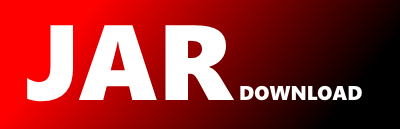
org.ow2.jonas.ant.jonasbase.wsdl.WsdlPublish Maven / Gradle / Ivy
/**
* JOnAS: Java(TM) Open Application Server
* Copyright (C) 2004-2009 Bull S.A.S.
* Contact: [email protected]
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307
* USA
*
* --------------------------------------------------------------------------
* $Id: WsdlPublish.java 16369 2009-02-03 21:47:51Z alitokmen $
* --------------------------------------------------------------------------
*/
package org.ow2.jonas.ant.jonasbase.wsdl;
import org.ow2.jonas.ant.JOnASBaseTask;
import org.ow2.jonas.ant.jonasbase.BaseTaskItf;
import org.ow2.jonas.ant.jonasbase.JReplace;
import org.ow2.jonas.ant.jonasbase.JTask;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import java.util.Properties;
/**
* Generates files for WSDL publish.
* @author Florent Benoit
*/
public class WsdlPublish extends JTask implements BaseTaskItf {
/**
* Info for the logger.
*/
private static final String INFO = "[WSDL-Publish] ";
/**
* Property for WSDL Publishers.
*/
private static final String WSDL_PUBLISHER_PROPERTY = "jonas.service.wsdl-publisher.publishers";
/**
* Default property for WSDL publishers.
*/
private static final String TOKEN_WSDLPUBLISHER = WSDL_PUBLISHER_PROPERTY + SEPARATORS + "file1";
/**
* List of files (WSDL publish).
*/
private List files = new ArrayList();
/**
* List of uddi (WSDL publish).
*/
private List uddis = new ArrayList();
/**
* Add file (wsdl publish).
* @param file properties file
*/
public void addConfiguredFile(final File file) {
files.add(file);
}
/**
* Add UDDI (wsdl publish).
* @param uddi properties file
*/
public void addConfiguredUddi(final Uddi uddi) {
uddis.add(uddi);
}
/**
* set files (wsdl publish).
* @param files list of properties file
*/
public void setFiles(final List files) {
this.files = files;
}
/**
* set uddis (wsdl publish).
* @param uddis list of properties uddi file
*/
public void setUddis(final List uddis) {
this.uddis = uddis;
}
/**
* Execute this task.
*/
public void execute() {
String fileNameList = "";
java.io.File jonasBaseConfDir = new java.io.File(getDestDir().getPath() + java.io.File.separator + "conf");
// Write file Publisher
for (Iterator it = files.iterator(); it.hasNext();) {
File f = it.next();
String dir = f.getDir();
String encoding = f.getEncoding();
String name = f.getName();
// Add file to handler list
if (fileNameList.length() == 0) {
fileNameList = name;
} else {
fileNameList += "," + name;
}
String fileName = name + ".properties";
// Build properties file and write it
Properties props = new Properties();
props.put("jonas.service.wsdl.class", File.PUBLISHER_CLASS);
props.put("jonas.service.publish.file.directory", dir);
props.put("jonas.service.publish.file.encoding", encoding);
java.io.File writeFile = new java.io.File(jonasBaseConfDir, fileName);
log(INFO + "Generating a WSDL publish file with name '" + name + "', dir '" + dir + "' and encoding '"
+ encoding + "' in file '" + writeFile + "'...");
writePropsToFile(INFO, props, writeFile);
}
// Write Uddi Publisher
for (Iterator it = uddis.iterator(); it.hasNext();) {
Uddi uddi = it.next();
String name = uddi.getName();
String username = uddi.getUserName();
String password = uddi.getPassword();
String orgName = uddi.getOrgName();
String orgDesc = uddi.getOrgDesc();
String orgPersonName = uddi.getOrgPersonName();
String lifecyclemanagerURL = uddi.getLifecyclemanagerURL();
String queryManagerURL = uddi.getQueryManagerURL();
// Add file to handler list
if (fileNameList.length() == 0) {
fileNameList = name;
} else {
fileNameList += "," + name;
}
String fileName = name + ".properties";
// Build properties file and write it
Properties props = new Properties();
props.put("jonas.service.wsdl.class", Uddi.PUBLISHER_CLASS);
props.put("jonas.service.publish.uddi.username", username);
props.put("jonas.service.publish.uddi.password", password);
props.put("jonas.service.publish.uddi.organization.name", orgName);
props.put("jonas.service.publish.uddi.organization.desc", orgDesc);
props.put("jonas.service.publish.uddi.organization.person_name", orgPersonName);
props.put("javax.xml.registry.lifeCycleManagerURL", lifecyclemanagerURL);
props.put("javax.xml.registry.queryManagerURL", queryManagerURL);
java.io.File writeFile = new java.io.File(jonasBaseConfDir, fileName);
log(INFO + "Generating a WSDL publish UDDI with name '" + name + "' in file '" + writeFile + "'...");
writePropsToFile(INFO, props, writeFile);
}
// Now set the handlers to the existing list for the property
JReplace propertyReplace = new JReplace();
propertyReplace.setProject(getProject());
propertyReplace.setConfigurationFile(JOnASBaseTask.JONAS_CONF_FILE);
propertyReplace.setDestDir(new java.io.File(getDestDir().getPath()));
propertyReplace.setToken(TOKEN_WSDLPUBLISHER);
propertyReplace.setValue(WSDL_PUBLISHER_PROPERTY + SEPARATORS + fileNameList);
log(INFO + "Adding WSDL Publishers '" + fileNameList + "' in " + JOnASBaseTask.JONAS_CONF_FILE + " file.");
propertyReplace.execute();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy