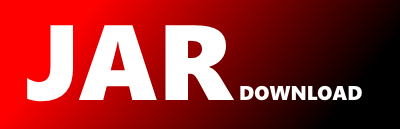
org.ow2.jonas.lib.management.javaee.JSR77ManagementIdentifier Maven / Gradle / Ivy
/**
* JOnAS: Java(TM) Open Application Server
* Copyright (C) 2007 Bull S.A.S.
* Contact: [email protected]
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA
*
* --------------------------------------------------------------------------
* $Id: JSR77ManagementIdentifier.java 15428 2008-10-07 11:20:29Z sauthieg $
* --------------------------------------------------------------------------
*/
package org.ow2.jonas.lib.management.javaee;
import java.util.Hashtable;
import java.util.Iterator;
import javax.management.MalformedObjectNameException;
import javax.management.ObjectName;
import org.ow2.jonas.jmx.JManagementIdentifier;
import org.ow2.util.log.Log;
import org.ow2.util.log.LogFactory;
/**
* Specialized {@link JManagementIdentifier} for JSR77 MBeans.
* @author Guillaume Sauthier
* @author Florent BENOIT
* @param Managed Type
*/
public abstract class JSR77ManagementIdentifier implements JManagementIdentifier {
/**
* Default domain name.
*/
private static final String DEFAULT_DOMAIN_NAME = "";
/**
* Logger.
*/
private static Log logger = LogFactory.getLog(JSR77ManagementIdentifier.class);
/**
* Domain name.
*/
private String domainName = null;
/**
* Server name.
*/
private String serverName = null;
/**
* Empty default constructor.
*/
protected JSR77ManagementIdentifier() {
}
/**
* @param name base ObjectName
* @return Returns a String that contains "inherited" properties from
* parent's ObjectName
*/
@SuppressWarnings("unchecked")
protected static String getInheritedPropertiesAsString(final ObjectName name) {
Hashtable table = (Hashtable) name.getKeyPropertyList().clone();
// we remove some attributes from the ObjectName
table.remove("j2eeType");
table.remove("type");
table.remove("subtype");
table.remove("name");
StringBuffer sb = new StringBuffer();
Iterator it = table.keySet().iterator();
while (it.hasNext()) {
String key = it.next();
sb.append(key + "=" + table.get(key) + ",");
}
if (sb.length() > 1) {
// remove the trailing comma
sb.setLength(sb.length() - 1);
}
return sb.toString();
}
/**
* @param parentObjectName Parent ObjectName.
* @return Returns the couple j2eetype=name of the parent ObjectName.
*/
protected static String getParentNameProperty(final String parentObjectName) {
ObjectName on = null;
try {
on = ObjectName.getInstance(parentObjectName);
} catch (MalformedObjectNameException e) {
logger.error("Cannot get objectname on {0}", parentObjectName, e);
return "";
} catch (NullPointerException e) {
logger.error("Cannot get objectname on {0}", parentObjectName, e);
return "";
}
String type = on.getKeyProperty("j2eeType");
String name = on.getKeyProperty("name");
return type + "=" + name;
}
/**
* @return Returns the JMX Domain name of the MBean.
*/
public String getDomain() {
if (domainName == null) {
return DEFAULT_DOMAIN_NAME;
}
return domainName;
}
/**
* Sets the domain for this identifier.
* @param domainName the JMX Domain name of the MBean.
*/
public void setDomain(final String domainName) {
this.domainName = domainName;
}
/**
* @return the JMX Server name of the MBean.
*/
public String getServerName() {
return serverName;
}
/**
* Sets the Server name for this identifier.
* @param serverName the JMX Server name of this MBean.
*/
public void setServerName(final String serverName) {
this.serverName = serverName;
}
/**
* {@inheritDoc}
*/
public String getTypeName() {
return "j2eeType";
}
/**
* {@inheritDoc}
*/
public String getTypeProperty() {
// TODO Move in an AbstractManagementIdentifier
return getTypeName() + "=" + getTypeValue();
}
/**
* @return the logger
*/
public static final Log getLogger() {
return logger;
}
/**
* @return the String for J2EEServer.
*/
protected String getJ2EEServerString() {
return "J2EEServer=" + serverName;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy