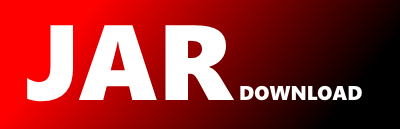
org.ow2.jonas.datasource.deployer.impl.DatasourceDeployer Maven / Gradle / Ivy
/**
* JOnAS: Java(TM) Open Application Server
* Copyright (C) 2010 Bull S.A.S.
* Contact: [email protected]
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA
*
* --------------------------------------------------------------------------
* $Id: DatasourceDeployer.java 20933 2011-02-23 10:46:30Z pelletib $
* --------------------------------------------------------------------------
*/
package org.ow2.jonas.datasource.deployer.impl;
import java.io.File;
import java.io.IOException;
import java.net.URL;
import java.util.HashMap;
import java.util.Map;
import java.util.Properties;
import org.ow2.jonas.datasource.binding.DatasourceType;
import org.ow2.jonas.datasource.binding.Datasources;
import org.ow2.jonas.datasource.deploy.deployable.api.DatasourceDeployable;
import org.ow2.jonas.datasource.deployer.api.IDatasourceDeployer;
import org.ow2.jonas.datasource.reader.DatasourceXmlReader;
import org.ow2.jonas.generators.raconfig.RAConfig;
import org.ow2.jonas.generators.raconfig.RAConfigException;
import org.ow2.jonas.lib.work.DeployerLog;
import org.ow2.jonas.properties.ServerProperties;
import org.ow2.util.archive.api.ArchiveException;
import org.ow2.util.archive.api.IArchive;
import org.ow2.util.archive.impl.ArchiveManager;
import org.ow2.util.ee.deploy.api.deployable.IDeployable;
import org.ow2.util.ee.deploy.api.deployer.DeployerException;
import org.ow2.util.ee.deploy.api.deployer.IDeployerManager;
import org.ow2.util.ee.deploy.api.deployer.UnsupportedDeployerException;
import org.ow2.util.ee.deploy.impl.helper.DeployableHelper;
import org.ow2.util.ee.deploy.impl.helper.DeployableHelperException;
import org.ow2.util.log.Log;
import org.ow2.util.log.LogFactory;
import org.ow2.util.url.URLUtils;
/**
* This deployer will deploy Datasource module. Generating .RAR files and deploy
* them.
* @author Alexis RENOUX
*/
public class DatasourceDeployer implements IDatasourceDeployer {
/**
* Logger.
*/
private Log logger = LogFactory.getLog(DatasourceDeployer.class);
/**
* The Deployer Manager that will be used to deploy RAR files.
*/
private IDeployerManager deployerManager = null;
/**
* The datasourceReader that will be used to read the datasource XML file.
*/
private DatasourceXmlReader datasourceReader;
/**
* The default RAR creation path.
*/
private static final String DEFAULT_ARCHIVE_PATH = "deployer" + File.separator +"datasources";
/**
* Working directory
*/
protected File archiveFile = null;
/**
* The datasources that were deployed, and the RAR path.
*/
private Map datasourceDeployables = new HashMap();
/**
* Server Properties.
*/
protected ServerProperties serverProps;
/**
* Reference to the {@link DeployerLog} which is the class that manage the
* accesses to the log file (to remove the jar).
*/
protected DeployerLog deployerLog = null;
/**
* Internal JDBC RAR used to build datasource RAR
*/
private String dmRar;
/**
* Creates a new DatasourceDeployer.
*/
public DatasourceDeployer() throws IOException {
}
/**
* Resource service used by this deployer.
*/
// private ResourceService resourceService = null;
/**
* Undeploy the given Datasource. (Not implemented yet)
* @param datasourceDeployable the deployable to remove.
* @throws DeployerException if the Datasource is not undeployed.
*/
protected void undeployDatasource(final DatasourceDeployable datasourceDeployable) throws DeployerException {
logger.info("Undeploying {0}", datasourceDeployable);
// get the RAR related to
File rarFile = new File(getRarFilePathForDeployable(datasourceDeployable));
IArchive archiveRar = ArchiveManager.getInstance().getArchive(rarFile);
if (archiveRar == null) {
throw new DeployerException("Null archive for file " + rarFile);
}
// Create a Deployable
IDeployable> rardeployable = null;
try {
rardeployable = DeployableHelper.getDeployable(archiveRar);
} catch (DeployableHelperException e) {
throw new DeployerException(e);
}
if (rardeployable == null) {
throw new DeployerException("Null deployable for archive " + archiveRar);
}
// Undeploy the Datasource file
try {
this.deployerManager.undeploy(rardeployable);
} catch (DeployerException e) {
throw new DeployerException("Exception while undeploying deployable " + rardeployable, e);
} catch (UnsupportedDeployerException e) {
throw new DeployerException("No deployer found for deployable " + rardeployable, e);
}
// Remove the entry deployment was succesfull.
this.datasourceDeployables.remove(datasourceDeployable);
}
/**
* Deploy the given Datasource.
* @param deployable the deployable to add.
* @throws DeployerException if the Datasource is not deployed.
*/
protected void deployDatasource(final IDeployable> deployable) throws DeployerException {
logger.debug("Request to deploy {0} received", deployable);
if (deployable == null) {
throw new DeployerException("Null deployable");
}
if (!(DatasourceDeployable.class.isAssignableFrom(deployable.getClass()))) {
throw new DeployerException("Bad deployable type " + deployable.getClass());
}
logger.info("Deploying datasource {0}", deployable);
DatasourceDeployable datasourceDeployable = DatasourceDeployable.class.cast(deployable);
datasourceReader = new DatasourceXmlReader();
if (datasourceReader == null) {
throw new DeployerException("Deployment aborted - The DatasourceReader is null");
}
File xmlfile = this.getFile(datasourceDeployable);
Datasources datasources = null;
// Create java objects from XML file
try {
datasources = datasourceReader.extractDataSources(xmlfile);
} catch (Exception e) {
throw new DeployerException("Error while parsing file " + xmlfile + " - Deployment aborted", e);
}
if (datasources == null) {
throw new DeployerException("The deployable " + deployable + " resolved in null datasource - Deployment aborted");
}
datasourceDeployable.setAttachedData(datasources);
for (DatasourceType ds : datasources.getDatasources()) {
DatasourceType dsInitialized = datasourceReader.initDataSource(ds);
// Get the Properties java object from datasourceType
Properties properties = datasourceReader.getProperties(dsInitialized);
String fileIn = null;
String fileOut = null;
String fileName = "";
try {
fileName = "ds-" + dsInitialized.getDatasourceConfiguration().getName().replace('/', '_') + "-";
fileOut = File.createTempFile(fileName, ".rar", archiveFile).getAbsolutePath();
} catch (IOException e1) {
String msg = "Error while creating temp file <" + fileName + ".rar> in directory <" + archiveFile.getPath() + ">";
throw new DeployerException(msg, e1);
}
// Generate .rar (only DM are supported)
fileIn = dmRar;
logger.info("Generate RAR {0} -> {1}", fileIn, fileOut);
try {
RAConfig.generateRars(properties, "JOnASJDBC_DM", fileIn, fileOut);
} catch (RAConfigException e) {
logger.error("Failure to deploy datasource {0}", ds);
throw new DeployerException(e);
}
/*
* Deploy .rar 1- Create IArchive 2- Create Deployable 3- Deploy
*/
// Create an IArchive
File generatedFile = new File(fileOut);
if (!generatedFile.exists()) {
logger.error("RAR was not generated with RAConfig- {0}", fileOut);
throw new DeployerException("RAR was not generated <" + fileOut + ">");
}
IArchive archiveRar = ArchiveManager.getInstance().getArchive(generatedFile);
if (archiveRar == null) {
throw new DeployerException("Null archive for file " + fileOut);
}
// Create a Deployable
IDeployable> rardeployable = null;
try {
rardeployable = DeployableHelper.getDeployable(archiveRar);
} catch (DeployableHelperException e) {
throw new DeployerException(e);
}
if (rardeployable == null) {
throw new DeployerException("Null deployable for archive " + archiveRar);
}
// Deploy
try {
this.deployerManager.deploy(rardeployable);
} catch (DeployerException e) {
throw new DeployerException("Exception while deploying deployable " + rardeployable, e);
} catch (UnsupportedDeployerException e) {
throw new DeployerException("No deployer found for deployable " + rardeployable, e);
}
// Here deployment was succesfull.
this.datasourceDeployables.put(datasourceDeployable, fileOut);
try {
// The file is unpacked, so log it
if (deployerLog != null) {
File unpackedFile = URLUtils.urlToFile(datasourceDeployable.getArchive().getURL());
deployerLog.addEntry(xmlfile, new File(fileOut));
}
} catch (Exception e) {
throw new DeployerException("Cannot get the url of the initial deployable for the datasource Module '" + deployable
+ "'.", e);
}
}
}
/**
* Allows to get a File from the given DataSource Deployable.
* @param datasourceDeployable the given DataSource deployable.
* @return a File object of this deployable
* @throws DeployerException if the File can't be obtained.
*/
protected File getFile(final DatasourceDeployable datasourceDeployable) throws DeployerException {
// Get URL
URL datasourceURL = null;
try {
datasourceURL = datasourceDeployable.getArchive().getURL();
} catch (ArchiveException e) {
throw new DeployerException("Cannot get URL from Datasource deployable '" + datasourceDeployable + "'.", e);
}
// Get File
return URLUtils.urlToFile(datasourceURL);
}
/**
* {@inheritDoc}
*/
public void deploy(final IDeployable> deployable) throws DeployerException {
check(deployable);
// Deploy the Datasource Deployable
if (DatasourceDeployable.class.isAssignableFrom(deployable.getClass())) {
deployDatasource(deployable);
}
}
/**
* {@inheritDoc}
*/
public boolean isDeployed(final IDeployable> deployable) throws DeployerException {
if (this.supports(deployable)) {
return (this.datasourceDeployables.get(deployable) != null);
} else {
return false;
}
}
/**
* check if the RAR is currently deployed
*/
public boolean isDeployed(final String rarFileName) {
return datasourceDeployables.containsValue(rarFileName);
}
/**
* {@inheritDoc}
*/
public boolean supports(final IDeployable> deployable) {
return (DatasourceDeployable.class.isAssignableFrom(deployable.getClass()));
}
/**
* {@inheritDoc}
*/
public void undeploy(final IDeployable> deployable) throws DeployerException {
if (!this.supports(deployable)) {
throw new DeployerException("Cannot undeploy non-datasource deployable " + deployable + ".");
}
if (!this.isDeployed(deployable)) {
throw new DeployerException("Cannot undeploy datasource deployable " + deployable + " - it is not deployed.");
}
undeployDatasource(DatasourceDeployable.class.cast(deployable));
}
/**
* Check if the given deployable is deployable or not.
* @param deployable the deployable to check.
* @throws DeployerException if the deployable is not supported.
*/
private void check(final IDeployable> deployable) throws DeployerException {
if (!supports(deployable)) {
throw new DeployerException("The deployment of the deployable'" + deployable
+ "' is not supported by this deployer.");
}
}
public String getRarFilePathForDeployable(final DatasourceDeployable deployable) {
return this.datasourceDeployables.get(deployable);
}
/**
* {@inheritDoc}
*/
public IDeployerManager getDeployerManager() {
return deployerManager;
}
/**
* {@inheritDoc}
*/
public void setDeployerManager(final IDeployerManager deployerManager) {
this.deployerManager = deployerManager;
}
/**
* Set the Server Properties.
* @param props {@link ServerProperties} instance
*/
public void setServerProperties(final ServerProperties props) {
this.serverProps = props;
}
/**
* Create working directory for the deployer.
*/
protected void initWorkingDirectory() {
if (archiveFile == null) {
archiveFile = new File(serverProps.getWorkDirectory() + File.separator + DEFAULT_ARCHIVE_PATH + File.separator + serverProps.getServerName());
archiveFile.mkdirs();
}
}
/**
* @return Get path to the DM rar
*/
public String getDmRar() {
return dmRar;
}
/**
* set the DM RAR path
* @param dmRar path to rar file
*/
public void setDmRar(final String dmRar) {
this.dmRar = dmRar;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy