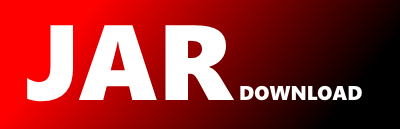
org.ow2.petals.ant.task.ConfigureRuntimeComponentTask Maven / Gradle / Ivy
/**
* Copyright (c) 2005-2012 EBM WebSourcing, 2012-2016 Linagora
*
* This program/library is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 2.1 of the License, or (at your
* option) any later version.
*
* This program/library is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License
* for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program/library; If not, see http://www.gnu.org/licenses/
* for the GNU Lesser General Public License version 2.1.
*/
package org.ow2.petals.ant.task;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.util.ArrayList;
import java.util.Hashtable;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Properties;
import java.util.Set;
import javax.management.MBeanAttributeInfo;
import org.apache.tools.ant.BuildException;
import org.apache.tools.ant.types.LogLevel;
import org.ow2.petals.ant.AbstractJBIAntTask;
import org.ow2.petals.jmx.api.api.configuration.component.RuntimeConfigurationComponentClient;
/**
* Ant task : petals--configure-runtime-component.
*
* This task allow to update JBI component attributes at runtime (service engine or binding component) into
* the JBI environment.
*
*
* @author Adrien Ruffie - EBM WebSourcing
*/
public class ConfigureRuntimeComponentTask extends AbstractJBIAntTask {
/**
* Parameter class for nested elements
*
* @author chamerling
*
*/
public static class Param {
/**
* Name attribute
*/
private String name;
/**
* Value attribute
*/
private String value;
/**
* Creates a new instance of {@link Param}
*
*/
public Param() {
this("", "");
}
/**
* Creates a new instance of {@link Param}
*
* @param name
* @param value
*/
public Param(final String name, final String value) {
this.name = name;
this.value = value;
}
/**
* @return the name
*/
public String getName() {
return name;
}
/**
* @return the value
*/
public String getValue() {
return value;
}
/**
* @param name
* the name to set
*/
public void setName(final String name) {
this.name = name;
}
/**
* @param value
* the value to set
*/
public void setValue(final String value) {
this.value = value;
}
}
private static final Map> primitiveClasses = new Hashtable>(12);
/**
* Name of the JBI component
*/
private String name;
/**
* The nested elements
*/
private List nestedParams = new ArrayList();
/**
* Location of the params file that contains MBean properties
*/
private String params;
/**
* Creates a new instance of {@link ConfigureRuntimeComponentTask}
*
*/
public ConfigureRuntimeComponentTask() {
super();
}
static {
primitiveClasses.put(Boolean.class.getName(), Boolean.class);
primitiveClasses.put(Boolean.TYPE.getName(), Boolean.class);
primitiveClasses.put(Short.class.getName(), Short.class);
primitiveClasses.put(Short.TYPE.getName(), Short.class);
primitiveClasses.put(Integer.class.getName(), Integer.class);
primitiveClasses.put(Integer.TYPE.getName(), Integer.class);
primitiveClasses.put(Long.class.getName(), Long.class);
primitiveClasses.put(Long.TYPE.getName(), Long.class);
primitiveClasses.put(Float.class.getName(), Float.class);
primitiveClasses.put(Float.TYPE.getName(), Float.class);
primitiveClasses.put(Double.class.getName(), Double.class);
primitiveClasses.put(Double.TYPE.getName(), Double.class);
}
/**
*
* @return
*/
public Param createParam() {
final Param param = new Param();
nestedParams.add(param);
return param;
}
@Override
public void doTask() throws Exception {
if (this.name == null) {
throw new BuildException("Missing attribute 'name'");
}
final Properties paramsProperties = loadProperties();
final RuntimeConfigurationComponentClient rccc = this.getJMXClient().getComponentClient(this.name)
.getRuntimeConfigurationClient();
this.log(this.nestedParams.size() + paramsProperties.size()
+ " runtime parameters will be set to the component '" + this.name + "'");
if (rccc != null) {
final Map runtimeAttributes = rccc
.getConfigurationMBeanAttributes();
setRuntimeAttributes(runtimeAttributes, nestedParams, paramsProperties);
rccc.setConfigurationMBeanAttributes(runtimeAttributes);
log("Runtime configuration of the component '" + this.name + "' set");
} else {
this.log("No runtime configuration MBean proposed by the runtime MBean!", LogLevel.WARN
.getLevel());
}
}
/**
* @return the nestedParams
*/
public List getNestedParams() {
return nestedParams;
}
/**
* Set the name parameter
*
* @param name
*/
public void setName(final String name) {
this.name = name;
}
/**
* @param nestedParams
* the nestedParams to set
*/
public void setNestedParams(final List nestedParams) {
this.nestedParams = nestedParams;
}
/**
* @nestedParams params the params to set
*/
public void setParams(final String params) {
this.params = params;
}
/**
* Id the nested params contains this param?
*
* @param nestedParams
* @param name
* @return
*/
private Param containParam(final List nestedParams, final String name) {
for (final Param p : nestedParams) {
if (p.getName().equals(name)) {
return p;
}
}
return null;
}
/**
* Is the properties params contains this param?
*
* @param props
* @param name
* @return
*/
private Param containParam(final Properties props, final String name) {
for (final Entry
© 2015 - 2025 Weber Informatics LLC | Privacy Policy