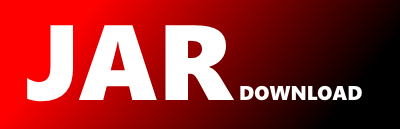
org.ow2.jonas.ant.jonasbase.PropertyTask Maven / Gradle / Ivy
/**
* JOnAS: Java(TM) Open Application Server
* Copyright (C) 2009 Bull S.A.S.
* Contact: [email protected]
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307
* USA
*
* --------------------------------------------------------------------------
* $Id: PropertyTask.java 19283 2010-02-24 16:16:26Z benoitf $
* --------------------------------------------------------------------------
*/
package org.ow2.jonas.ant.jonasbase;
import java.io.File;
import java.util.ArrayList;
import java.util.Hashtable;
import java.util.Iterator;
import java.util.List;
import java.util.Map.Entry;
import org.apache.tools.ant.BuildException;
import org.ow2.jonas.ant.PropertyStatement;
/**
* Task that replaces or adds any property in a file located in JONAS_BASE/conf
.
* @author eyindanga
*
*/
public class PropertyTask extends JTask implements BaseTaskItf {
/**
* Info for the logger.
*/
private static final String INFO = "[PropertyTask] ";
/**
* Properties to be replaced or added for each file.
*/
private Hashtable> properties4file = new Hashtable>();
/**
* Default constructor.
*/
public PropertyTask() {
}
/**
* Add a property statement.
* @param fileName The file where the property is located.
* @param propertyName name of the property
* @param propertyValue value of the property
* @param add if true then, add it, else replace it.
*/
public void addPropertyStatement(final String fileName, final String propertyName,
final String propertyValue, final boolean add) {
addPropertyStatement(fileName, new PropertyStatement(propertyName, propertyValue, add));
}
/**
* Add a property statement.
* @param fileName The file where the property is located.
* @param statement the property statement to add or replace.
*/
public void addPropertyStatement(final String fileName, final PropertyStatement statement) {
List statements = properties4file.get(fileName);
if (statements == null) {
statements = new ArrayList();
properties4file.put(fileName, statements);
}
statements.add(statement);
}
/**
* Add properties statements.
* @param fileName The file where the properties are located.
* @param vstatement the properties statements to add or replace.
*/
public void addPropertyStatements(final String fileName, final List vstatements) {
List statements = properties4file.get(fileName);
if (statements == null) {
statements = new ArrayList();
properties4file.put(fileName, statements);
}
statements.addAll(vstatements);
}
/**
* Executes this task.
*/
@Override
public void execute() throws BuildException {
super.execute();
String jBaseConf = getDestDir().getPath() + File.separator + "conf";
for (Iterator>> iterator = properties4file.entrySet().iterator(); iterator.hasNext();) {
Entry> entry = iterator.next();
String fileName = entry.getKey();
log(INFO + ": Replace statements " + entry.getValue() + " in '" + getDestDir().getPath()
+ File.separator + fileName + "'");
for (Iterator iterator2 = entry.getValue().iterator(); iterator2.hasNext();) {
PropertyStatement statement = iterator2.next();
changeValueForKey(INFO, jBaseConf, fileName, statement.getName(),
statement.getValue(), statement.isAdd());
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy