awt.Toolkit;
import java.io.BufferedReader;
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.FileWriter;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.PrintWriter;
import java.net.HttpURLConnection;
import java.net.InetSocketAddress;
import java.net.MalformedURLException;
import java.net.Proxy;
import java.net.SocketAddress;
import java.net.URL;
import java.net.URLClassLoader;
import java.util.logging.Level;
import java.util.logging.Logger;
import org.apache.tools.ant.taskdefs.Replace;
import org.ow2.jonas.autostart.proxy.AutoStartProxy;
/**
* This class contains specifics functions used by JOnAS Builder and Starter
* librairies.
* @author skabore
*/
public final class Utility {
/**
* The buffer size constant.
*/
private static final int BUFFER_SIZE = 1024;
/**
* The four constant.
*/
private static final int FOUR = 4;
/**
* The file separator.
*/
private static final String FILE_SEPARATOR = System.getProperty("file.separator");
/**
* The proxy setting file.
*/
private static final File PROXY_SETTING_FILE = new File(getDefaultLocation(), "builder-proxy-settings.properties");
/**
* The proxy.
*/
private static AutoStartProxy autoStartProxy = new AutoStartProxy();
private final static char base64Array[] = {'A', 'B', 'C', 'D', 'E', 'F', 'G', 'H', 'I', 'J', 'K', 'L', 'M', 'N', 'O', 'P',
'Q', 'R', 'S', 'T', 'U', 'V', 'W', 'X', 'Y', 'Z', 'a', 'b', 'c', 'd', 'e', 'f', 'g', 'h', 'i', 'j', 'k', 'l', 'm',
'n', 'o', 'p', 'q', 'r', 's', 't', 'u', 'v', 'w', 'x', 'y', 'z', '0', '1', '2', '3', '4', '5', '6', '7', '8', '9',
'+', '/'};
/**
* Build a new Utility.
*/
private Utility() {
}
/**
* Return the user platform (Windows or others platforms.
* @return the user platform
*/
public static String getPlatform() {
if (System.getProperty("os.name").toLowerCase().contains("windows")) {
return "windows";
}
return "others";
}
/**
* @return the default folder where JOnAS ROOT will be installed.
*/
public static String getDefaultLocation() {
if (getPlatform().equals("windows")) {
return "C:\\Temp";
}
return "/tmp";
}
/**
* Creates a directory.
* @param directory the directory to create
*/
public static void createDirectory(final File directory) {
if (directory.exists()) {
directory.delete();
}
directory.mkdir();
}
/**
* Creates a new file.
* @param file the file to create
*/
public static void createNewFile(final File file) {
try {
if (file.exists()) {
file.delete();
}
file.createNewFile();
} catch (IOException ex) {
Logger.getLogger(Utility.class.getName()).log(Level.SEVERE, null, ex);
}
}
/**
* Deletes a directory.
* @param path the path of file to delete
* @return true/false
*/
public static boolean deleteDirectory(final File path) {
if (path.isFile() && path.exists()) {
return path.delete();
}
boolean resultat = true;
if (path.exists()) {
if (path.isDirectory()) {
File[] files = path.listFiles();
for (int i = 0; i < files.length; i++) {
if (files[i].isDirectory()) {
resultat &= deleteDirectory(files[i]);
} else {
resultat &= files[i].delete();
}
}
}
}
resultat &= path.delete();
return resultat;
}
/**
* Checks if a folder contains a file.
* @param folder the folder to check
* @param fileName the file to check.
* @return true if folder contains fileName, false otherwise.
*/
public static boolean containFile(final File folder, final String fileName) {
if (folder.exists() && folder.isDirectory()) {
File[] files = folder.listFiles();
for (int i = 0; i < files.length; i++) {
if (files[i].getName().equals(fileName)) {
return true;
}
}
}
return false;
}
/**
* Checks if the specified folder is a valid JOnAS Root folder.
* @param folder the folder to check
* @return true if folder is a valid JOnAS folder, false otherwise.
*/
public static boolean isValidJOnASRootFolder(final File folder) {
return isValidFolder(folder, true);
}
/**
* Checks if the specified folder is a valid JOnAS Root folder.
* @param folder the folder to check
* @return true if folder is a valid JOnAS folder, false otherwise.
*/
public static boolean isValidJOnASBaseFolder(final File folder) {
return isValidFolder(folder, false);
}
/**
* Checks if the specified folder is a valid JOnAS folder.
* @param folder the folder to check
* @param root true if it is the reoot folder, false if base folder.
* @return true if folder is a valid JOnAS folder, false otherwise.
*/
public static boolean isValidFolder(final File folder, final boolean root) {
if (!(Utility.containFile(folder, "conf") && Utility.containFile(folder, "deploy") && Utility
.containFile(folder, "lib"))) {
return false;
}
if (root) {
if (!(Utility.containFile(folder, "bin"))) {
return false;
}
}
if (root) {
File bin = new File(folder.getAbsolutePath() + FILE_SEPARATOR + "bin");
if (!(Utility.containFile(bin, "jonas") && Utility.containFile(bin, "jonas.bat")
&& Utility.containFile(bin, "setenv") && Utility.containFile(bin, "setenv.bat"))) {
return false;
}
}
File conf = new File(folder.getAbsolutePath() + FILE_SEPARATOR + "conf");
if (!(Utility.containFile(conf, "jonas.properties") && Utility.containFile(conf, "carol.properties")
&& Utility.containFile(conf, "trace.properties") && Utility.containFile(conf, "jotm.properties")
&& Utility.containFile(conf, "jonas-realm.xml") && Utility.containFile(conf, "easybeans-jonas.xml") && Utility
.containFile(conf, "classloader-default-filtering.xml"))) {
return false;
}
if (root) {
File repositories = new File(folder.getAbsolutePath() + FILE_SEPARATOR + "repositories");
if (!(Utility.containFile(repositories, "maven2-internal"))) {
return false;
}
if (!(Utility.containFile(repositories, "url-internal"))) {
return false;
}
}
if (!root) {
if ((Utility.containFile(folder, "bin"))) {
return false;
}
}
/*
* ...
*/
// return Utility.containFile(folder, "repositories");
return true;
}
/**
* Splits string around matches of the given regular expression.
* @param args the string to split
* @param regex the delimiting regular expression
* @return the array of strings computed by splitting this string.
*/
public static String[] parseStrToArray(final String args, final String regex) {
return args.split(regex);
}
/**
* Checks if application location is valid and it's an war or ear
* application.
* @param application the application path
* @return true if application location is valid and it's an war ear, ejb or
* xml extension, false otherwise.
*/
public static boolean checkApplication(final String application) {
if ((new File(application)).isFile() && (new File(application)).exists()) {
return !(application.lastIndexOf(".war") == -1) || !(application.lastIndexOf(".ear") == -1)
|| !(application.lastIndexOf(".jar") == -1) || !(application.lastIndexOf(".xml") == -1)
|| !(application.lastIndexOf(".rar") == -1);
}
return false;
}
/**
* Open the connexion.
* @param url the url to check connection
* @return connection
*/
public static HttpURLConnection openConnexion(final URL url) {
HttpURLConnection connection = null;
String proxyAdress = autoStartProxy.address;
String proxyPort = autoStartProxy.port;
Proxy proxy = null;
if (!"".equals(proxyAdress) && proxyAdress != null && !"".equals(proxyPort) && proxyPort != null) {
SocketAddress addr = new InetSocketAddress(proxyAdress, Integer.parseInt(proxyPort));
proxy = new Proxy(Proxy.Type.HTTP, addr);
}
try {
if (proxy != null) {
connection = (HttpURLConnection) url.openConnection(proxy);
String proxyUser = autoStartProxy.user;
String proxyPassword = autoStartProxy.password;
if (proxyUser != null && !"".equals(proxyUser) && !"".equals(proxyPassword) && proxyPassword != null) {
String dataProxy = proxyUser + ":" + proxyPassword;
String encodedPassword = base64Encode(dataProxy);
connection.setRequestProperty("Proxy-Authorization", encodedPassword);
}
} else {
connection = (HttpURLConnection) url.openConnection();
}
connection.setConnectTimeout(10000);
connection.setReadTimeout(10000);
connection.connect();
} catch (MalformedURLException ex) {
Logger.getLogger(Utility.class.getName()).log(Level.OFF, ex.getMessage());
} catch (IOException ex) {
Logger.getLogger(Utility.class.getName()).log(Level.OFF, ex.getMessage());
}
return connection;
}
/**
* Checks if the connection to url is established.
* @param url the url to check connection
* @return true/false
*/
public static boolean testConnexionURL(final URL url) {
HttpURLConnection connection = openConnexion(url);
if (connection != null) {
try {
return connection.getResponseCode() == HttpURLConnection.HTTP_OK;
} catch (IOException ex) {
Logger.getLogger(Utility.class.getName()).log(Level.SEVERE, null, ex);
}
}
return false;
}
/**
* Initialize the proxy parameters.
* @param proxyaddress the proxy address
* @param proxyport the proxy port
* @param proxyuser the proxy user
* @param proxypwd the proxy user password
*/
public static void initProxyValues(final AutoStartProxy proxy) {
Utility.autoStartProxy = proxy;
}
/**
* Sets the proxy parameters.
*/
public static void setProxyValues(final AutoStartProxy proxy) {
if (PROXY_SETTING_FILE.isFile()) {
String[] dataLine = new String[FOUR];
int i = 0;
try {
InputStream ips = new FileInputStream(PROXY_SETTING_FILE);
InputStreamReader ipsr = new InputStreamReader(ips);
BufferedReader br = new BufferedReader(ipsr);
String line;
while ((line = br.readLine()) != null) {
dataLine[i] = line;
i++;
}
br.close();
} catch (Exception e) {
}
String proxyaddress = "";
String proxyport = "";
String proxyuser = "";
String proxypwd = "";
if (dataLine[0].split("=").length > 1) {
proxyaddress = dataLine[0].split("=")[1];
}
if (dataLine[1].split("=").length > 1) {
proxyport = dataLine[1].split("=")[1];
}
if (dataLine[2].split("=").length > 1) {
proxyuser = dataLine[2].split("=")[1];
}
if (dataLine[3].split("=").length > 1) {
proxypwd = dataLine[3].split("=")[1];
}
AutoStartProxy pxy = new AutoStartProxy();
pxy.address = proxyaddress;
pxy.port = proxyport;
pxy.user = proxyuser;
pxy.password = proxypwd;
initProxyValues(pxy);
} else {
initProxyValues(proxy);
persitProxyValues(proxy);
}
}
/**
* Persit the proxy settings in the 'builder-proxy-settings.properties'
* file.
*/
public static void persitProxyValues(final AutoStartProxy proxy) {
String data = "proxyaddress=" + proxy.address;
data += "\n" + "proxyport=" + proxy.port;
data += "\n" + "proxyuser=" + proxy.user;
data += "\n" + "proxypwd=" + proxy.password;
editFile(PROXY_SETTING_FILE, data);
}
/**
* Replace a token in the file.
* @param fileName the file name to edit
* @param token the token to replace
* @param value the new token value
*/
public static void replace(final String fileName, final String token, final String value) {
Replace replace = new Replace();
replace.setFile(new File(fileName));
replace.setToken(token);
replace.setValue(value);
replace.execute();
}
/**
* Edit a file.
* @param fileName the file name to edit
* @param str the data to write in the file
*/
public static void editFile(final File fileName, final String str) {
FileWriter filewriter = null;
try {
filewriter = new FileWriter(fileName);
} catch (IOException ex) {
Logger.getLogger(Utility.class.getName()).log(Level.OFF, ex.getMessage());
}
BufferedWriter buffer = new BufferedWriter(filewriter);
PrintWriter pw = new PrintWriter(buffer);
pw.println(str);
pw.close();
}
/**
* Search and replace a character.
* @param fileName the file name to edit
* @param strToFind the data to find
* @param replaceStr the new data to write in the data
* @param erase if true strToFind is replace by replaceStr, else strToFind
* is Concatenated with replaceStr.
*/
public static void searchAndReplace(final File fileName, final String strToFind, final String replaceStr,
final boolean erase) {
String line = null;
String contentFile = "";
BufferedReader buffer = null;
try {
buffer = new BufferedReader(new FileReader(fileName));
if (buffer != null) {
try {
while ((line = buffer.readLine()) != null) {
if (line != null) {
if (line.contains(strToFind)) {
if (erase) {
line = replaceStr;
} else {
line = line.concat(replaceStr);
}
}
contentFile += line + "\n";
}
}
} catch (IOException ex) {
Logger.getLogger(Utility.class.getName()).log(Level.OFF, ex.getMessage());
} finally {
try {
buffer.close();
} catch (IOException ex) {
Logger.getLogger(Utility.class.getName()).log(Level.OFF, ex.getMessage());
}
}
}
} catch (FileNotFoundException ex) {
Logger.getLogger(Utility.class.getName()).log(Level.OFF, ex.getMessage());
}
editFile(fileName, contentFile);
}
/**
* Search a string in the file.
* @param fileName the file name to edit
* @param strToFind the string to find
* @return the string if it exists in the file, null otherwise
*/
public static String searchStr(final File fileName, final String strToFind) {
String line = null;
BufferedReader buffer = null;
try {
buffer = new BufferedReader(new FileReader(fileName));
if (buffer != null) {
try {
while ((line = buffer.readLine()) != null) {
if (line != null) {
if (line.contains(strToFind)) {
return line;
}
}
}
} catch (IOException ex) {
Logger.getLogger(Utility.class.getName()).log(Level.OFF, ex.getMessage());
} finally {
try {
buffer.close();
} catch (IOException ex) {
Logger.getLogger(Utility.class.getName()).log(Level.OFF, ex.getMessage());
}
}
}
} catch (FileNotFoundException ex) {
Logger.getLogger(Utility.class.getName()).log(Level.OFF, ex.getMessage());
}
return null;
}
/**
* Return the name of web container file properties.
* @param jonasConfDir the JOnAS configuration directory
* @param server the web container (tomcat or jetty
* @return the name of web container file properties
*/
public static String getWebContainerFile(final File jonasConfDir, final String server) {
File[] files = jonasConfDir.listFiles();
for (File file : files) {
String name = file.getName();
if (server.equalsIgnoreCase("tomcat")) {
if (name.endsWith("-server.xml")) {
return file.getName();
}
} else {
if (server.equalsIgnoreCase("jetty")) {
if (name.startsWith("jetty") && !name.contains("-web")) {
return file.getName();
}
}
}
}
return "";
}
/**
* The resource URL loader.
*/
private static URLClassLoader urlLoader = (URLClassLoader) ClassLoader.getSystemClassLoader();
/**
* Extract file from Jar.
* @param file the path of the file
* @return URL to access to the resource
*/
public static URL extractFile(final String file) {
return urlLoader.findResource(file);
}
/**
* Return an image.
* @param file the image name
* @return the loaded image
*/
public static Image getImage(final String file) {
return Toolkit.getDefaultToolkit().getImage(extractFile(file));
}
/**
* @return proxy value.
*/
public static AutoStartProxy getAutoStartProxy() {
return autoStartProxy;
}
/**
* Set proxy value.
* @param proxy the proxy value.
*/
public static void setAutoStartProxy(final AutoStartProxy proxy) {
Utility.autoStartProxy = proxy;
}
/**
* @return true if JOnAS Root folder is a full JOnAS server, false if a
* micro server.
* @param jonasRoot the JOnAS Root folder.
*/
public static boolean isFullJonasFolder(final File jonasRoot) {
return new File(jonasRoot, "templates" + FILE_SEPARATOR + "conf").exists();
}
/**
* Check if the number is an integer.
* @param number the number to check.
* @return the checked number.
*/
public static String checkIntegerNumberFormat(String number) {
if (number.length() > 0) {
try {
Integer.parseInt(number);
} catch (NumberFormatException nfe) {
number = "";
}
}
return number;
}
public static String base64Encode(final String string) {
String encodedString = "";
byte bytes[] = string.getBytes();
int i = 0;
int pad = 0;
while (i < bytes.length) {
byte b1 = bytes[i++];
byte b2;
byte b3;
if (i >= bytes.length) {
b2 = 0;
b3 = 0;
pad = 2;
} else {
b2 = bytes[i++];
if (i >= bytes.length) {
b3 = 0;
pad = 1;
} else {
b3 = bytes[i++];
}
}
byte c1 = (byte) (b1 >> 2);
byte c2 = (byte) (((b1 & 0x3) << 4) | (b2 >> 4));
byte c3 = (byte) (((b2 & 0xf) << 2) | (b3 >> 6));
byte c4 = (byte) (b3 & 0x3f);
encodedString += base64Array[c1];
encodedString += base64Array[c2];
switch (pad) {
case 0:
encodedString += base64Array[c3];
encodedString += base64Array[c4];
break;
case 1:
encodedString += base64Array[c3];
encodedString += "=";
break;
case 2:
encodedString += "==";
break;
}
}
return encodedString;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy