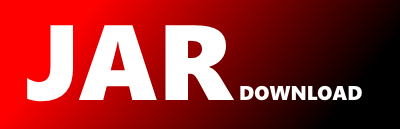
org.ow2.jonas.eclipse.compiler.JOnASCompilationUnit Maven / Gradle / Ivy
/**
* JOnAS: Java(TM) Open Application Server
* Copyright (C) 2007 Bull S.A.S.
* Contact: [email protected]
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA
*
* --------------------------------------------------------------------------
* $Id: JOnASCompilationUnit.java 15428 2008-10-07 11:20:29Z sauthieg $
* --------------------------------------------------------------------------
*/
package org.ow2.jonas.eclipse.compiler;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.util.List;
import org.eclipse.jdt.internal.compiler.env.ICompilationUnit;
/**
* JOnAS glue for Eclipse compiler.
* @author Guillaume Sauthier
*/
public class JOnASCompilationUnit implements ICompilationUnit {
/**
* Fully qualified class name (x.y.Z).
*/
private String className;
/**
* Java source file.
*/
private String sourceFile;
/**
* Compilations errors.
*/
private List errors;
/**
* Cache the file content, as it is requested twice.
*/
private char[] cachedContent = null;
/**
* Compilation Context.
*/
private CompilationContext context;
/**
* Creates a new {@link ICompilationUnit} targeting a given class.
* @param className target classname.
* @param sourceFile input source file.
* @param context Compilation context.
* @param errors errors holder.
*/
public JOnASCompilationUnit(final String className,
final String sourceFile,
final CompilationContext context,
final List errors) {
super();
this.className = className;
this.sourceFile = sourceFile;
this.context = context;
this.errors = errors;
}
/**
* @return the file's content as a char array.
* @see org.eclipse.jdt.internal.compiler.env.ICompilationUnit#getContents()
*/
public char[] getContents() {
if (cachedContent == null) {
try {
// load cache
cachedContent = Util.toCharArray(new FileInputStream(new File(sourceFile)),
context.getEncoding());
} catch (FileNotFoundException e) {
errors.add(new CompilerError(className, e));
return null;
}
}
// use cache
return cachedContent;
}
/**
* @return the non qualified Java classname.
* @see org.eclipse.jdt.internal.compiler.env.ICompilationUnit#getMainTypeName()
*/
public char[] getMainTypeName() {
int dot = className.lastIndexOf('.');
if (dot > 0) {
return className.substring(dot + 1).toCharArray();
}
return className.toCharArray();
}
/**
* @return the package name (without the type name).
* @see org.eclipse.jdt.internal.compiler.env.ICompilationUnit#getPackageName()
*/
public char[][] getPackageName() {
String[] parts = className.split("\\.");
char[][] result = new char[parts.length - 1][];
for (int i = 0; i < result.length; i++) {
result[i] = parts[i].toCharArray();
}
return null;
}
/**
* @return the filename.
* @see org.eclipse.jdt.internal.compiler.env.IDependent#getFileName()
*/
public char[] getFileName() {
return sourceFile.toCharArray();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy