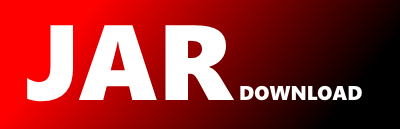
org.ow2.mind.io.BasicOutputFileLocator Maven / Gradle / Ivy
/**
* Copyright (C) 2009 STMicroelectronics
*
* This file is part of "Mind Compiler" is free software: you can redistribute
* it and/or modify it under the terms of the GNU Lesser General Public License
* as published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS
* FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License for more
* details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program. If not, see .
*
* Contact: [email protected]
*
* Authors: Matthieu Leclercq
* Contributors:
*/
package org.ow2.mind.io;
import static org.ow2.mind.PathHelper.isRelative;
import java.io.File;
import java.io.IOException;
import java.util.Map;
import org.objectweb.fractal.adl.ADLException;
import org.objectweb.fractal.adl.CompilerError;
import org.objectweb.fractal.adl.error.GenericErrors;
import org.ow2.mind.ForceRegenContextHelper;
public class BasicOutputFileLocator implements OutputFileLocator {
public static final String OUTPUT_DIR_CONTEXT_KEY = "outputdir";
public static final String DEFAULT_OUTPUT_DIR = "build";
public static final String TEMPORARY_OUTPUT_DIR_CONTEXT_KEY = "temporaryOutputDir";
public File getCSourceOutputFile(final String path,
final Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy