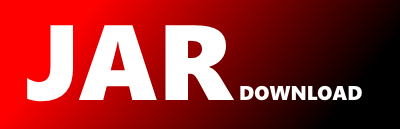
org.objectweb.util.monolog.wrapper.config.BasicLogger Maven / Gradle / Ivy
The newest version!
/**
* Copyright (C) 2001-2003 France Telecom R&D
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*/
package org.objectweb.util.monolog.wrapper.config;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Enumeration;
import java.util.HashMap;
import org.objectweb.util.monolog.api.Handler;
import org.objectweb.util.monolog.api.Level;
import org.objectweb.util.monolog.api.LevelFactory;
import org.objectweb.util.monolog.api.TopicalLogger;
/**
* This class is a basic implementatio of the TopicalLogger interface. It is not
* linked to any underlying log system. The log methods do nothing. Only the
* configuration aspect is treated. Therefore all the logger structure is
* stored into internal struture.
*
* @author Sebastien Chassande-Barrioz
*/
public class BasicLogger implements TopicalLogger, Serializable {
/**
* This fields references by their name the handlers associated to the
* logger.
* key = a handler name
* value = a Handler instance
*/
protected HashMap handlers = null;
/**
* The fields lists all topics the logger.
*/
protected ArrayList topics = null;
/**
* This field references the level factory.
*/
protected LevelFactory levelFactory = null;
boolean additivity = true;
/**
* The current level of the logger.
*/
protected Level level = null;
BasicLogger(String topic, LevelFactory lf) {
topics = new ArrayList();
handlers = new HashMap();
topics.add(topic);
levelFactory = lf;
}
// IMPLEMENTATION OF THE TopicalLogger INTERFACE //
//--------------------------------------------//
public void addHandler(Handler h) throws Exception {
handlers.put(h.getName(), h);
}
public void removeHandler(Handler h) throws Exception {
handlers.remove(h.getName());
}
public Handler[] getHandler() {
return (Handler[]) handlers.values().toArray(new Handler[0]);
}
public Handler getHandler(String hn) {
return (Handler) handlers.get(hn);
}
public void removeAllHandlers() throws Exception {
handlers.clear();
}
public void setAdditivity(boolean a) {
additivity = a;
}
public boolean getAdditivity() {
return additivity;
}
public void addTopic(String topic) throws Exception {
if (!topics.contains(topic)) {
topics.add(topic);
}
}
/**
* TODO
*/
public Enumeration getTopics() {
//TODO
return null;
}
public String[] getTopic() {
return (String[]) topics.toArray(new String[0]);
}
public void removeTopic(String topic) throws Exception {
topics.remove(topic);
}
// IMPLEMENTATION OF THE Handler INTERFACE //
//----------------------------------------//
public String getName() {
return (String) topics.get(0);
}
public void setName(String name) {
topics.set(0, name);
}
public String getType() {
return "logger";
}
public String[] getAttributeNames() {
return new String[0];
}
public Object getAttribute(String name) {
return null;
}
public Object setAttribute(String name, Object value) {
return null;
}
// IMPLEMENTATION OF THE Logger INTERFACE //
//----------------------------------------//
public void setIntLevel(int l) {
level = levelFactory.getLevel(l);
}
public void setLevel(Level l) {
level = l;
}
public int getCurrentIntLevel() {
return (level != null ? level.getIntValue() : 0);
}
public Level getCurrentLevel() {
return level;
}
public boolean isLoggable(int level) {
return false;
}
public boolean isLoggable(Level l) {
return false;
}
public boolean isOn() {
return false;
}
public void log(int level, Object message) {
}
public void log(Level level, Object message) {
}
public void log(int level, Object message, Throwable throwable) {
}
public void log(Level level, Object message, Throwable throwable) {
}
public void log(int level, Object message, Object location, Object method) {
}
public void log(Level l, Object message, Object location, Object method) {
}
public void log(int level, Object message, Throwable throwable,
Object location, Object method) {
}
public void log(Level level, Object message, Throwable throwable,
Object location, Object method) {
}
public void turnOn() {
}
public void turnOff() {
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy