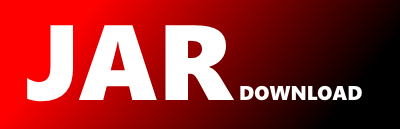
org.ow2.jonas.ant.JOnASAntTool Maven / Gradle / Ivy
/**
* JOnAS: Java(TM) Open Application Server
* Copyright (C) 2005-2008 Bull S.A.S.
* Contact: [email protected]
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307
* USA
*
* --------------------------------------------------------------------------
* $Id: JOnASAntTool.java 15428 2008-10-07 11:20:29Z sauthieg $
* --------------------------------------------------------------------------
*/
package org.ow2.jonas.ant;
import java.io.File;
import org.ow2.jonas.ant.jonasbase.Apps;
import org.ow2.jonas.ant.jonasbase.Archives;
import org.ow2.jonas.ant.jonasbase.Ejbjars;
import org.ow2.jonas.ant.jonasbase.Rars;
import org.ow2.jonas.ant.jonasbase.Wars;
import org.apache.tools.ant.Project;
import org.apache.tools.ant.Task;
import org.apache.tools.ant.types.Environment;
import org.apache.tools.ant.types.FileSet;
import org.apache.tools.ant.types.Environment.Variable;
import org.apache.tools.ant.types.PatternSet.NameEntry;
/**
* Common constants or methods used by several classes of the package.
* @author Benoit Pelletier
*/
public final class JOnASAntTool {
/**
* List of Wars to copy for each JONAS_BASE.
*/
public static final String[] WARS_LIST = new String[] {"jonasAdmin.war"};
/**
* List of Rars to copy for each JONAS_BASE.
*/
public static final String[] RARS_LIST = new String[] {"jonas-jca-jdbc-cp.rar",
"jonas-jca-jdbc-dm.rar",
"jonas-jca-jdbc-ds.rar",
"jonas-jca-jdbc-xa.rar"};
/**
* List of EjbJars to copy for each JONAS_BASE.
*/
public static final String[] EJBJARS_LIST = new String[] {""};
/**
* List of Apps to copy for each JONAS_BASE.
*/
public static final String[] APPS_LIST = new String[] {""};
/**
* Private constructor for tool class.
*/
private JOnASAntTool() {
}
/**
* Update the JONAS_BASE directory with ejbjars, webapps, rars, etc.
* @param antTask target task
* @param jonasRoot JONAS_ROOT
* @param destDir destination directory
**/
public static void updateJonasBase(final Task antTask,
final File jonasRoot,
final File destDir) {
Archives wars = new Wars();
updateJonasBaseForArchives(antTask, jonasRoot, destDir, wars, "deploy", WARS_LIST);
Archives ejbjars = new Ejbjars();
updateJonasBaseForArchives(antTask, jonasRoot, destDir, ejbjars, "deploy", EJBJARS_LIST);
Archives rars = new Rars();
updateJonasBaseForArchives(antTask, jonasRoot, destDir, rars, "deploy", RARS_LIST);
Archives apps = new Apps();
updateJonasBaseForArchives(antTask, jonasRoot, destDir, apps, "deploy", APPS_LIST);
}
/**
* Update JONAS_BASE with given archives with lists of includes.
* @param antTask target task
* @param jonasRoot JONAS_ROOT
* @param destDir destination directory
* @param archives tasks (webapps, rars, ejbjars)
* @param folderName where to put files
* @param listOfIncludes files to include
*/
public static void updateJonasBaseForArchives(final Task antTask,
final File jonasRoot,
final File destDir,
final Archives archives,
final String folderName,
final String[] listOfIncludes) {
configure(antTask, archives);
archives.setDestDir(destDir);
FileSet fileSet = new FileSet();
fileSet.setDir(new File(jonasRoot, folderName));
for (int f = 0; f < listOfIncludes.length; f++) {
NameEntry ne = fileSet.createInclude();
ne.setName(listOfIncludes[f]);
}
archives.addFileset(fileSet);
archives.setOverwrite(true);
String info = archives.getLogInfo();
if (info != null) {
antTask.log(info, Project.MSG_INFO);
}
archives.execute();
}
/**
* Configure the given task by setting name, project root, etc.
* @param srcTask source task
* @param dstTask destination task
*/
public static void configure(final Task srcTask, final Task dstTask) {
dstTask.setTaskName(srcTask.getTaskName());
dstTask.setProject(srcTask.getProject());
}
/**
* Delete a file. If the file is a directory, delete recursively all the
* files inside.
* @param aFile file to delete.
*/
public static void deleteAllFiles(final File aFile) {
if (aFile.isDirectory()) {
File[] someFiles = aFile.listFiles();
for (int i = 0; i < someFiles.length; i++) {
deleteAllFiles(someFiles[i]);
}
}
aFile.delete();
}
/**
* Create an Ant {@link Variable} configured with a simple value.
* @param key variable name
* @param value variable value
* @return a configured {@link Variable}
*/
public static Environment.Variable createVariable(final String key, final String value) {
Environment.Variable variable = new Environment.Variable();
variable.setKey(key);
variable.setValue(value);
return variable;
}
/**
* Create an Ant {@link Variable} configured with an absolute File path as value.
* @param key variable name
* @param value variable value
* @return a configured {@link Variable}
*/
public static Environment.Variable createVariable(final String key, final File value) {
Environment.Variable variable = new Environment.Variable();
variable.setKey(key);
variable.setFile(value);
return variable;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy