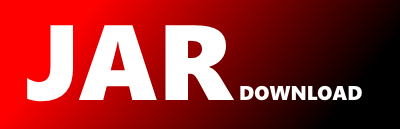
org.ow2.jonas.ant.jonasbase.Carol Maven / Gradle / Ivy
/**
* JOnAS: Java(TM) Open Application Server
* Copyright (C) 2004-2008 Bull S.A.S.
* Contact: [email protected]
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307
* USA
*
* --------------------------------------------------------------------------
* $Id: Carol.java 15428 2008-10-07 11:20:29Z sauthieg $
* --------------------------------------------------------------------------
*/
package org.ow2.jonas.ant.jonasbase;
import java.io.File;
import org.ow2.jonas.ant.JOnASBaseTask;
/**
* Allow to configure the properties in carol.properties file.
*
* carol.protocols
* rmi.local.registry
* rmi.local.call
* carol.start.cmi
* cmi.server.provider.urls
* @author Florent Benoit
* @author coqp
*
*/
public class Carol extends JTask implements BaseTaskItf {
/**
* Info for the logger.
*/
private static final String INFO = "[Carol] ";
/**
* Property separators (4 spaces).
*/
public static final String SEPARATOR = "=";
/**
* Default Host.
*/
private static final String DEFAULT_HOST = "localhost";
/**
* Default protocol header.
*/
private static final String PROTOCOL_HEADER = "://";
/**
* Default jrmp port number.
*/
private static final String DEFAULT_JRMP_PORT = "1099";
/**
* Default iiop port number.
*/
private static final String DEFAULT_IIOP_PORT = "2001";
/**
* Default irmi port number.
*/
private static final String DEFAULT_IRMI_PORT = "1098";
/**
* Protocols property.
*/
private static final String PROTOCOLS_PROPERTY = "carol.protocols";
/**
* Carol JRMP property.
*/
private static final String JRMP_PROPERTY = "carol.jrmp.url";
/**
* Carol IIOP property.
*/
private static final String IIOP_PROPERTY = "carol.iiop.url";
/**
* Carol IRMI property.
*/
private static final String IRMI_PROPERTY = "carol.irmi.url";
/**
* Optimization.
*/
private static final String OPTIMIZATION_PROPERTY = "carol.jvm.rmi.local.call";
/**
* Local Registry.
*/
private static final String LOCALREGISTRY_PROPERTY = "carol.jvm.rmi.local.registry";
/**
* Start CMI.
*/
public static final String START_CMI_PROPERTY = "carol.start.cmi";
/**
* Sequence of associations between a protocol and a list of provider URLs.
*/
public static final String SEQ_PROTOCOLS_TO_PROVIDER_URLS = "cmi.server.provider.urls";
/**
* List of provider URLs.
*/
private String serverproviderurls = null;
/**
* connection url for jrmp.
*/
private String jrmpconnectionurl = "rmi"+PROTOCOL_HEADER+DEFAULT_HOST+":"+DEFAULT_JRMP_PORT;
/**
* connection url for iiop.
*/
private String iiopconnectionurl = "iiop"+PROTOCOL_HEADER+DEFAULT_HOST+":"+DEFAULT_IIOP_PORT;
/**
* connection url for irmi.
*/
private String irmiconnectionurl = "rmi"+PROTOCOL_HEADER+DEFAULT_HOST+":"+DEFAULT_IRMI_PORT;
/**
* for starting cmi automatically.
*/
private Boolean startcmi = false;
/**
* local call with jrmp optimisation.
*/
private Boolean rmilocalcall = false;
/**
* local registry optimisation.
*/
private Boolean rmilocalregistry = true;
/**
* jonas rmi activation (iiop, irmi, jrmp).
*/
private String carolprotocols = null;
/**
* host.
*/
private String host = DEFAULT_HOST;
/**
* Default constructor.
*/
public Carol() {
super();
}
/**
* Set the connection url for JRMP.
*
* @param portNumber
* port for JRMP
*/
public void setJrmpPort(final String portNumber) {
jrmpconnectionurl = "rmi" + PROTOCOL_HEADER + host + ":" + portNumber;
}
/**
* Set the connection url for IIOP.
*
* @param portNumber
* port for IIOP
*/
public void setIiopPort(final String portNumber) {
iiopconnectionurl = "iiop" + PROTOCOL_HEADER + host + ":" + portNumber;
}
/**
* Set the connection url for IRMI.
*
* @param portNumber
* port for IRMI
*/
public void setIrmiPort(final String portNumber) {
irmiconnectionurl = "rmi" + PROTOCOL_HEADER + host + ":" + portNumber;
}
/**
* Set the port for all protocols.
*
* @param portNumber
* port for all protocols
*/
public void setDefaultPort(final String portNumber) {
setJrmpPort(portNumber);
setIiopPort(portNumber);
setIrmiPort(portNumber);
}
/**
* Set the initial protocols when JOnAS start.
*
* @param protocols
* comma separated list of protocols
*/
public void setProtocols(final String protocols) {
this.carolprotocols = protocols;
}
/**
* Set the host.
*
* @param host hostname
*
*/
public void setHost(final String host) {
this.host = host;
}
/**
* Enable or disable optimization.
*
* @param enabled
* (true or false)
* the default value is currently false in carol.properties
*/
public void setJrmpOptimization(final boolean enabled) {
this.rmilocalcall = enabled;
}
/**
* Enable or disable local registry.
*
* @param enabled
* (true or false)
* the default value is currently true in carol.properties
*/
public void setLocalRegistry(final boolean enabled) {
this.rmilocalregistry = enabled;
}
/**
* Enable or disable the use of CMI.
* @param enabled (true or false)
* the default value is currently false in carol.properties
*/
public void setCmiStarted(final boolean enabled) {
this.startcmi = enabled;
}
/**
* Set the sequence of protocols and provider urls.
* @param seqProtocol2ProviderURLs a sequence of protocols and provider urls
*/
public void setClusterViewProviderUrls(final String seqProtocol2ProviderURLs) {
this.serverproviderurls = seqProtocol2ProviderURLs;
}
/**
* Execute this task.
*/
@Override
public void execute() {
// Path to JONAS_BASE
String jBaseConf = getDestDir().getPath() + File.separator + "conf";
changeValueForKey(INFO,
jBaseConf,
JOnASBaseTask.CAROL_CONF_FILE,
PROTOCOLS_PROPERTY,
carolprotocols,
SEPARATOR,
false); // replace
changeValueForKey(INFO,
jBaseConf,
JOnASBaseTask.CAROL_CONF_FILE,
OPTIMIZATION_PROPERTY,
rmilocalcall.toString(),
SEPARATOR,
false); // replace
changeValueForKey(INFO,
jBaseConf,
JOnASBaseTask.CAROL_CONF_FILE,
LOCALREGISTRY_PROPERTY,
rmilocalregistry.toString(),
SEPARATOR,
false); // replace
changeValueForKey(INFO,
jBaseConf,
JOnASBaseTask.CAROL_CONF_FILE,
START_CMI_PROPERTY,
startcmi.toString(),
SEPARATOR,
false); // replace
changeValueForKey(INFO,
jBaseConf,
JOnASBaseTask.CAROL_CONF_FILE,
JRMP_PROPERTY ,
jrmpconnectionurl ,
SEPARATOR,
false); // replace
changeValueForKey(INFO,
jBaseConf,
JOnASBaseTask.CAROL_CONF_FILE,
IIOP_PROPERTY ,
iiopconnectionurl ,
SEPARATOR,
false); // replace
changeValueForKey(INFO,
jBaseConf,
JOnASBaseTask.CAROL_CONF_FILE,
IRMI_PROPERTY ,
irmiconnectionurl ,
SEPARATOR,
false); // replace
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy