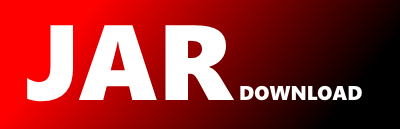
org.ow2.jonas.ant.cluster.Script Maven / Gradle / Ivy
/**
* JOnAS: Java(TM) Open Application Server
* Copyright (C) 2006-2009 Bull S.A.S.
* Contact: [email protected]
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307
* USA
*
* Initial developer: Benoit Pelletier
* --------------------------------------------------------------------------
* $Id: Script.java 20369 2010-09-24 15:36:20Z benoitf $
* --------------------------------------------------------------------------
*/
package org.ow2.jonas.ant.cluster;
import java.io.File;
import org.ow2.jonas.ant.jonasbase.JEcho;
import org.ow2.jonas.ant.jonasbase.JMkdir;
import org.ow2.jonas.ant.jonasbase.JTouch;
//import org.apache.tools.ant.taskdefs.Chmod;
/**
* Allow to launch the cluster nodes
* @author Benoit Pelletier
*/
public class Script extends ClusterTasks {
/**
* Info for the logger
*/
private static final String INFO = "[Script] ";
/**
* Name of the unix script file
*/
private static final String UNIX_SCRIPT_FILE = "jcl4sc";
/**
* Name of the unix script file
*/
private static final String WIN_SCRIPT_FILE = "jcl4sc.bat";
/**
* Script file Unix
*/
private File unixScriptFile = null;
/**
* Script file
*/
private File winScriptFile = null;
/**
* domain name
*/
private String domainName = null;
/**
* node name prefix
*/
private String nodeNamePrefix = null;
/**
* script dir
*/
private String scriptDir = null;
/**
* nodes nb
*/
private int instNb = 0;
/**
* cluster daemon directory
*/
private String cdDir = null;
/**
* db directory
*/
private String dbDir = null;
/**
* db instance name
*/
private String dbName = null;
/**
* master directory
*/
private String masterDir = null;
/**
* master instance name
*/
private String masterName = null;
/**
* Default constructor
*/
public Script() {
super();
}
/**
* Creation of the script files
* @param destDir destination directory
*/
public void createFiles(final String destDir) {
JMkdir mkdir = new JMkdir();
mkdir.setDestDir(new File(destDir));
addTask(mkdir);
// depending the os, the unix or the windows script is created
if (isOsWindows()) {
JTouch touchWin = new JTouch();
winScriptFile = new File(destDir + "/" + WIN_SCRIPT_FILE);
touchWin.setDestDir(winScriptFile);
addTask(touchWin);
} else {
JTouch touchUnix = new JTouch();
unixScriptFile = new File(destDir + "/" + UNIX_SCRIPT_FILE);
touchUnix.setDestDir(unixScriptFile);
addTask(touchUnix);
}
}
/**
* creation of the unix file
*/
private void flushUnixScriptFile() {
JEcho echo = new JEcho();
echo.setDestDir(unixScriptFile);
String contentFile = "\n"
+
"# -----------------------------------------------------------" + "\n"
+
"# start/stop/kill the cluster nodes" + "\n"
+
"# Note : " + "\n"
+
"# - JONAS_ROOT has to be set" + "\n"
+
"# - XWINDOWS can be set to 'yes' for starting the process in xterm" + "\n"
+
"# -----------------------------------------------------------" + "\n"
+
"\n"
+
"JCL_NUMBER_OF_NODES="
+
this.instNb + "\n"
+
"\n"
+
"JCL_CLUSTER_DAEMON_DIR="
+
this.cdDir + "\n"
+
"JCL_BASE_PREFIX="
+
this.getDestDirPrefix() + "\n"
+
"\n"
+
"JCL_NODE_NAME_PREFIX="
+
this.nodeNamePrefix + "\n"
+
"JCL_DOMAIN_NAME="
+
this.domainName + "\n"
+
"\n"
+
"JCL_DB_DIR="
+
this.dbDir + "\n"
+
"JCL_DB_NAME="
+
this.dbName + "\n"
+
"\n"
+
"JCL_MASTER_DIR="
+
this.masterDir + "\n"
+
"JCL_MASTER_NAME="
+
this.masterName + "\n"
+
"\n"
+
"export JCL_MASTER_NAME JCL_MASTER_DIR JCL_DB_NAME JCL_DB_DIR JCL_DOMAIN_NAME JCL_NODE_NAME_PREFIX"
+
"\n"
+
"export JCL_NUMBER_OF_NODES JCL_CLUSTER_DAEMON_DIR JCL_BASE_PREFIX"
+
"\n"
+
"$JONAS_ROOT/bin/jcl.sh $*" + "\n";
echo.setMessage(contentFile);
echo.setLogInfo(INFO + "Flushing Configuration in '" + unixScriptFile + "'");
addTask(echo);
}
/**
* creation of the windows file
*/
private void flushWinScriptFile() {
JEcho echo = new JEcho();
echo.setDestDir(winScriptFile);
String contentFile = "\n"
+
"@echo off" + "\n"
+
":: -----------------------" + "\n"
+
":: start/stop the cluster nodes" + "\n"
+
":: Note : JONAS_ROOT has to be set" + "\n"
+
":: -----------------------" + "\n"
+
"set JCL_NUMBER_OF_NODES="
+
this.instNb + "\n"
+
"set JCL_BASE_PREFIX="
+
this.getDestDirPrefix().replace('/', '\\') + "\n"
+
"set JCL_DOMAIN_NAME="
+
this.domainName + "\n"
+
"set JCL_NODE_NAME_PREFIX="
+
this.nodeNamePrefix + "\n"
+
"set JCL_CLUSTER_DAEMON_DIR="
+
this.cdDir + "\n"
+
"set JCL_DB_DIR="
+
this.dbDir + "\n"
+
"set JCL_DB_NAME="
+
this.dbName + "\n"
+
"set JCL_MASTER_DIR="
+
this.masterDir + "\n"
+
"set JCL_MASTER_NAME="
+
this.masterName + "\n"
+
"%JONAS_ROOT%\\bin\\jcl.bat %*" + "\n";
echo.setMessage(contentFile);
echo.setLogInfo(INFO + "Flushing Configuration in '" + winScriptFile + "'");
addTask(echo);
}
/**
* Generation of the config files
*/
public void flushFiles() {
if (isOsWindows()) {
flushWinScriptFile();
} else {
flushUnixScriptFile();
}
}
/**
* Set domainName
* @param domainName domain name
*/
public void setDomainName(final String domainName) {
this.domainName = domainName;
}
/**
* Set nodeNamePrefix
* @param nodeNamePrefix node name prefix
*/
public void setNodeNamePrefix(final String nodeNamePrefix) {
this.nodeNamePrefix = nodeNamePrefix;
}
/**
* Set script directory
* @param scriptDir Script directory
*/
public void setScriptDir(final String scriptDir) {
this.scriptDir = scriptDir;
}
/**
* Set nodes number
* @param instNb nodes nb
*/
public void setInstNb(final int instNb) {
this.instNb = instNb;
}
/**
* cluster daemon directory
* @param cdDir directory
*/
public void setCdDir(final String cdDir) {
this.cdDir = cdDir;
if (File.separatorChar == '/') {
this.cdDir = this.cdDir.replace('\\', File.separatorChar);
} else {
this.cdDir = this.cdDir.replace('/', File.separatorChar);
}
}
/**
* db instance directory
* @param dbDir directory
*/
public void setDbDir(final String dbDir) {
this.dbDir = dbDir;
if (File.separatorChar == '/') {
this.dbDir = this.dbDir.replace('\\', File.separatorChar);
} else {
this.dbDir = this.dbDir.replace('/', File.separatorChar);
}
}
/**
* db instance name
* @param dbName name
*/
public void setDbName(final String dbName) {
this.dbName = dbName;
}
/**
* master instance directory
* @param dbDir directory
*/
public void setMasterDir(final String masterDir) {
this.masterDir = masterDir;
if (File.separatorChar == '/') {
this.masterDir = this.masterDir.replace('\\', File.separatorChar);
} else {
this.masterDir = this.masterDir.replace('/', File.separatorChar);
}
}
/**
* master instance name
* @param dbName name
*/
public void setMasterName(final String masterName) {
this.masterName = masterName;
}
/**
* Generates the script tasks
*/
@Override
public void generatesTasks() {
// creation of the Script tasks
createFiles(scriptDir);
flushFiles();
}
/**
* @return true if the os.name starts with "Windows"
*/
public static boolean isOsWindows() {
String osName = System.getProperty("os.name", "");
return (osName.startsWith("Windows"));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy