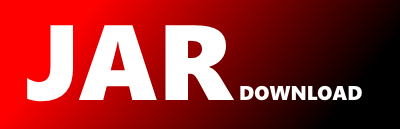
org.ow2.mind.AbstractADLCompiler Maven / Gradle / Ivy
The newest version!
/**
* Copyright (C) 2010 STMicroelectronics
*
* This file is part of "Mind Compiler" is free software: you can redistribute
* it and/or modify it under the terms of the GNU Lesser General Public License
* as published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS
* FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License for more
* details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program. If not, see .
*
* Contact: [email protected]
*
* Authors: Matthieu Leclercq
* Contributors:
*/
package org.ow2.mind;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import org.objectweb.fractal.adl.ADLException;
import org.objectweb.fractal.adl.Definition;
import org.ow2.mind.adl.graph.ComponentGraph;
import org.ow2.mind.compilation.CompilationCommand;
import org.ow2.mind.compilation.CompilationCommandExecutor;
import org.ow2.mind.compilation.CompilerCommand;
import org.ow2.mind.compilation.LinkerCommand;
import org.ow2.mind.error.ErrorManager;
import com.google.common.collect.Lists;
import com.google.inject.Inject;
public abstract class AbstractADLCompiler implements ADLCompiler {
@Inject
protected ErrorManager errorManager;
@Inject
protected CompilationCommandExecutor executor;
protected abstract void initContext(final String adlName,
final String execName, final CompilationStage stage,
final Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy