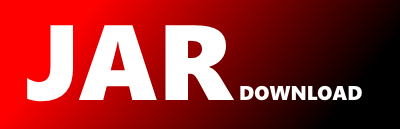
org.ow2.jonas.lib.management.javaee.J2EEManagedObject Maven / Gradle / Ivy
/**
* JOnAS: Java(TM) Open Application Server
* Copyright (C) 2007-2008 Bull S.A.S.
* Contact: [email protected]
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA
*
* --------------------------------------------------------------------------
* $Id: J2EEManagedObject.java 16706 2009-03-03 15:56:26Z danesa $
* --------------------------------------------------------------------------
*/
package org.ow2.jonas.lib.management.javaee;
/**
* @author Adriana Danes
*/
public class J2EEManagedObject extends ManagedObject {
// ------------------------------------------------------------- Private
// Constants
// the following strings represent key properties within
// a J2EEManagedObject's objectName cf. JSR 77
/**
* Name constant.
*/
public static final String NAME = "name";
/**
* Constant for j2ee server.
*/
public static final String J2EE_TYPE_SERVER = "J2EEServer";
/**
* Constant for a j2ee application.
*/
public static final String J2EE_TYPE_APPLICATION = "J2EEApplication";
// ------------------------------------------------------------- Properties
/**
* The managed object name.
*/
private String objectName;
/**
* State management support for this managed object (start, stop, ...).
*/
private boolean stateManageable;
/**
* Performance statistics support for this managed object.
*/
private boolean statisticsProvider;
/**
* Event provider support for this managed object.
*/
private boolean eventProvider;
/**
* MBean constructor.
*/
protected J2EEManagedObject() {
this.stateManageable = false;
this.statisticsProvider = false;
this.eventProvider = false;
}
/**
* MBean constructor.
* @param objectName The complete name of the managed object
*/
protected J2EEManagedObject(final String objectName) {
this();
this.objectName = objectName;
}
/**
* MBean constructor.
* @param stateManageable if true, this managed object implements J2EE State
* Management Model
* @param statisticsProvider if true, this managed object implements the
* J2EE StatisticProvide Model
* @param eventProvider if true, this managed object implements the J2EE
* EventProvider Model
*/
protected J2EEManagedObject(final boolean stateManageable, final boolean statisticsProvider, final boolean eventProvider) {
this.stateManageable = stateManageable;
this.statisticsProvider = statisticsProvider;
this.eventProvider = eventProvider;
}
/**
* MBean constructor.
* @param objectName object name of the managed object
* @param stateManageable if true, this managed object implements J2EE State
* Management Model
* @param statisticsProvider if true, this managed object implements the
* J2EE StatisticProvide Model
* @param eventProvider if true, this managed object implements the J2EE
* EventProvider Model
*/
protected J2EEManagedObject(final String objectName, final boolean stateManageable, final boolean statisticsProvider,
final boolean eventProvider) {
this.objectName = objectName;
this.stateManageable = stateManageable;
this.statisticsProvider = statisticsProvider;
this.eventProvider = eventProvider;
}
/**
* Return this MBean's name.
* @return The name of the MBean (see OBJECT_NAME in the JSR77)
*/
public String getObjectName() {
return objectName;
}
/**
* Set the MBean's name.
* @param objectName The objectName to set
*/
public void setObjectName(final String objectName) {
this.objectName = objectName;
}
public void setStateManageable(final boolean stateManageable) {
this.stateManageable = stateManageable;
}
public void setStatisticsProvider(final boolean statisticsProvider) {
this.statisticsProvider = statisticsProvider;
}
public void setEventProvider(final boolean eventProvider) {
this.eventProvider = eventProvider;
}
/**
* @return true if it is an event provider
*/
public boolean isEventProvider() {
return eventProvider;
}
/**
* @return true if this managed object implements J2EE State Management
* Model
*/
public boolean isStateManageable() {
return stateManageable;
}
/**
* @return true if this managed object implements the J2EE StatisticProvider
* Model
*/
public boolean isStatisticsProvider() {
return statisticsProvider;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy