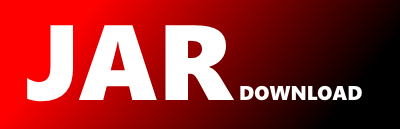
org.ow2.jonas.ant.cluster.DiscoveryCluster Maven / Gradle / Ivy
/**
* JOnAS: Java(TM) Open Application Server
* Copyright (C) 2005 Bull S.A.
* Contact: [email protected]
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307
* USA
*
* Initial developer: Benoit Pelletier
* --------------------------------------------------------------------------
* $Id: DiscoveryCluster.java 15428 2008-10-07 11:20:29Z sauthieg $
* --------------------------------------------------------------------------
*/
package org.ow2.jonas.ant.cluster;
import java.io.File;
import java.util.Iterator;
import org.apache.tools.ant.BuildException;
import org.apache.tools.ant.Task;
import org.ow2.jonas.ant.jonasbase.BaseTaskItf;
import org.ow2.jonas.ant.jonasbase.Discovery;
/**
* Define DiscoveryCluster task
* @author Benoit Pelletier
*/
public class DiscoveryCluster extends ClusterTasks {
/**
* Info for the logger
*/
private static final String INFO = "[DiscoveryCluster] ";
/**
* greeting ports range
*/
private String[] greetingPortRange = null;
/**
* source ports range
*/
private String[] sourcePortRange = null;
/**
* multicast port
*/
private String mcastPort = null;
/**
* multicast addr
*/
private String mcastAddr = null;
/**
* ind of master node
*/
private int masterNode = -1;
/**
* jonas_root
*/
private String jonasRoot = null;
/**
* domain name
*/
private String domainName = null;
/**
* protocol
*/
private String protocol = null;
/**
* carol ports range
*/
private String[] carolPortRange = null;
/**
* domain desc
*/
private String domainDesc = null;
/**
* cluster name
*/
private String clusterName = null;
/**
* cluster desc
*/
private String clusterDesc = null;
/**
* nodes name
*/
private String clusterNodesName = null;
/**
* cluster daemon name
*/
private String cdName = null;
/**
* cluster daemon protocol
*/
private String cdProtocol = null;
/**
* cluster daemon port
*/
private String cdPort = null;
/**
* Default constructor
*/
public DiscoveryCluster() {
super();
}
/**
* Set master node
* @param masterNode inf of the master node
*/
public void setMasterNode(int masterNode) {
this.masterNode = masterNode;
}
/**
* Set mcastPort
* @param mcastPort multicast port to set
*/
public void setMcastPort(String mcastPort) {
this.mcastPort = mcastPort;
}
/**
* Set mcastAddr
* @param mcastAddr multicast address to set
*/
public void setMcastAddr(String mcastAddr) {
this.mcastAddr = mcastAddr;
}
/**
* Set greeting ports range
* @param portRange ports range
*/
public void setGreetingPortRange(String portRange) {
this.greetingPortRange = portRange.split(",");
}
/**
* Set source ports range
* @param portRange ports range
*/
public void setSourcePortRange(String portRange) {
this.sourcePortRange = portRange.split(",");
}
/**
* Set domainName
* @param domainName domain name
*/
public void setDomainName(String domainName) {
this.domainName = domainName;
}
/**
* Set domainDesc
* @param domainDesc domain desc
*/
public void setDomainDesc(String domainDesc) {
this.domainDesc = domainDesc;
}
/**
* Set clusterName
* @param clusterName cluster name
*/
public void setClusterName(String clusterName) {
this.clusterName = clusterName;
}
/**
* Set clusterDesc
* @param clusterDesc cluster desc
*/
public void setClusterDesc(String clusterDesc) {
this.clusterDesc = clusterDesc;
}
/**
* Generates the discovery tasks for each JOnAS's instances
*/
public void generatesTasks() {
int portInd = 0;
for (int i = getDestDirSuffixIndFirst(); i <= getDestDirSuffixIndLast(); i++) {
String destDir = getDestDir(getDestDirPrefix(), i);
log(INFO + "tasks generation for " + destDir);
// creation of the Discovery tasks
Discovery discovery = new Discovery();
if (i == masterNode) {
discovery.setJonasRoot(jonasRoot);
discovery.setSourcePort(sourcePortRange[portInd]);
discovery.setDomainName(domainName);
discovery.setDomainDesc(domainDesc);
discovery.setDomainCluster(clusterName, clusterDesc, clusterNodesName, getWebInstNb() + getEjbInstNb(), protocol, carolPortRange, cdName, getCdUrl());
}
discovery.setGreetingPort(greetingPortRange[portInd]);
discovery.setMcastPort(mcastPort);
discovery.setMcastAddr(mcastAddr);
// set destDir for each task
for (Iterator it = discovery.getTasks().iterator(); it.hasNext();) {
BaseTaskItf task = (BaseTaskItf) it.next();
task.setDestDir(new File(destDir));
}
addTasks(discovery);
portInd++;
}
}
/**
* set the name prefix for the cluster nodes
* @param clusterNodesName prefix of the nodes names in the cluster
*/
public void setClusterNodesName(String clusterNodesName) {
this.clusterNodesName = clusterNodesName;
}
/**
* Set the protocol used by the server nodes
* @param protocol protocol
*/
public void setProtocol(String protocol) {
this.protocol = protocol;
}
/**
* Set the name used by the cluster daemon
* @param cdName cdName
*/
public void setCdName(String cdName) {
this.cdName = cdName;
}
/**
* Set the protocol used by the cluster daemon
* @param cdProtocol cdProtocol
*/
public void setCdProtocol(String cdProtocol) {
this.cdProtocol = cdProtocol;
}
/**
* Set the port used by the cluster daemon
* @param cdPort cdPort
*/
public void setCdPort(String cdPort) {
this.cdPort = cdPort;
}
/**
* Build the cluster daemon JRM remote url
* @return url
*/
public String getCdUrl(){
String scheme;
if(cdProtocol.equals("jrmp") || cdProtocol.equals("irmi")) {
scheme = "rmi";
} else if(cdProtocol.equals("iiop")) {
scheme = "iiop";
} else {
throw new BuildException(INFO + "Unknown protocol for cluster daemon: " + cdProtocol);
}
String url = "service:jmx:" + scheme + "://localhost/jndi/"
+ scheme + "://localhost:" + cdPort + "/" + cdProtocol + "connector_" + cdName;
return url;
}
/**
* set the carol port range
* @param carolPortRange carol port range
*/
public void setCarolPortRange(String carolPortRange) {
this.carolPortRange = carolPortRange.split(",");
}
/**
* Set JONAS ROOT
* @param jonasRoot directory
*/
public void setJonasRoot(String jonasRoot) {
this.jonasRoot = jonasRoot;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy