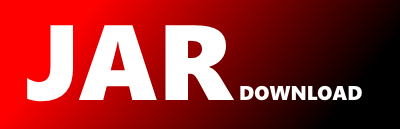
org.ow2.petals.admin.jmx.topology.TopologyUtils Maven / Gradle / Ivy
/**
* Copyright (c) 2012 EBM WebSourcing, 2012-2016 Linagora
*
* This program/library is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 2.1 of the License, or (at your
* option) any later version.
*
* This program/library is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License
* for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program/library; If not, see http://www.gnu.org/licenses/
* for the GNU Lesser General Public License version 2.1.
*/
package org.ow2.petals.admin.jmx.topology;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Set;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import org.apache.commons.collections4.BidiMap;
import org.apache.commons.collections4.bidimap.DualHashBidiMap;
import org.ow2.petals.admin.topology.Container;
import org.ow2.petals.admin.topology.Container.PortType;
import org.ow2.petals.admin.topology.Container.State;
import org.ow2.petals.admin.topology.Domain;
import org.ow2.petals.clientserverapi.topology.TopologyService;
import org.ow2.petals.clientserverapi.topology.TopologyService.ContainerPropertyValues;
import com.ebmwebsourcing.easycommons.lang.UncheckedException;
/**
*
* @author Nicolas Oddoux - EBM WebSourcing
*/
public class TopologyUtils {
private static final BidiMap PORT_TYPE_CONVERTER = new DualHashBidiMap();
private static final BidiMap STATE_VALUE_CONVERTER = new DualHashBidiMap();
static {
PORT_TYPE_CONVERTER.put(TopologyService.ContainerPropertyName.CONF_JMX_RMI_PORT, PortType.JMX);
PORT_TYPE_CONVERTER.put(TopologyService.ContainerPropertyName.CONF_TRANSPORT_TCP_PORT, PortType.TCP_TRANSPORT);
STATE_VALUE_CONVERTER.put(ContainerPropertyValues.STATE_REACHABLE, State.REACHABLE);
STATE_VALUE_CONVERTER.put(ContainerPropertyValues.STATE_UNREACHABLE, State.UNREACHABLE);
}
/**
*
* Create a {@link Domain} according to:
*
* - the provided topology,
* - a regexp filtering container to add.
*
*
*
* @param containerFilterRegexp
* A container name regexp to filter the containers to return
* @return A complete domain definition or limited to the provided filters.
*/
public static final Domain createListOfDomains(final Set
© 2015 - 2025 Weber Informatics LLC | Privacy Policy