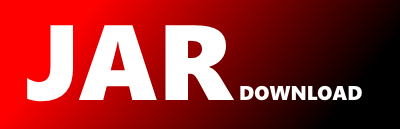
org.objectweb.util.monolog.wrapper.javaLog.Logger Maven / Gradle / Ivy
/**
* Copyright (C) 2001-2003 France Telecom R&D
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*/
package org.objectweb.util.monolog.wrapper.javaLog;
import java.util.Enumeration;
import org.objectweb.util.monolog.api.BasicLevel;
import org.objectweb.util.monolog.api.Handler;
import org.objectweb.util.monolog.api.Level;
import org.objectweb.util.monolog.wrapper.common.AbstractFactory;
/**
* Is an extension of the java.util.logging.Logger class used to wrap Monolog
* on the logging system provided in the JDK since the 1.4 version.
*
* @author S.Chassande-Barrioz
*/
public class Logger
extends java.util.logging.Logger
implements org.objectweb.util.monolog.api.TopicalLogger {
/**
* indicates if the logger is enabled
*/
boolean enabled = true;
/**
* This fieds is the real logger. In some cases inner is equal to this.
*/
protected java.util.logging.Logger inner = null;
/**
* Builds a a Logger with an inner logger
* @param inner the real logger
*/
protected Logger(java.util.logging.Logger inner) {
super(inner.getName(), inner.getResourceBundleName());
this.inner = inner;
}
/**
* Builds a Logger without inner logger (==> inner = this)
* @param name is the loggerName
* @param resName is the resource bundle name
*/
protected Logger(String name, String resName) {
super(name, resName);
this.inner = this;
}
// OVERRIDE THE java.util.logging.Logger CLASS //
//---------------------------------------------//
public void addHandler(java.util.logging.Handler handler) {
GenericHandler gh = null;
if (handler instanceof GenericHandler) {
gh = (GenericHandler) handler;
} else {
gh = new GenericHandler(handler.toString(), handler);
}
if (inner == this) {
super.addHandler(gh);
} else {
inner.addHandler(gh);
}
}
// IMPLEMENT THE Handler INTERFACE //
//---------------------------------//
/**
* It assigns the name of the handler
*/
public void setName(String name) {
}
/**
* It retrieves the Handler type
*/
public String getType() {
return "logger";
}
/**
* It retrieves the attributes of the handler
*/
public String[] getAttributeNames() {
return new String[0];
}
/**
* It retrieves the value of an attribute value of the handler.
* @param name is an attribute name
*/
public Object getAttribute(String name) {
return null;
}
/**
* It assigns an attributte to the handler.
* @param name is the attribute name
* @param value is the attribute value
* @return the old value is the attribute was already defined
*/
public Object setAttribute(String name, Object value) {
return null;
}
// IMPLEMENTATION OF org.objectweb.util.monolog.api.TopicalLogger INTERFACE //
//--------------------------------------------------------------------------//
/**
* A TopicalLogger manages a list of Handler instances. This method
* allows adding a handler to this list. The addHandler method returns
* true only if the Handler did not exist
*/
public void addHandler(Handler h) throws Exception {
if (inner == this) {
super.addHandler((GenericHandler) h);
if (AbstractFactory.debug) {
AbstractFactory.debug("logger(" + getName() + ").addHandler: "
+ h.getName() + "=>" + super.getHandlers().length);
}
} else {
inner.addHandler((GenericHandler) h);
if (AbstractFactory.debug) {
AbstractFactory.debug("logger(" + getName() + ").addHandler: "
+ h.getName() + "=>" + inner.getHandlers().length);
}
}
}
/**
* It returns the handler which the name is equals to the parameter
* @param hn is the handler name
* @return an Handler or a null value.
*/
public Handler getHandler(String hn) {
Object[] hs;
if (inner == this) {
hs = super.getHandlers();
} else {
hs = inner.getHandlers();
}
for (int i = 0; i < hs.length; i++) {
if (hs[i] instanceof Handler && hn.equals(((Handler) hs[i]).getName())) {
return (Handler) hs[i];
}
}
return null;
}
/**
* Get the Handlers associated with this logger.
*
* @return an array of all registered Handlers
*/
public synchronized Handler[] getHandler() {
Object[] os;
if (inner == this) {
os = super.getHandlers();
} else {
os = inner.getHandlers();
}
Handler[] hs = new Handler[os.length];
for(int i = 0; i < os.length; i++) {
if (os[i] instanceof Handler) {
hs[i] = (Handler) os[i];
} else {
hs[i] = new GenericHandler("", (java.util.logging.Handler) os[i]);
}
}
return hs;
}
/**
* A TopicalLogger manages a list of Handler instances. This method
* allows removing a handler to this list.
*/
public void removeHandler(Handler h) throws Exception {
if (inner == this) {
super.removeHandler((GenericHandler) h);
} else {
inner.removeHandler((GenericHandler) h);
}
}
/**
* A TopicalLogger manages a list of Handler instances. This method
* allows removing all handler.
*/
public void removeAllHandlers() throws Exception {
java.util.logging.Handler[] hs = inner.getHandlers();
if (AbstractFactory.debug) {
AbstractFactory.debug("logger(" + getName()
+ ").removeAllHandlers(): before: " + hs.length);
}
for (int i = 0; i < hs.length; i++) {
if (inner == this) {
super.removeHandler(hs[i]);
} else {
inner.removeHandler(hs[i]);
}
}
if (AbstractFactory.debug) {
AbstractFactory.debug("logger(" + getName()
+ ").removeAllHandlers(): before: "
+ inner.getHandlers().length);
}
}
/**
* It assigns the additivity flag for this logger instance.
*/
public void setAdditivity(boolean a) {
inner.setUseParentHandlers(a);
}
/**
* It retrieves the additivity flag for this logger instance.
*/
public boolean getAdditivity() {
return inner.getUseParentHandlers();
}
/**
*This method allows adding a topic to a TopicalLogger. This actions change
* the hierarchical structure, but also the list of handlers. The list of handlers
* of a TopicalLogger is composed of its handlers and all handlers inherited
* from its parents. Adding a topic changes the inherited handlers list.
*/
public void addTopic(String topic) throws Exception {
//TODO: support multiple topic
}
/**
*This method allows getting a topic list of this TopicalLogger.
*/
public String[] getTopic() {
//TODO: support multiple topic
return new String[] { AbstractFactory.getTopicWithoutPrefix(inner.getName()) };
}
/**
* This method allows getting a topic list of this TopicalLogger.
* Only kept for the backward compatibility.
*/
public Enumeration getTopics() {
//TODO:
return null;
}
/**
*This method allows removing a topic to a TopicalLogger. This actions change
* the hierarchical structure, but also the list of handlers. The list of handlers
* of a TopicalLogger is composed of its handlers and all handlers inherited
* from its parents. Removing a topic changes the inherited handlers list.
*/
public void removeTopic(String topic) throws Exception {
//TODO: support multiple topic
}
// IMPLEMENTATION OF org.objectweb.util.monolog.api.Logger INTERFACE //
//-------------------------------------------------------------------//
public void setIntLevel(int level) {
inner.setLevel(LevelImpl.int2Level(level));
}
public void setLevel(org.objectweb.util.monolog.api.Level l) {
inner.setLevel(LevelImpl.convertLevel(l));
}
public int getCurrentIntLevel() {
java.util.logging.Level l = getLevel();
return (l == null ? BasicLevel.INHERIT : l.intValue());
}
public Level getCurrentLevel() {
return LevelImpl.getLevel(getCurrentIntLevel());
}
public boolean isLoggable(int l) {
return enabled && super.isLoggable(LevelImpl.int2Level(l));
}
public boolean isLoggable(org.objectweb.util.monolog.api.Level l) {
return enabled && super.isLoggable(LevelImpl.convertLevel(l));
}
public boolean isOn() {
return enabled;
}
public void log(int l, Object o) {
inner.log(LevelImpl.int2Level(l), (o == null ? "null" : o.toString()));
}
public void log(Level l, Object o) {
inner.log(LevelImpl.convertLevel(l), (o == null ? "null" : o.toString()));
}
public void log(int l, Object o, Throwable t) {
inner.log(LevelImpl.int2Level(l), (o == null ? "null" : o.toString()), t);
}
public void log(Level l, Object o, Throwable t) {
inner.log(LevelImpl.convertLevel(l), (o == null ? "null" : o.toString()), t);
}
public void log(int level, Object o, Object location, Object method) {
inner.logp(LevelImpl.int2Level(level),
(location == null ? "null" : location.toString()),
(method == null ? "null" : method.toString()),
(o == null ? "null" : o.toString()));
}
public void log(Level l, Object o, Object location, Object method) {
inner.logp(LevelImpl.convertLevel(l),
(location == null ? "null" : location.toString()),
(method == null ? "null" : method.toString()),
(o == null ? "null" : o.toString()));
}
public void log(int level, Object o, Throwable t, Object location,
Object method) {
inner.logp(LevelImpl.int2Level(level),
(location == null ? "null" : location.toString()),
(method == null ? "null" : method.toString()),
(o == null ? "null" : o.toString()),
t);
}
public void log(Level l, Object o, Throwable t, Object location,
Object method) {
inner.logp(LevelImpl.convertLevel(l),
(location == null ? "null" : location.toString()),
(method == null ? "null" : method.toString()),
(o == null ? "null" : o.toString()),
t);
}
public void turnOn() {
enabled = true;
}
public void turnOff() {
enabled = false;
}
}