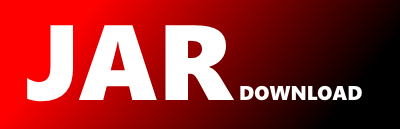
org.ow2.jonas.ant.jonasbase.Jms Maven / Gradle / Ivy
/**
* JOnAS: Java(TM) Open Application Server
* Copyright (C) 2004-2008 Bull S.A.S.
* Contact: [email protected]
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307
* USA
*
* --------------------------------------------------------------------------
* $Id: Jms.java 18196 2009-08-07 14:39:52Z alitokmen $
* --------------------------------------------------------------------------
*/
package org.ow2.jonas.ant.jonasbase;
import java.util.StringTokenizer;
import org.ow2.jonas.ant.JOnASBaseTask;
/**
* Allow to configure the JMS service.
* @author Florent Benoit
*/
public class Jms extends Tasks {
/**
* Info for the logger.
*/
private static final String INFO = "[JMS] ";
/**
* Default host name.
*/
private static final String DEFAULT_HOST = "localhost";
/**
* Default port number.
*/
private static final String DEFAULT_PORT = "16010";
/**
* Token for the end of the joramAdmin configuration file.
*/
private static final String TOKEN_END_CONF_FILE = "";
/**
* Default constructor.
*/
public Jms() {
super();
}
/**
* Set the host name for Joram.
* @param host host name for Joram
*/
public void setHost(final String host) {
// For JMS
JReplace propertyReplace = new JReplace();
propertyReplace.setConfigurationFile(JOnASBaseTask.JORAM_CONF_FILE);
propertyReplace.setToken(DEFAULT_HOST);
propertyReplace.setValue(host);
propertyReplace.setLogInfo(INFO + "Setting Joram host name to : " + host + " in "
+ JOnASBaseTask.JORAM_CONF_FILE + " file.");
addTask(propertyReplace);
// for RAR file
propertyReplace = new JReplace();
propertyReplace.setConfigurationFile(JOnASBaseTask.JORAM_ADMIN_CONF_FILE);
propertyReplace.setToken(DEFAULT_HOST);
propertyReplace.setValue(host);
propertyReplace.setLogInfo(INFO + "Setting Joram host name to : " + host + " in "
+ JOnASBaseTask.JORAM_ADMIN_CONF_FILE + " file.");
addTask(propertyReplace);
// Patch the RAR file
JmsRa jmsRa = new JmsRa();
jmsRa.setServerHost(host);
addTask(jmsRa);
}
/**
* Set the port number for Joram.
* @param portNumber the port for Joram
*/
public void setPort(final String portNumber) {
// For JMS
JReplace propertyReplace = new JReplace();
propertyReplace.setConfigurationFile(JOnASBaseTask.JORAM_CONF_FILE);
propertyReplace.setToken(DEFAULT_PORT);
propertyReplace.setValue(portNumber);
propertyReplace.setLogInfo(INFO + "Setting Joram port number to : " + portNumber + " in "
+ JOnASBaseTask.JORAM_CONF_FILE + " file.");
addTask(propertyReplace);
// for RAR file
propertyReplace = new JReplace();
propertyReplace.setConfigurationFile(JOnASBaseTask.JORAM_ADMIN_CONF_FILE);
propertyReplace.setToken(DEFAULT_PORT);
propertyReplace.setValue(portNumber);
propertyReplace.setLogInfo(INFO + "Setting Joram port number to : " + portNumber + " in "
+ JOnASBaseTask.JORAM_ADMIN_CONF_FILE + " file.");
addTask(propertyReplace);
// Patch the RAR file
JmsRa jmsRa = new JmsRa();
jmsRa.setServerPort(portNumber);
addTask(jmsRa);
}
/**
* Set the initial topics when JOnAS start.
* @param initialTopics comma separated list of topics
*/
public void setInitialTopics(final String initialTopics) {
JReplace propertyReplace = new JReplace();
propertyReplace.setConfigurationFile(JOnASBaseTask.JORAM_ADMIN_CONF_FILE);
propertyReplace.setToken(TOKEN_END_CONF_FILE);
String tokenValue = "";
StringTokenizer st = new StringTokenizer(initialTopics, ",");
while (st.hasMoreTokens()) {
String topic = st.nextToken();
tokenValue += " "
+ "\n"
+ " "
+ "\n"
+ " "
+ "\n"
+ " "
+ "\n"
+ " "
+ "\n";
}
tokenValue += TOKEN_END_CONF_FILE;
propertyReplace.setValue(tokenValue);
propertyReplace.setLogInfo(INFO + "Setting initial topics to : " + initialTopics);
addTask(propertyReplace);
}
/**
* Set the initial queues when JOnAS start.
* @param initialQueues comma separated list of topics
*/
public void setInitialQueues(final String initialQueues) {
JReplace propertyReplace = new JReplace();
propertyReplace.setConfigurationFile(JOnASBaseTask.JORAM_ADMIN_CONF_FILE);
propertyReplace.setToken(TOKEN_END_CONF_FILE);
String tokenValue = "";
StringTokenizer st = new StringTokenizer(initialQueues, ",");
while (st.hasMoreTokens()) {
String queue = st.nextToken();
tokenValue += " "
+ "\n"
+ " "
+ "\n"
+ " "
+ "\n"
+ " "
+ "\n"
+ " "
+ "\n";
}
tokenValue += TOKEN_END_CONF_FILE;
propertyReplace.setValue(tokenValue);
propertyReplace.setLogInfo(INFO + "Setting initial queues to : " + initialQueues);
addTask(propertyReplace);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy