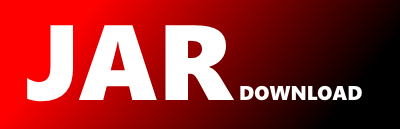
org.ow2.petals.admin.jmx.JMXArtifactAdministration Maven / Gradle / Ivy
/****************************************************************************
*
* Copyright (c) 2012, EBM WebSourcing
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA
*
*****************************************************************************/
package org.ow2.petals.admin.jmx;
import java.io.ByteArrayInputStream;
import java.util.ArrayList;
import java.util.List;
import javax.management.ObjectName;
import org.ow2.petals.admin.api.ArtifactAdministration;
import org.ow2.petals.admin.api.artifact.Artifact;
import org.ow2.petals.admin.api.artifact.ArtifactState.State;
import org.ow2.petals.admin.api.artifact.Component;
import org.ow2.petals.admin.api.artifact.Component.ComponentType;
import org.ow2.petals.admin.api.artifact.ServiceAssembly;
import org.ow2.petals.admin.api.artifact.ServiceUnit;
import org.ow2.petals.admin.api.artifact.SharedLibrary;
import org.ow2.petals.admin.api.artifact.lifecycle.ComponentLifecycle;
import org.ow2.petals.admin.api.artifact.lifecycle.ServiceAssemblyLifecycle;
import org.ow2.petals.admin.api.exception.ArtifactAdministrationException;
import org.ow2.petals.admin.api.exception.ArtifactNotFoundException;
import org.ow2.petals.admin.api.exception.ArtifactTypeIsNeededException;
import org.ow2.petals.admin.api.exception.UnsupportedArtifactTypeException;
import org.ow2.petals.admin.jmx.artifact.JMXArtifactLifecycleFactory;
import org.ow2.petals.jbi.descriptor.original.JBIDescriptorBuilder;
import org.ow2.petals.jbi.descriptor.original.generated.Jbi;
import org.ow2.petals.jmx.DeploymentServiceClient;
import org.ow2.petals.jmx.InstallationServiceClient;
import org.ow2.petals.jmx.JMXClient;
import org.ow2.petals.jmx.exception.AdminDoesNotExistException;
import org.ow2.petals.jmx.exception.AdminServiceErrorException;
import org.ow2.petals.jmx.exception.ConnectionErrorException;
import org.ow2.petals.jmx.exception.DeploymentServiceDoesNotExistException;
import org.ow2.petals.jmx.exception.DeploymentServiceErrorException;
import org.ow2.petals.jmx.exception.InstallationServiceDoesNotExistException;
import org.ow2.petals.jmx.exception.InstallationServiceErrorException;
/**
*
* @author Nicolas Oddoux - EBM WebSourcing
*/
public class JMXArtifactAdministration extends ArtifactAdministration {
protected JMXArtifactAdministration() {
super();
}
public void stopAndUndeployAllArtifacts() throws ArtifactAdministrationException {
try {
JMXClient client = JMXClientConnection.getJMXClient();
DeploymentServiceClient deploymentServiceClient = client.getDeploymentServiceClient();
deploymentServiceClient.stopAllServiceAssemblies();
deploymentServiceClient.shutdownAllServiceAssemblies();
deploymentServiceClient.undeployAllServiceAssemblies(true);
InstallationServiceClient installationServiceClient = client
.getInstallationServiceClient();
installationServiceClient.stopAllComponents();
installationServiceClient.shutdownAllComponents();
installationServiceClient.uninstallAllComponents();
installationServiceClient.unloadAllInstallers(true);
installationServiceClient.uninstallAllSharedLibrary();
} catch (ConnectionErrorException cee) {
throw new ArtifactAdministrationException(cee);
} catch (DeploymentServiceErrorException dsee) {
throw new ArtifactAdministrationException(dsee);
} catch (DeploymentServiceDoesNotExistException dsdnee) {
throw new ArtifactAdministrationException(dsdnee);
} catch (InstallationServiceDoesNotExistException isdnee) {
throw new ArtifactAdministrationException(isdnee);
} catch (InstallationServiceErrorException isee) {
throw new ArtifactAdministrationException(isee);
}
}
@Override
public List listArtifacts() throws ArtifactAdministrationException {
try {
JMXArtifactLifecycleFactory factory = new JMXArtifactLifecycleFactory();
final List artifacts = new ArrayList();
JMXClient client = JMXClientConnection.getJMXClient();
// Retrieve shared libraries
String[] sls = client.getInstallationServiceClient().getInstalledSharedLibraries();
for (String sl : sls) {
artifacts.add(new SharedLibrary(sl));
}
// Retrieve components BC and SE
ObjectName[] bindingComponents = client.getAdminServiceClient().getBindingComponents();
for (ObjectName bindingComponent : bindingComponents) {
String name = bindingComponent.getKeyProperty("name");
Component component = new Component(name, ComponentType.BC);
ComponentLifecycle componentLifecycle = factory.createComponentLifecycle(component);
componentLifecycle.updateState();
artifacts.add(component);
}
ObjectName[] serviceEngines = client.getAdminServiceClient().getEngineComponents();
for (ObjectName serviceEngine : serviceEngines) {
String name = serviceEngine.getKeyProperty("name");
Component component = new Component(name, ComponentType.SE);
ComponentLifecycle componentLifecycle = factory.createComponentLifecycle(component);
componentLifecycle.updateState();
artifacts.add(component);
}
// Retrieve components that have only their installer loaded
final String[] installers = client.getInstallationServiceClient().getInstallers();
for (final String installer : installers) {
boolean alreadyAdded = false;
for (final Artifact alreadyAddedArtifact : artifacts) {
// We check the name first because it is more differential
// than the type of artifact
if (alreadyAddedArtifact.getName().equals(installer)
&& alreadyAddedArtifact instanceof Component) {
alreadyAdded = true;
break;
}
}
if (!alreadyAdded) {
final Component component;
if (client.getAdminServiceClient().isBinding(installer)) {
component = new Component(installer, ComponentType.BC);
} else if (client.getAdminServiceClient().isEngine(installer)) {
component = new Component(installer, ComponentType.SE);
} else {
// This exception should not occurs
throw new ArtifactAdministrationException(
"Unable to determine the type of the component for installer '"
+ installer + "'.");
}
component.setState(State.LOADED);
artifacts.add(component);
}
}
// Retrieve service assemblies
String[] saNames = client.getDeploymentServiceClient().getDeployedServiceAssemblies();
for (String saName : saNames) {
ServiceAssembly serviceAssembly = createServiceAssembly(client, saName);
ServiceAssemblyLifecycle saLifecycle = factory
.createServiceAssemblyLifecycle(serviceAssembly);
saLifecycle.updateState();
artifacts.add(serviceAssembly);
}
return artifacts;
} catch (ConnectionErrorException cee) {
throw new ArtifactAdministrationException(cee);
} catch (AdminServiceErrorException asee) {
throw new ArtifactAdministrationException(asee);
} catch (AdminDoesNotExistException adnee) {
throw new ArtifactAdministrationException(adnee);
} catch (DeploymentServiceErrorException dsee) {
throw new ArtifactAdministrationException(dsee);
} catch (DeploymentServiceDoesNotExistException dsdnee) {
throw new ArtifactAdministrationException(dsdnee);
} catch (InstallationServiceErrorException e) {
throw new ArtifactAdministrationException(e);
} catch (InstallationServiceDoesNotExistException e) {
throw new ArtifactAdministrationException(e);
}
}
@Override
public Artifact getArtifact(String type, String name) throws UnsupportedArtifactTypeException,
ArtifactNotFoundException, ArtifactTypeIsNeededException,
ArtifactAdministrationException {
final Artifact artifact;
if (type != null) {
if (ComponentType.BC.toString().equals(type)) {
artifact = this.getArtifactBC(name);
} else if (ComponentType.SE.toString().equals(type)) {
artifact = this.getArtifactSE(name);
} else if (SharedLibrary.TYPE.equals(type)) {
artifact = this.getArtifactSL(name);
} else if (ServiceAssembly.TYPE.equals(type)) {
artifact = this.getArtifactSA(name);
} else {
throw new UnsupportedArtifactTypeException(type);
}
if (artifact == null) {
throw new ArtifactNotFoundException(type, name);
}
}
else {
final Artifact artifactBC = this.getArtifactBC(name);
final Artifact artifactSE = this.getArtifactSE(name);
final Artifact artifactSL = this.getArtifactSL(name);
final Artifact artifactSA = this.getArtifactSA(name);
if (artifactBC != null && artifactSE == null && artifactSL == null && artifactSA == null) {
artifact = artifactBC;
}
else if (artifactBC == null && artifactSE != null && artifactSL == null && artifactSA == null) {
artifact = artifactSE;
}
else if (artifactBC == null && artifactSE == null && artifactSL != null && artifactSA == null) {
artifact = artifactSL;
}
else if (artifactBC == null && artifactSE == null && artifactSL == null && artifactSA != null) {
artifact = artifactSA;
}
else if (artifactBC == null && artifactSE == null && artifactSL == null && artifactSA == null) {
throw new ArtifactNotFoundException(type, name);
}
else {
// More than one artifact match the name.
throw new ArtifactTypeIsNeededException(name);
}
}
return artifact;
}
private Artifact getArtifactBC(final String name) throws ArtifactAdministrationException {
try {
final JMXClient client = JMXClientConnection.getJMXClient();
final ObjectName[] bindingComponents = client.getAdminServiceClient()
.getBindingComponents();
for (final ObjectName bindingComponent : bindingComponents) {
final String bcName = bindingComponent.getKeyProperty("name");
if (bcName.equals(name)) {
return new Component(name, ComponentType.BC);
}
}
// Here, no BC with the given name is installed. We check if a
// component installer was only loaded.
if (client.getAdminServiceClient().isBinding(name)) {
// A component installer exists for this binding component
return new Component(name, ComponentType.BC);
} else {
return null;
}
} catch (final ConnectionErrorException e) {
throw new ArtifactAdministrationException(e);
} catch (final AdminServiceErrorException e) {
throw new ArtifactAdministrationException(e);
} catch (final AdminDoesNotExistException e) {
throw new ArtifactAdministrationException(e);
}
}
private Artifact getArtifactSE(final String name) throws ArtifactAdministrationException {
try {
final JMXClient client = JMXClientConnection.getJMXClient();
final ObjectName[] bindingComponents = client.getAdminServiceClient()
.getEngineComponents();
for (final ObjectName bindingComponent : bindingComponents) {
final String seName = bindingComponent.getKeyProperty("name");
if (seName.equals(name)) {
return new Component(name, ComponentType.SE);
}
}
// Here, no SE with the given name is installed. We check if a
// component installer was only loaded.
if (client.getAdminServiceClient().isEngine(name)) {
// A component installer exists for this service engine
return new Component(name, ComponentType.SE);
} else {
return null;
}
} catch (final ConnectionErrorException e) {
throw new ArtifactAdministrationException(e);
} catch (AdminServiceErrorException e) {
throw new ArtifactAdministrationException(e);
} catch (AdminDoesNotExistException e) {
throw new ArtifactAdministrationException(e);
}
}
private Artifact getArtifactSL(final String name) throws ArtifactAdministrationException {
try {
final JMXClient client = JMXClientConnection.getJMXClient();
final String[] sls = client.getInstallationServiceClient()
.getInstalledSharedLibraries();
for (final String sl : sls) {
if (sl.equals(name)) {
return new SharedLibrary(name);
}
}
return null;
} catch (final ConnectionErrorException e) {
throw new ArtifactAdministrationException(e);
} catch (final InstallationServiceErrorException e) {
throw new ArtifactAdministrationException(e);
} catch (final InstallationServiceDoesNotExistException e) {
throw new ArtifactAdministrationException(e);
}
}
private Artifact getArtifactSA(final String name) throws ArtifactAdministrationException {
try {
final JMXClient client = JMXClientConnection.getJMXClient();
final String[] saNames = client.getDeploymentServiceClient()
.getDeployedServiceAssemblies();
for (final String saName : saNames) {
if (saName.equals(name)) {
return createServiceAssembly(client, saName);
}
}
return null;
} catch (final ConnectionErrorException e) {
throw new ArtifactAdministrationException(e);
} catch (final DeploymentServiceErrorException e) {
throw new ArtifactAdministrationException(e);
} catch (final DeploymentServiceDoesNotExistException e) {
throw new ArtifactAdministrationException(e);
}
}
private ServiceAssembly createServiceAssembly(JMXClient client, String saName)
throws ArtifactAdministrationException {
try {
String descriptor;
List serviceUnits;
descriptor = client.getDeploymentServiceClient().getServiceAssemblyDescriptor(saName);
ByteArrayInputStream bais = new ByteArrayInputStream(descriptor.getBytes());
Jbi saJbiDescriptor = JBIDescriptorBuilder.buildJavaJBIDescriptor(bais);
bais.close();
serviceUnits = new ArrayList();
List suDescriptors = saJbiDescriptor
.getServiceAssembly().getServiceUnit();
for (org.ow2.petals.jbi.descriptor.original.generated.ServiceUnit suDescriptor : suDescriptors) {
String suName = suDescriptor.getIdentification().getName();
String suTargetComponentName = suDescriptor.getTarget().getComponentName();
ServiceUnit serviceUnit = new ServiceUnit(suName, "NOVERSION",
suTargetComponentName);
serviceUnits.add(serviceUnit);
}
return new ServiceAssembly(saName, serviceUnits);
} catch (Exception e) {
throw new ArtifactAdministrationException(e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy