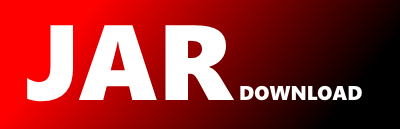
org.ow2.mind.compilation.CompilerContextHelper Maven / Gradle / Ivy
/**
* Copyright (C) 2009 STMicroelectronics
*
* This file is part of "Mind Compiler" is free software: you can redistribute
* it and/or modify it under the terms of the GNU Lesser General Public License
* as published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS
* FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License for more
* details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program. If not, see .
*
* Contact: [email protected]
*
* Authors: Matthieu Leclercq
* Contributors: Julien Tous
*/
package org.ow2.mind.compilation;
import java.util.Collections;
import java.util.List;
import java.util.Map;
public final class CompilerContextHelper {
public static final String LINKER_COMMAND_CONTEXT_KEY = "linker-command";
public static final String ASSEMBLER_COMMAND_CONTEXT_KEY = "assembler-command";
public static final String COMPILER_COMMAND_CONTEXT_KEY = "compiler-command";
public static final String DEFAULT_COMPILER_COMMAND = "gcc";
public static final String DEFAULT_ASSEMBLER_COMMAND = "gcc";
public static final String DEFAULT_LINKER_COMMAND = "gcc";
public static final String EXECUTABLE_NAME_CONTEXT_KEY = "executable-name";
public static final String C_FLAGS_CONTEXT_KEY = "c-flags";
public static final String AS_FLAGS_CONTEXT_KEY = "as-flags";
public static final String CPP_FLAGS_CONTEXT_KEY = "cpp-flags";
public static final String LD_FLAGS_CONTEXT_KEY = "ld-flags";
public static final String LINKER_SCRIPT_CONTEXT_KEY = "linker-script";
private CompilerContextHelper() {
}
public static void setCPPFlags(final Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy