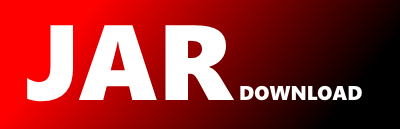
org.ow2.petals.ant.AbstractJBIAntTask Maven / Gradle / Ivy
/**
* Copyright (c) 2005-2012 EBM WebSourcing, 2012-2016 Linagora
*
* This program/library is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 2.1 of the License, or (at your
* option) any later version.
*
* This program/library is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License
* for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program/library; If not, see http://www.gnu.org/licenses/
* for the GNU Lesser General Public License version 2.1.
*/
package org.ow2.petals.ant;
import java.util.regex.Pattern;
import org.apache.tools.ant.BuildException;
import org.apache.tools.ant.Task;
import org.apache.tools.ant.types.LogLevel;
import org.ow2.petals.jmx.api.api.JMXClient;
import org.ow2.petals.jmx.api.api.PetalsJmxApiFactory;
import org.ow2.petals.jmx.api.api.exception.ConnectionErrorException;
import org.ow2.petals.jmx.api.api.exception.DuplicatedServiceException;
import org.ow2.petals.jmx.api.api.exception.MissingServiceException;
/**
* This class is used to give the main parameters of the Ant tasks.
* @author ddesjardins - EBM WebSourcing
* @author Christophe Hamerling - EBM WebSourcing
*/
public abstract class AbstractJBIAntTask extends Task {
public static final String DEFAULT_JMX_HOST = "localhost";
public static final String DEFAULT_JMX_PORT = "7700";
/**
* Pattern of a service-assembly (un)deployment report about a failed service-assembly (un)deployment because of the
* service-assembly, not because of its service-units.
*/
public static final Pattern saNamepattern = Pattern
.compile(
".+?.+?(\\w+?) .+?(.+?) ",
Pattern.DOTALL);
/**
* Pattern of the service-assembly (un)deployment report about a failed service-unit (un)deployment.
*/
public static final Pattern suResultpattern = Pattern
.compile(
".+?.+?FAILED .+?(.+?) .+?(.+?) ",
Pattern.DOTALL);
/**
* Fail on error
*/
protected boolean failOnError = true;
/**
* Host jmx
*/
protected String host = DEFAULT_JMX_HOST;
/**
* Password for security
*/
protected String password;
/**
* Port jmx
*/
protected String port = DEFAULT_JMX_PORT;
/**
* User name for security
*/
protected String username;
/**
* Do the task job
*
* @throws Exception
*/
public abstract void doTask() throws Exception;
@Override
public void execute() throws BuildException {
try {
doTask();
} catch (final Throwable e) {
if (isFailOnError()) {
throw new BuildException(e);
}
} finally {
if (jmxClient != null) {
try {
jmxClient.disconnect();
} catch (ConnectionErrorException e) {
this.log(e, LogLevel.ERR.getLevel());
}
}
}
}
/**
* @return the host
*/
public String getHost() {
return host;
}
/**
* An instance of a JMX client lazily initialised if used by the task
*/
private JMXClient jmxClient = null;
/**
* Get a connection to JMX
*
* @return
* @throws ConnectionErrorException
* @throws NumberFormatException
* @throws MissingServiceException
* @throws DuplicatedServiceException
*/
public JMXClient getJMXClient() throws NumberFormatException, ConnectionErrorException,
DuplicatedServiceException, MissingServiceException {
if (jmxClient == null) {
// this method returns a new instance everytime
jmxClient = PetalsJmxApiFactory.getInstance().createJMXClient(host, Integer.valueOf(port), username,
password);
}
return jmxClient;
}
/**
* @return the password
*/
public String getPassword() {
return password;
}
/**
* @return the port
*/
public String getPort() {
return port;
}
/**
* @return the username
*/
public String getUsername() {
return username;
}
/**
* @return the failOnError
*/
public boolean isFailOnError() {
return failOnError;
}
/**
* @param failOnError
* the failOnError to set
*/
public void setFailOnError(final boolean failOnError) {
this.failOnError = failOnError;
}
/**
* @param host
* the host to set
*/
public void setHost(final String host) {
this.host = host;
}
/**
* @param password
* the password to set
*/
public void setPassword(final String password) {
this.password = password;
}
/**
* @param port
* the port to set
*/
public void setPort(final String port) {
this.port = port;
}
/**
* @param username
* the username to set
*/
public void setUsername(final String username) {
this.username = username;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy