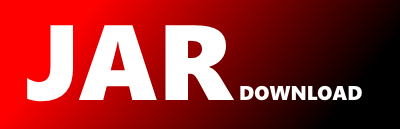
org.ow2.jonas.eclipse.compiler.CompilerError Maven / Gradle / Ivy
The newest version!
/**
* JOnAS: Java(TM) Open Application Server
* Copyright (C) 2007 Bull S.A.S.
* Contact: [email protected]
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA
*
* --------------------------------------------------------------------------
* $Id: CompilerError.java 15428 2008-10-07 11:20:29Z sauthieg $
* --------------------------------------------------------------------------
*/
package org.ow2.jonas.eclipse.compiler;
import org.eclipse.jdt.core.compiler.IProblem;
/**
* Error that happened during the compilation process.
* @author Guillaume Sauthier
*/
public class CompilerError {
/**
* Error message.
*/
private String message;
/**
* Error cause (if the cause was an execution Exception).
*/
private Exception cause;
/**
* The error problem (if the cause is a compilation error).
*/
private IProblem problem;
/**
* Creates a new {@link CompilerError} coming from the execution.
* @param message Descriptive error message.
* @param exception Exception's cause.
*/
public CompilerError(final String message, final Exception exception) {
this.message = message;
this.cause = exception;
}
/**
* Creates a new {@link CompilerError} coming from the compilation itself.
* @param message Descriptive error message.
* @param problem Original compilation problem.
*/
public CompilerError(final String message, final IProblem problem) {
this.message = message;
this.problem = problem;
}
/**
* @return a String-ified representation of the error.
*/
@Override
public String toString() {
if (cause != null) {
return message + ": " + cause.toString();
} else if (problem != null) {
// Output as much data as possible
StringBuilder sb = new StringBuilder();
sb.append(problem.getOriginatingFileName());
sb.append("[" + problem.getSourceLineNumber() + "]");
sb.append("\n");
sb.append(problem.getMessage());
sb.append("[" + problem.getSourceStart());
sb.append("," + problem.getSourceEnd());
sb.append("]\n");
String[] args = problem.getArguments();
for (int i = 0; i < args.length; i++) {
String arg = args[i];
sb.append("[" + i + "] ");
sb.append(arg);
sb.append("\n");
}
return sb.toString();
} else {
return message;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy