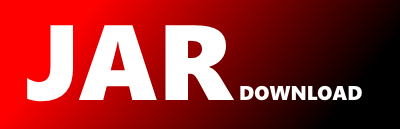
org.ow2.jonas.ant.JOnASTask Maven / Gradle / Ivy
/**
* JOnAS: Java(TM) Open Application Server
* Copyright (C) 2004-2008 Bull S.A.S.
* Contact: [email protected]
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307
* USA
*
* --------------------------------------------------------------------------
* $Id: JOnASTask.java 15428 2008-10-07 11:20:29Z sauthieg $
* --------------------------------------------------------------------------
*/
package org.ow2.jonas.ant;
import org.apache.tools.ant.taskdefs.Java;
/**
* Uses to start/stop/... JOnAS
* @author Florent Benoit
*/
public class JOnASTask extends BootstrapTask {
/**
* Stop class (Admin class).
*/
private static final String ADMIN_CLASS = "org.ow2.jonas.commands.admin.ClientAdmin";
/**
* Check Environment class.
*/
private static final String CHECKENV_CLASS = "org.ow2.jonas.commands.check.CheckEnv";
/**
* Version class.
*/
private static final String VERSION_CLASS = "org.ow2.jonas.Version";
/**
* Enumeration of the different execution modes supported by this task.
*/
private enum Mode {
/**
* (START) mode.
*/
START {
/**
* {@inheritDoc}
*/
public void configureJava(final Java task) {
task.clearArgs();
task.setClassname(ADMIN_CLASS);
task.createArg().setValue("-start");
}
/**
* {@inheritDoc}
*/
public String getMainClass() {
return ADMIN_CLASS;
}
},
/**
* (STOP) mode.
*/
STOP {
/**
* {@inheritDoc}
*/
public void configureJava(final Java task) {
task.createArg().setValue("-halt");
}
/**
* {@inheritDoc}
*/
public String getMainClass() {
return ADMIN_CLASS;
}
},
/**
* (JNDI) mode (print jndi names of the registry).
*/
JNDI {
/**
* {@inheritDoc}
*/
public void configureJava(final Java task) {
task.createArg().setValue("-j");
}
/**
* {@inheritDoc}
*/
public String getMainClass() {
return ADMIN_CLASS;
}
},
/**
* (CHECK) mode.
*/
CHECK {
/**
* {@inheritDoc}
*/
public void configureJava(final Java task) {
// nothing special to do
}
/**
* {@inheritDoc}
*/
public String getMainClass() {
return CHECKENV_CLASS;
}
},
/**
* (VERSION) mode.
*/
VERSION {
/**
* {@inheritDoc}
*/
public void configureJava(final Java task) {
// nothing special to do
}
/**
* {@inheritDoc}
*/
public String getMainClass() {
return VERSION_CLASS;
}
},
/**
* (PING) mode.
*/
PING {
/**
* {@inheritDoc}
*/
public void configureJava(final Java task) {
task.createArg().setValue("-ping");
}
/**
* {@inheritDoc}
*/
public String getMainClass() {
return ADMIN_CLASS;
}
};
/**
* Configure the given {@link Java} task according to the execution mode.
* @param task {@link Java} task to be configured
*/
public abstract void configureJava(final Java task);
/**
* @return the main class to be used by the {@link Java} task.
*/
public abstract String getMainClass();
}
/**
* Mode (start or stop).
* START is default mode
*/
private Mode mode = Mode.START;
/**
* Run the task.
* @see org.apache.tools.ant.Task#execute()
*/
public void execute() {
// Start the task
Java bootstrapTask = getBootstraptask(mode.getMainClass());
mode.configureJava(bootstrapTask);
bootstrapTask.executeJava();
}
/**
* Sets the mode for the server (start or stop).
* @param mode The mode to set.
*/
public void setMode(final String mode) {
// This enum.valueOf may throw an IllegalArgumentException if the specified mode is incorrect
this.mode = Mode.valueOf(mode.toUpperCase());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy