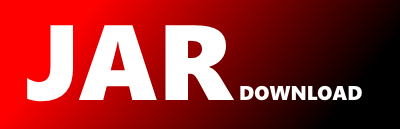
org.ow2.petals.admin.jmx.topology.TopologyUtils Maven / Gradle / Ivy
/****************************************************************************
*
* Copyright (c) 2010-2012, EBM WebSourcing
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA
*
*****************************************************************************/
package org.ow2.petals.admin.jmx.topology;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Set;
import org.ow2.petals.admin.topology.Container;
import org.ow2.petals.admin.topology.Container.NodeType;
import org.ow2.petals.admin.topology.Container.PortType;
import org.ow2.petals.admin.topology.Domain;
import org.ow2.petals.admin.topology.Subdomain;
import org.ow2.petals.jmx.commons.PetalsAdminServiceConstants;
import org.ow2.petals.jmx.commons.PetalsAdminServiceConstants.Topology;
import com.ebmwebsourcing.easycommons.lang.UncheckedException;
/**
*
* @author Nicolas Oddoux - EBM WebSourcing
*/
public class TopologyUtils {
private static final Map portTypes;
static {
HashMap tempPortTypes = new HashMap();
tempPortTypes.put(PetalsAdminServiceConstants.Container.CONF_WEBSERVICE_HTTP_PORT, PortType.HTTP_WEBSERVICE);
tempPortTypes.put(PetalsAdminServiceConstants.Container.CONF_JMX_RMI_PORT, PortType.JMX);
tempPortTypes.put(PetalsAdminServiceConstants.Container.CONF_TRANSPORT_TCP_PORT, PortType.TCP_TRANSPORT);
tempPortTypes.put(PetalsAdminServiceConstants.Container.CONF_REGISTRY_PORT, PortType.REGISTRY);
portTypes = Collections.unmodifiableMap(tempPortTypes);
}
public static final Domain createListOfDomains(Set
© 2015 - 2025 Weber Informatics LLC | Privacy Policy