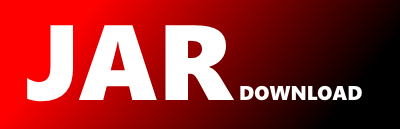
org.ow2.jonas.ant.cluster.CarolCluster Maven / Gradle / Ivy
/**
* JOnAS: Java(TM) Open Application Server
* Copyright (C) 2005-2007 Bull S.A.S.
* Contact: [email protected]
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307
* USA
*
* Initial developer: Benoit Pelletier
* --------------------------------------------------------------------------
* $Id: CarolCluster.java 15428 2008-10-07 11:20:29Z sauthieg $
* --------------------------------------------------------------------------
*/
package org.ow2.jonas.ant.cluster;
import java.io.File;
import org.apache.tools.ant.BuildException;
import org.ow2.jonas.ant.jonasbase.Carol;
/**
* Define CarolCluster task.
* @author Benoit Pelletier
*/
public class CarolCluster extends ClusterTasks {
/**
* Info for the logger.
*/
private static final String INFO = "[CarolCluster] ";
/**
* Ports range.
*/
private String[] portRange = null;
/**
* Protocols.
*/
private String protocols = null;
/**
* Jrmp optimization.
*/
private boolean jrmpOptimization = false;
/**
* Default constructor
*/
public CarolCluster() {
super();
}
/**
* Set protocols.
* @param protocols protocols to set
*/
public void setProtocols(final String protocols) {
this.protocols = protocols;
}
/**
* Set ports range.
* @param portRange ports range
*/
public void setPortRange(final String portRange) {
this.portRange = portRange.split(",");
}
/**
* Set jrmp optimization.
* @param jrmpOptimization jrmp optimization
*/
public void setJrmpOptimization(final boolean jrmpOptimization) {
this.jrmpOptimization = jrmpOptimization;
}
/**
* Generates the carol tasks for each JOnAS's instances.
*/
@Override
public void generatesTasks() {
int portInd = 0;
for (int i = getDestDirSuffixIndFirst(); i <= getDestDirSuffixIndLast(); i++) {
String destDir = getDestDir(getDestDirPrefix(), i);
// creation of the Carol tasks
Carol carol = new Carol();
log(INFO + "tasks generation for " + destDir);
carol.setDefaultPort(portRange[portInd]);
carol.setProtocols(protocols);
carol.setJrmpOptimization(jrmpOptimization);
// Enable the clustering
carol.setCmiStarted(true);
//
int webIstNb = getWebInstNb();
if(getArch().equals(ARCH_SEPARATED_WEB_EJB) && i <= webIstNb) {
int ejbInstNb = getEjbInstNb();
String seqProtocol2ProviderURLs = "";
for(String protocol : protocols.split(",")) {
if(seqProtocol2ProviderURLs != "") {
seqProtocol2ProviderURLs += ";";
}
seqProtocol2ProviderURLs += protocol + "::";
if(protocol.equals("jrmp") || protocol.equals("irmi")) {
seqProtocol2ProviderURLs += "rmi://";
} else if(protocol.equals("iiop")) {
seqProtocol2ProviderURLs += "iiop://";
} else {
log(INFO + "Unknown protocol: " + protocol);
throw new BuildException("Unknown protocol: " + protocol);
}
for(int j = webIstNb ; j < webIstNb + ejbInstNb ; j++) {
if(j != webIstNb) {
seqProtocol2ProviderURLs += ",";
}
seqProtocol2ProviderURLs += "localhost:" + portRange[j];
}
}
carol.setClusterViewProviderUrls(seqProtocol2ProviderURLs);
}
// set destDir for carol task
carol.setDestDir(new File(destDir));
addTask(carol);
portInd++;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy