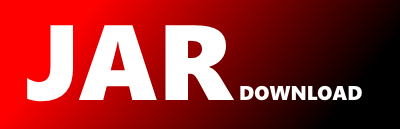
org.ow2.petals.ant.AbstractConfigureArchiveAntTask Maven / Gradle / Ivy
/**
* Copyright (c) 2008-2012 EBM WebSourcing, 2012-2016 Linagora
*
* This program/library is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 2.1 of the License, or (at your
* option) any later version.
*
* This program/library is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License
* for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program/library; If not, see http://www.gnu.org/licenses/
* for the GNU Lesser General Public License version 2.1.
*/
package org.ow2.petals.ant;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import java.net.MalformedURLException;
import java.net.URISyntaxException;
import java.net.URL;
import org.apache.tools.ant.BuildException;
import org.apache.tools.ant.Task;
import org.ow2.petals.ant.util.NioUtil;
/**
* Abstract class for all tasks configuring an archive.
* @author Roland Naudin - EBM WebSourcing
*/
public class AbstractConfigureArchiveAntTask extends Task {
/**
* Ant task attribute 'file' locating the archive to configure.
*/
protected String file;
/**
* Ant task attribute 'outputFile' defined the filename of the archive
* configured.
*/
protected String outputFile;
/**
* Overwrite the output archive
*/
protected boolean overwriteOutputFile = true;
@Override
public void execute() throws BuildException {
super.execute();
final URL outputFileURL = validateFileParameter(this.outputFile, "outputFile");
final File outputFileFile;
try {
outputFileFile = new File(outputFileURL.toURI());
if (!this.overwriteOutputFile && outputFileFile.exists()) {
throw new BuildException("The output component archive '" + this.outputFile
+ "' already exists.");
}
} catch (final URISyntaxException e) {
// This exception should not occur.
throw new BuildException(e);
}
}
/**
* Download the given URL into a File.
*
* @param componentURL
* @return
* @throws IOException
*/
protected static final File downloadURL(final URL componentURL) throws IOException {
final String path = componentURL.getPath();
final String fileName = path.substring(path.lastIndexOf('/') + 1);
final File outputFile = File.createTempFile(fileName, null);
final InputStream inputStream = componentURL.openStream();
try {
NioUtil.copyStreamToFile(inputStream, outputFile);
} finally {
inputStream.close();
}
return outputFile;
}
/**
* Validate the file parameter. The file parameter should not be null and
* must be a jar or a zip archive.
*
* @throws BuildException
*/
protected static final URL validateFileParameter(final String fileParameter, final String paramaterName)
throws BuildException {
if (fileParameter == null) {
throw new BuildException("Missing attribute '" + paramaterName + "'");
}
if (fileParameter.isEmpty()) {
throw new BuildException("Empty attribute '" + paramaterName + "'");
}
if (!fileParameter.endsWith(".zip") && !fileParameter.endsWith(".jar")) {
throw new BuildException("File '" + fileParameter
+ "' is not a valid archive (zip or jar required)");
}
URL fileURL;
try {
fileURL = new URL(fileParameter);
} catch (MalformedURLException e) {
try {
fileURL = new File(fileParameter).toURI().toURL();
} catch (final MalformedURLException e1) {
throw new BuildException("Wrong format for attribute '" + paramaterName
+ "', a valid file location is required");
}
}
return fileURL;
}
/**
* File getter.
*
* @return
*/
public String getFile() {
return file;
}
/**
* File setter.
*
* @param file
*/
public void setFile(final String file) {
this.file = file;
}
/**
* OutputFile getter.
*
* @return
*/
public String getOutputFile() {
return outputFile;
}
/**
* OutputFile setter.
*
* @param outputFile
*/
public void setOutputFile(final String outputFile) {
this.outputFile = outputFile;
}
/**
* @return the overwriteOutputFile
*/
public boolean isOverwriteOutputFile() {
return this.overwriteOutputFile;
}
/**
* @param overwriteOutputFile
* the overwriteOutputFile to set
*/
public void setOverwriteOutputFile(final boolean overwriteOutputFile) {
this.overwriteOutputFile = overwriteOutputFile;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy