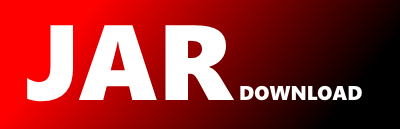
org.ow2.petals.admin.jmx.artifact.JMXServiceAssemblyLifecycle Maven / Gradle / Ivy
/****************************************************************************
*
* Copyright (c) 2012, EBM WebSourcing
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA
*
*****************************************************************************/
package org.ow2.petals.admin.jmx.artifact;
import java.net.URL;
import java.util.logging.Logger;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import org.ow2.petals.admin.api.artifact.ArtifactState.State;
import org.ow2.petals.admin.api.artifact.ServiceAssembly;
import org.ow2.petals.admin.api.artifact.lifecycle.ServiceAssemblyLifecycle;
import org.ow2.petals.admin.api.exception.ArtifactAdministrationException;
import org.ow2.petals.admin.api.exception.ArtifactDeployedException;
import org.ow2.petals.admin.api.exception.ArtifactNotDeployedException;
import org.ow2.petals.admin.api.exception.ArtifactNotFoundException;
import org.ow2.petals.admin.api.exception.ArtifactStartedException;
import org.ow2.petals.admin.api.exception.ArtifactStoppedException;
import org.ow2.petals.admin.jmx.JMXClientConnection;
import org.ow2.petals.jmx.DeploymentServiceClient;
import org.ow2.petals.jmx.JMXClient;
import org.ow2.petals.jmx.exception.ConnectionErrorException;
import org.ow2.petals.jmx.exception.DeploymentServiceDoesNotExistException;
import org.ow2.petals.jmx.exception.DeploymentServiceErrorException;
import org.ow2.petals.jmx.exception.ServiceAssemblyDoesNotExistException;
/**
* This class represents a remote JBI service assembly.
*
* @author Nicolas Oddoux - EBM WebSourcing
*/
public class JMXServiceAssemblyLifecycle extends ServiceAssemblyLifecycle {
private static final Logger LOG = Logger.getLogger(JMXServiceAssemblyLifecycle.class.getName());
private static final String SA_SUCCESS_RESULT = "SUCCESS";
private static final Pattern SA_RESULT_PATTERN = Pattern
.compile(
".+?.+?(\\w+?) .+?(.+?) ",
Pattern.DOTALL);
private static final Pattern SU_RESULT_PATTERN = Pattern
.compile(
".+?.+?FAILED .+?(.+?) .+?(.+?) ",
Pattern.DOTALL);
protected JMXServiceAssemblyLifecycle(ServiceAssembly sa) {
super(sa);
}
/**
* {@inheritDoc}
*
* Special note for service assembly deployment:
*
* - if the service assembly is in state 'SHUTDOWN', it will be undeployed
* before to be be redeplyed,
* - otherwise an error is thrown.
*
*
*/
@Override
public void deploy(URL artifactUrl) throws ArtifactDeployedException,
ArtifactAdministrationException {
try {
final JMXClient client = JMXClientConnection.getJMXClient();
final String saName = this.getServiceAssembly().getName();
final DeploymentServiceClient dplSvcClt = client.getDeploymentServiceClient();
try {
final DeploymentServiceClient.State state = dplSvcClt.getState(saName);
if (state != DeploymentServiceClient.State.SHUTDOWN) {
throw new ArtifactDeployedException(saName, state.toString());
} else {
LOG.warning("The service assembly is in state '"
+ DeploymentServiceClient.State.SHUTDOWN.name()
+ "', it has been automatically undeployed before to be re-deployed.");
checkResult(client.getDeploymentServiceClient().undeploy(saName), saName,
"Failed to undeploy SA '%s'");
}
} catch (final ServiceAssemblyDoesNotExistException e) {
// The service assembly is not already deployed
}
checkResult(client.getDeploymentServiceClient().deploy(artifactUrl),
"Failed to deploy SU '%s':\n%s",
"SA '%s' deployed with some SU deployment in failure",
"Failed to deploy SA '%s'");
} catch (final ConnectionErrorException cee) {
throw new ArtifactAdministrationException(cee);
} catch (final DeploymentServiceErrorException dsee) {
throw new ArtifactAdministrationException(dsee);
} catch (final DeploymentServiceDoesNotExistException dsdnee) {
throw new ArtifactAdministrationException(dsdnee);
}
}
/**
* {@inheritDoc}
*
* Special note for service assembly start:
*
* - if the SA is in state 'STARTED', the error
* {@link ArtifactStartedException} is raised,
* - if the SA is in state 'STOPPED', it will be started,
* - if the SA is in state 'SHUTDOWN', it will be started,
* - if the SA is not deployed, the error
* {@link ArtifactNotFoundException} is raised.
*
*
*/
@Override
public void start() throws ArtifactStartedException, ArtifactNotDeployedException,
ArtifactNotFoundException, ArtifactAdministrationException {
try {
final JMXClient client = JMXClientConnection.getJMXClient();
final String saName = this.getServiceAssembly().getName();
final DeploymentServiceClient dplSvcClt = client.getDeploymentServiceClient();
try {
final DeploymentServiceClient.State state = dplSvcClt.getState(saName);
if (state == DeploymentServiceClient.State.STARTED) {
throw new ArtifactStartedException(this.getServiceAssembly().getType(), saName);
} else {
checkResult(client.getDeploymentServiceClient().start(saName), saName,
"Failed to start SA '%s'");
}
} catch (final ServiceAssemblyDoesNotExistException e) {
throw new ArtifactNotFoundException(this.getServiceAssembly().getType(), saName);
}
} catch (ConnectionErrorException cee) {
throw new ArtifactAdministrationException(cee);
} catch (DeploymentServiceErrorException dsee) {
throw new ArtifactAdministrationException(dsee);
} catch (DeploymentServiceDoesNotExistException dsdnee) {
throw new ArtifactAdministrationException(dsdnee);
}
}
/**
* {@inheritDoc}
*
* Special note for service assembly stop:
*
* - if the SA is in state 'STARTED', it will be stop,
* - if the SA is in state 'STOPPED', the error
* {@link ArtifactStoppedException} is raised,
* - if the SA is in state 'SHUTDOWN', the error
* {@link ArtifactStoppedException} is raised,
* - if the SA is not deployed, the error
* {@link ArtifactNotFoundException} is raised.
*
*
*/
@Override
public void stop() throws ArtifactStoppedException, ArtifactNotFoundException,
ArtifactAdministrationException {
try {
final JMXClient client = JMXClientConnection.getJMXClient();
final String saName = this.getServiceAssembly().getName();
final DeploymentServiceClient dplSvcClt = client.getDeploymentServiceClient();
try {
final DeploymentServiceClient.State state = dplSvcClt.getState(saName);
if (state == DeploymentServiceClient.State.STOPPED
|| state == DeploymentServiceClient.State.SHUTDOWN) {
throw new ArtifactStoppedException(this.getServiceAssembly().getType(), saName);
} else {
checkResult(client.getDeploymentServiceClient().stop(saName), saName,
"Failed to stop SA '%s'");
}
} catch (final ServiceAssemblyDoesNotExistException e) {
throw new ArtifactNotFoundException(this.getServiceAssembly().getType(), saName);
}
} catch (final ConnectionErrorException cee) {
throw new ArtifactAdministrationException(cee);
} catch (final DeploymentServiceErrorException dsee) {
throw new ArtifactAdministrationException(dsee);
} catch (final DeploymentServiceDoesNotExistException dsdnee) {
throw new ArtifactAdministrationException(dsdnee);
}
}
/**
* {@inheritDoc}
*
* Special note for service assembly undeployment:
*
* - if the SA is in state 'STARTED', the error
* {@link ArtifactStartedException} is raised,
* - if the SA is in state 'STOPPED', the SA will be shutdown and
* undeployed,
* - if the SA is in state 'SHUTDOWN', the SA will be undeployed.
* - if the SA is not deployed, the error
* {@link ArtifactNotFoundException} is raised.
*
*
*/
@Override
public void undeploy() throws ArtifactStartedException, ArtifactNotFoundException,
ArtifactAdministrationException {
try {
final JMXClient client = JMXClientConnection.getJMXClient();
final String saName = this.getServiceAssembly().getName();
final DeploymentServiceClient dplSvcClt = client.getDeploymentServiceClient();
try {
final DeploymentServiceClient.State state = dplSvcClt.getState(saName);
if (state == DeploymentServiceClient.State.STARTED) {
throw new ArtifactStartedException(this.getServiceAssembly().getType(), saName);
}
else if (state == DeploymentServiceClient.State.STOPPED) {
checkResult(dplSvcClt.shutdown(saName), saName, "Failed to shutdown SA '%s'");
checkResult(dplSvcClt.undeploy(saName), "Failed to undeploy SU '%s':\n%s",
"SA '%s' undeployed with some SU undeployment in failure",
"Failed to undeploy SA '%s'");
}
else if (state == DeploymentServiceClient.State.SHUTDOWN) {
checkResult(dplSvcClt.undeploy(saName), "Failed to undeploy SU '%s':\n%s",
"SA '%s' undeployed with some SU undeployment in failure",
"Failed to undeploy SA '%s'");
} else {
throw new ArtifactAdministrationException("The artifact '" + saName
+ "' is in an unknown state '" + state + "'.");
}
} catch (final ServiceAssemblyDoesNotExistException e) {
throw new ArtifactNotFoundException(this.getServiceAssembly().getType(), saName);
}
} catch (ConnectionErrorException cee) {
throw new ArtifactAdministrationException(cee);
} catch (DeploymentServiceErrorException dsee) {
throw new ArtifactAdministrationException(dsee);
} catch (DeploymentServiceDoesNotExistException dsdnee) {
throw new ArtifactAdministrationException(dsdnee);
}
}
private static final void checkResult(String serviceAssemblyReport,
String suErrorMessageFormat, String saPartialErrorMessageFormat,
String saErrorMessageFormat) throws ArtifactAdministrationException {
boolean isFailed = false;
StringBuilder errorMessage = new StringBuilder();
Matcher matcher = SU_RESULT_PATTERN.matcher(serviceAssemblyReport);
if (matcher.find()) {
isFailed = true;
errorMessage.append(String.format(suErrorMessageFormat, matcher.group(1),
matcher.group(2)));
}
matcher = SA_RESULT_PATTERN.matcher(serviceAssemblyReport);
if (matcher.find()) {
if (SA_SUCCESS_RESULT.equals(matcher.group(1).toUpperCase())) {
if (isFailed) {
errorMessage
.append(String.format(saPartialErrorMessageFormat, matcher.group(2)));
}
} else {
isFailed = true;
errorMessage.append(String.format(saErrorMessageFormat, matcher.group(2)));
}
}
if (isFailed) {
throw new ArtifactAdministrationException(errorMessage.toString());
}
}
private static final void checkResult(String serviceAssemblyReport, String errorMessageFormat,
String saName) throws ArtifactAdministrationException {
final Matcher matcher = SA_RESULT_PATTERN.matcher(serviceAssemblyReport);
if (matcher.find()) {
if (!SA_SUCCESS_RESULT.equals(matcher.group(1).toUpperCase())) {
String errorMessage = String.format(errorMessageFormat, saName);
throw new ArtifactAdministrationException(errorMessage);
}
}
}
@Override
public void updateState() throws ArtifactAdministrationException {
try {
JMXClient client = JMXClientConnection.getJMXClient();
String serviceAssemblyName = this.getServiceAssembly().getName();
final DeploymentServiceClient.State state = client.getDeploymentServiceClient()
.getState(serviceAssemblyName);
this.getServiceAssembly().setState(State.fromStringValue(state.toString()));
} catch (final ConnectionErrorException cee) {
throw new ArtifactAdministrationException(cee);
} catch (final DeploymentServiceErrorException dsee) {
throw new ArtifactAdministrationException(dsee);
} catch (final DeploymentServiceDoesNotExistException dsdnee) {
throw new ArtifactAdministrationException(dsdnee);
} catch (final ServiceAssemblyDoesNotExistException e) {
throw new ArtifactAdministrationException(e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy