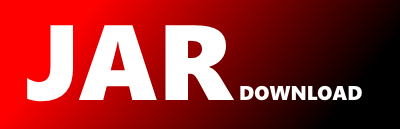
org.ow2.petals.admin.jmx.registry.JMXRegistryView Maven / Gradle / Ivy
/****************************************************************************
*
* Copyright (c) 2010-2012, EBM WebSourcing
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA
*
*****************************************************************************/
package org.ow2.petals.admin.jmx.registry;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import java.util.regex.PatternSyntaxException;
import org.ow2.petals.admin.api.exception.RegistryRegexpPatternException;
import org.ow2.petals.admin.registry.Endpoint;
import org.ow2.petals.admin.registry.Endpoint.EndpointType;
import org.ow2.petals.admin.registry.RegistryView;
import org.ow2.petals.jmx.commons.PetalsEndpointRegistryServiceConstants;
/**
*
* @author Nicolas Oddoux - EBM WebSourcing
*/
public class JMXRegistryView implements RegistryView {
private final List endpoints;
private final Map> endpointsByServiceName;
private final Map> endpointsByInterfaceName;
public JMXRegistryView(List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy