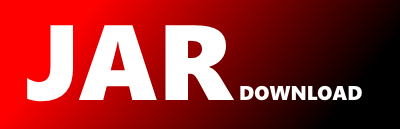
org.ow2.jonas.web.base.proxy.HTTPResponse Maven / Gradle / Ivy
/**
* JOnAS: Java(TM) Open Application Server
* Copyright (C) 2009 Bull S.A.S.
* Contact: [email protected]
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307
* USA
*
* --------------------------------------------------------------------------
* $Id: HTTPResponse.java 19283 2010-02-24 16:16:26Z benoitf $
* --------------------------------------------------------------------------
*/
package org.ow2.jonas.web.base.proxy;
import java.io.ByteArrayOutputStream;
import java.io.PrintStream;
import java.io.IOException;
import java.util.Date;
import org.ow2.util.log.Log;
import org.ow2.util.log.LogFactory;
/**
* Allows to define some HTTP response
* @author Florent Benoit
*/
public class HTTPResponse {
/**
* Logger.
*/
private static Log logger = LogFactory.getLog(HTTPResponse.class);
/**
* Default status code.
*/
private static final String DEFAULT_STATUS_CODE = "HTTP/1.0 200 OK";
/**
* Carriage return.
*/
final static String CRLF = "\r\n";
/**
* Body of the http answer.
*/
private StringBuilder body = null;
/**
* HTML Title.
*/
private String title = "Http/OnDemandProxy Service";
/**
* Status code (200, 404, ...).
*/
private String statusCode = DEFAULT_STATUS_CODE;
/**
* Default is text/html.
*/
private String contentType = "text/html; charset=ISO-8859-1";
/**
* If body should be an array of bytes.
*/
private byte[] bodyBytes = null;
/**
* Needs to send a refresh javascript call ?
*/
private boolean refresh = false;
/**
* Build a new object with the default status code.
*/
public HTTPResponse() {
this(DEFAULT_STATUS_CODE);
}
/**
* Build an answer with the given status code.
* @param statusCode given code
*/
public HTTPResponse(String statusCode) {
this.statusCode = statusCode;
this.body = new StringBuilder();
}
/**
* Sets the title.
* @param title given HTML title
*/
public void setTitle(String title) {
this.title = title;
}
/**
* Append text into the body.
* @param string the given string object
*/
public void println(String string) {
body.append(string);
body.append("\n");
}
/**
* Append text into the body.
* @param string the given string object
*/
public void print(String string) {
body.append(string);
}
/**
* Refresh mode is enabled or disabled.
* @param refresh if true, send in the answer a refresh code
*/
public void setRefresh(boolean refresh) {
this.refresh = refresh;
}
/**
* Gets the array of bytes of this answer.
* @return the bytes of the answer
*/
public byte[] getContent() {
// Body
StringBuilder bodyContent = new StringBuilder();
bodyContent.append("");
if (refresh) {
bodyContent.append("");
}
bodyContent.append("");
bodyContent.append(title);
bodyContent.append(" ");
bodyContent.append("");
bodyContent.append("");
bodyContent.append(body.toString());
bodyContent.append(" ");
bodyContent.append("
");
bodyContent.append("");
// Header
StringBuilder headerContent = new StringBuilder();
headerContent.append(statusCode);
headerContent.append(CRLF);
headerContent.append("Content-Type: ");
headerContent.append(contentType);
headerContent.append(CRLF);
headerContent.append("Date: ");
headerContent.append(new Date());
headerContent.append(CRLF);
headerContent.append("Cache-Control: no-cache");
headerContent.append(CRLF);
headerContent.append("Server: JOnAS/HttpOnDemand Proxy");
headerContent.append(CRLF);
headerContent.append("Content-Length: ");
if (bodyBytes != null) {
headerContent.append(bodyBytes.length);
} else {
headerContent.append(bodyContent.toString().getBytes().length);
}
headerContent.append(CRLF);
// Write message (header + body)
ByteArrayOutputStream byteArrayOutputStream = new ByteArrayOutputStream();
PrintStream printStream = new PrintStream(byteArrayOutputStream);
if (bodyBytes != null) {
try {
byteArrayOutputStream.write(headerContent.toString().getBytes());
byteArrayOutputStream.write(CRLF.getBytes());
byteArrayOutputStream.write(bodyBytes);
} catch (IOException e) {
logger.error("Unable to write bytes", e);
}
} else {
// Header
printStream.print(headerContent);
// empty line between header and body
printStream.print(CRLF);
// then body
printStream.print(bodyContent);
}
// Get bytes of the stream
byte[] messageBytes = byteArrayOutputStream.toByteArray();
try {
byteArrayOutputStream.close();
} catch (IOException e) {
throw new IllegalStateException("Unable to get bytes", e);
}
// send it back
return messageBytes;
}
public void setContentType(String contentType) {
this.contentType = contentType;
}
public void setBodyBytes(byte[] bodyBytes) {
this.bodyBytes = bodyBytes;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy