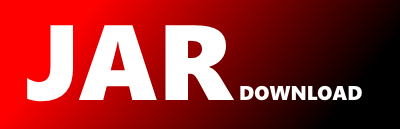
org.ow2.jonas.ant.jonasbase.JmsRa Maven / Gradle / Ivy
/**
* JOnAS: Java(TM) Open Application Server
* Copyright (C) 2004-2008 Bull S.A.S.
* Contact: [email protected]
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307
* USA
*
* --------------------------------------------------------------------------
* $Id: JmsRa.java 15428 2008-10-07 11:20:29Z sauthieg $
* --------------------------------------------------------------------------
*/
package org.ow2.jonas.ant.jonasbase;
import java.io.File;
import java.util.Date;
import org.apache.tools.ant.BuildException;
import org.apache.tools.ant.taskdefs.Copy;
import org.apache.tools.ant.taskdefs.Expand;
import org.apache.tools.ant.taskdefs.Java;
import org.apache.tools.ant.types.FileSet;
import org.apache.tools.ant.types.Path;
import org.apache.tools.ant.types.selectors.FilenameSelector;
import org.ow2.jonas.ant.JOnASAntTool;
/**
* Allow to create/adapt the JMS resource adaptor.
* @author Benoit Pelletier
*/
public class JmsRa extends JTask implements BaseTaskItf {
/**
* Info for the logger.
*/
private static final String INFO = "[JmsRa] ";
/**
* Name of the rar.
*/
private static final String JONAS_JORAMRA = "joram_ra_for_jonas.rar";
/**
* RAConfig classname.
*/
private static final String RACONFIG_CLASS = "org.ow2.jonas.generators.raconfig.RAConfig";
/**
* Default port number.
*/
private static final String DEFAULT_PORT = "16010";
/**
* Port number of the server.
*/
private String serverPort = DEFAULT_PORT;
/**
* Set the port number.
* @param serverPort the port nb
*/
public void setServerPort(final String serverPort) {
this.serverPort = serverPort;
}
/**
* Execute this task.
*/
@Override
public void execute() {
// Build new temp file for making a resource adaptor
File tmpFile = new File(System.getProperty("java.io.tmpdir"), String.valueOf(new Date().getTime()));
// Copy the RAR file from JONAS_ROOT to JONAS_BASE
String srcRarDir = getJonasRoot().getPath() + File.separator + "deploy";
String dstRarDir = getDestDir().getPath() + File.separator + "deploy";
String dstRarFileName = dstRarDir + File.separator + JONAS_JORAMRA;
Copy copy = new Copy();
JOnASAntTool.configure(this, copy);
copy.setTodir(new File(dstRarDir));
FilenameSelector fns1 = new FilenameSelector();
fns1.setName(JONAS_JORAMRA);
FileSet fs1 = new FileSet();
fs1.setDir(new File(srcRarDir));
fs1.addFilename(fns1);
copy.addFileset(fs1);
copy.setOverwrite(true);
try {
copy.execute();
} catch (Exception rae) {
rae.printStackTrace();
JOnASAntTool.deleteAllFiles(tmpFile);
throw new BuildException(INFO + "Cannot copy " + JONAS_JORAMRA + " file : ", rae);
}
// Extract the jonas-ra.xml
Expand jarTask = new Expand();
JOnASAntTool.configure(this, jarTask);
jarTask.setDest(tmpFile);
jarTask.setSrc(new File(dstRarFileName));
try {
jarTask.execute();
} catch (Exception rae) {
rae.printStackTrace();
JOnASAntTool.deleteAllFiles(tmpFile);
throw new BuildException(INFO + "Cannot extract jonas-ra.xml with jar command : ", rae);
}
// update the jonas-ra.xml
Replace replaceTask = new Replace();
JOnASAntTool.configure(this, replaceTask);
replaceTask.setFile(new File(tmpFile.getPath() + File.separator + "META-INF/jonas-ra.xml"));
String token = "ServerPort "
+ "\n"
+ " ";
String value = "ServerPort "
+ "\n"
+ " " + serverPort + " ";
replaceTask.setToken(token);
replaceTask.setValue(value);
try {
replaceTask.execute();
} catch (Exception rae) {
rae.printStackTrace();
JOnASAntTool.deleteAllFiles(tmpFile);
throw new BuildException(INFO + "Cannot replace port number in the jonas-ra.xml file : ", rae);
}
// update the archive
Java raConfigTask = getBootstraptask("", false);
// Add the ra-config Jar in the Java classpath path
String clientJar = getJonasRoot().getPath() + File.separator + "lib" + File.separator + "client.jar";
String raConfigJar = getJonasRoot().getPath() + File.separator + "lib" + File.separator + "jonas-generators-raconfig.jar";
Path classpath = new Path(raConfigTask.getProject(), clientJar);
classpath.append(new Path(raConfigTask.getProject(), raConfigJar));
raConfigTask.setClasspath(classpath);
JOnASAntTool.configure(this, raConfigTask);
raConfigTask.clearArgs();
raConfigTask.createArg().setValue(RACONFIG_CLASS);
raConfigTask.createArg().setValue("-u");
raConfigTask.createArg().setValue(tmpFile.getPath() + File.separator + "META-INF/jonas-ra.xml");
raConfigTask.createArg().setValue(dstRarFileName);
try {
raConfigTask.execute();
} catch (Exception rae) {
rae.printStackTrace();
throw new BuildException(INFO + "Cannot make a resource adaptor on RAConfig: ", rae);
} finally {
JOnASAntTool.deleteAllFiles(tmpFile);
}
log(INFO + "Setting Port number to :" + serverPort + " in the rar '" + dstRarFileName + "'.");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy