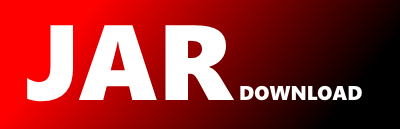
org.ow2.jonas.ant.cluster.ClusterTasks Maven / Gradle / Ivy
/**
* JOnAS: Java(TM) Open Application Server
* Copyright (C) 2005-2007 Bull S.A.S.
* Contact: [email protected]
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307
* USA
*
* Initial developer: Benoit Pelletier
* --------------------------------------------------------------------------
* $Id: ClusterTasks.java 15428 2008-10-07 11:20:29Z sauthieg $
* --------------------------------------------------------------------------
*/
package org.ow2.jonas.ant.cluster;
import java.util.ArrayList;
import java.util.List;
import org.ow2.jonas.ant.jonasbase.Tasks;
import org.apache.tools.ant.Task;
/**
* Defines a common cluster task
* @author Benoit Pelletier
*/
public abstract class ClusterTasks extends Tasks {
/**
* Architecture of the JOnAS's instances : contain both Web level and Ejb level.
*/
protected static final String ARCH_BOTH_WEB_EJB = "bothWebEjb";
/**
* Architecture of the JOnAS's instances : separate Web level and Ejb level.
*/
protected static final String ARCH_SEPARATED_WEB_EJB = "diffWebEjb";
/**
* Information for the logger.
*/
private String logInfo = null;
/**
* Prefix to the destination directory (JONAS_BASE).
*/
private String destDirPrefix = null;
/**
* First index for the destDir suffix.
*/
private int destDirSuffixIndFirst = -1;
/**
* Last index for the destDir suffix.
*/
private int destDirSuffixIndLast = -1;
/**
* Cluster tasks List.
*/
private List clusterTasks = null;
/**
* Cluster architecture : share instance for web/ejb or separate them.
*/
private String arch = null;
/**
* number of JOnAS's instance for web level
*/
private int webInstNb = -1;
/**
* number of JOnAS's instance for ejb level
*/
private int ejbInstNb = -1;
/**
* Root task set just for logging purpose.
*/
private Task rootTask = null;
/**
* Constructor.
*/
public ClusterTasks() {
clusterTasks = new ArrayList();
}
/**
* Set directory prefix.
* @param destDirPrefix The destDirPrefix to set.
*/
public void setDestDirPrefix(String destDirPrefix) {
this.destDirPrefix = destDirPrefix;
}
/**
* Gets the prefix of the destination directory.
* @return the prefix of the destination directory
*/
public String getDestDirPrefix() {
return destDirPrefix;
}
/**
* @param destDirSuffixIndFirst The destDirSuffixIndFirst to set.
*/
public void setDestDirSuffixIndFirst(int destDirSuffixIndFirst) {
this.destDirSuffixIndFirst = destDirSuffixIndFirst;
}
/**
* Gets the first index of the destDir suffix.
* @return the first index of the destDir suffix
*/
public int getDestDirSuffixIndFirst() {
return destDirSuffixIndFirst;
}
/**
* @param destDirSuffixIndLast The destDirSuffixIndLast to set.
*/
public void setDestDirSuffixIndLast(int destDirSuffixIndLast) {
this.destDirSuffixIndLast = destDirSuffixIndLast;
}
/**
* Gets the last index of the destDir suffix.
* @return the last index of the destDir suffix
*/
public int getDestDirSuffixIndLast() {
return destDirSuffixIndLast;
}
/**
* Gets logger info (to be displayed).
* @return logger info
* @see org.ow2.jonas.ant.jonasbase.BaseTaskItf#getLogInfo()
*/
public String getLogInfo() {
return logInfo;
}
/**
* Set the info to be displayed by the logger.
* @param logInfo information to be displayed
* @see org.ow2.jonas.ant.jonasbase.BaseTaskItf#setLogInfo(java.lang.String)
*/
public void setLogInfo(String logInfo) {
this.logInfo = logInfo;
}
/**
* Build dest dir with an index.
* @param destDirPrefix destination directory prefix
* @param i index
* @return destination directory
*/
public static String getDestDir(String destDirPrefix, int i) {
String destDir = destDirPrefix + i;
return destDir;
}
/**
* Set architecture.
* @param arch the architecture
*/
public void setArch(String arch) {
this.arch = arch;
}
/**
* Get architecture.
* @return architecture
*/
public String getArch() {
return arch;
}
/**
* Set the web instances number.
* @param webInstNb number of web instances
*/
public void setWebInstNb(final int webInstNb) {
this.webInstNb = webInstNb;
}
/**
* Get the web instances number.
* @return number of web instances
*/
public int getWebInstNb() {
return webInstNb;
}
/**
* Set the ejb instances number.
* @param ejbInstNb number of ejb instances
*/
public void setEjbInstNb(final int ejbInstNb) {
this.ejbInstNb = ejbInstNb;
}
/**
* Get the ejb instances number.
* @return number of ejb instances
*/
public int getEjbInstNb() {
return ejbInstNb;
}
/**
* Set root task.
* @param rootTask root task
*/
public void setRootTask(Task rootTask) {
this.rootTask = rootTask;
}
/**
* Get root Task.
* @return rootTask root task
*/
public Task getRootTask() {
return rootTask;
}
/**
* Add a task to the list of defined tasks.
* @param ct task to add
*/
public void addClusterTask(ClusterTasks ct) {
clusterTasks.add(ct);
}
/**
* @return a list of all tasks to do
*/
public List getClusterTasks() {
return clusterTasks;
}
/**
* Logging.
* @param msg message to log
*/
public void log(String msg) {
if (rootTask != null) {
rootTask.log(msg);
}
}
/**
* Abstract generatesTasks().
*/
public abstract void generatesTasks();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy