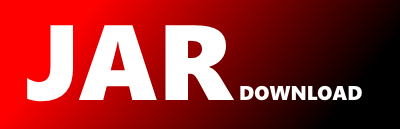
org.ow2.petals.ant.task.monit.ExtractFlowStepInfoTask Maven / Gradle / Ivy
/**
* Copyright (c) 2015-2016 Linagora
*
* This program/library is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 2.1 of the License, or (at your
* option) any later version.
*
* This program/library is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License
* for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program/library; If not, see http://www.gnu.org/licenses/
* for the GNU Lesser General Public License version 2.1.
*/
package org.ow2.petals.ant.task.monit;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import java.util.Random;
import java.util.Set;
import org.apache.tools.ant.Project;
import org.ow2.petals.ant.task.monit.exception.BuildCriteriaSelectionTooLargeException;
import org.ow2.petals.ant.task.monit.exception.BuildExclusifParametersException;
import org.ow2.petals.ant.task.monit.exception.BuildNoFlowStepMatchException;
import org.ow2.petals.ant.task.monit.exception.BuildPropertyMissingException;
import org.ow2.petals.ant.task.monit.exception.BuildRowInvalidException;
import org.ow2.petals.log.parser.api.model.Flow;
import org.ow2.petals.log.parser.api.model.FlowStep;
/**
* The Ant task to extract information from a flow step selected from MONIT traces.
*
* @author Christophe DENEUX - Linagora
*
*/
public class ExtractFlowStepInfoTask extends AbstractMonitFlowsAntTaskRequiringRefId {
private static final Random RANDOM_GENERATOR = new Random();
/**
* Criterias selecting ONE flow step from which information will be extracted
*/
private final List flowStepCriterias = new ArrayList<>();
/**
* If several flow steps match criterias, we select the flow step randomly according to this flag.
*/
private boolean selectRandomly = false;
/**
*
* If several flow steps match criterias, we select the flow step with this row number.
*
*
* First row is '0'. If null
, and several flow steps match criterias and {@link #selectRandomly} is set
* to {@code false}, the error {@link BuildCriteriaSelectionTooLargeException} is thrown. If the value is bigger
* than the number of selected flow steps, the error {@link BuildRowInvalidException} is thrown.
*
*/
private Integer row = null;
/**
* Information to extract from the selected flow step
*/
private final List flowStepInfos = new ArrayList<>();
public void addFlowStepCriteria(final FlowStepCriteria flowStepCriteria) {
this.flowStepCriterias.add(flowStepCriteria);
}
/**
* @param selectRandomly
*/
public void setSelectRandomly(final boolean selectRandomly) {
this.selectRandomly = selectRandomly;
}
/**
* @param row
*/
public void setRow(final int row) {
this.row = Integer.valueOf(row);
}
public void addFlowStepInfo(final FlowStepInfo flowStepInfo) {
this.flowStepInfos.add(flowStepInfo);
}
@Override
public final void doTaskWithFlows(final Set flows) throws Exception {
if (this.row != null && this.selectRandomly) {
throw new BuildExclusifParametersException("selectRandomly", "row", this.getLocation());
}
final List filteredFlowSteps = this.filterFlowSteps(flows);
if (!filteredFlowSteps.isEmpty()) {
final FlowStep selectedFlowStep = this.selectFlowStep(filteredFlowSteps);
this.extractFlowStepInfos(selectedFlowStep);
} else {
// For debug purpose, we print all flow step loaded
final List allFlowSteps = new ArrayList<>();
for (final Flow flow : flows) {
allFlowSteps.addAll(this.getAllFlowSteps(flow.getRoot()));
}
this.getProject().log("All flow steps available in reference '" + this.refId + "'.", Project.MSG_VERBOSE);
for (final FlowStep flowStep : allFlowSteps) {
this.getProject().log("A flow step read: " + flowStep.toString(), Project.MSG_VERBOSE);
}
throw new BuildNoFlowStepMatchException(this.getLocation());
}
}
/**
* Filter flow steps of flows according to flow step criterias
*
* @param flows
* The flows containing flow steps to filter
* @return Filtered flow steps
*/
private List filterFlowSteps(final Set flows) {
// We build an array with all available flow steps of all flow instances
final List allFlowSteps = new ArrayList<>();
for (final Flow flow : flows) {
allFlowSteps.addAll(this.getAllFlowSteps(flow.getRoot()));
}
for (final FlowStepCriteria flowStepCriteria : this.flowStepCriterias) {
this.getProject().log("Executing criteria: " + flowStepCriteria.getName() + " = '"
+ flowStepCriteria.getValue() + "', without \"'\"", Project.MSG_VERBOSE);
final Iterator itAllFlowSteps = allFlowSteps.iterator();
while (itAllFlowSteps.hasNext()) {
final FlowStep flowStep = itAllFlowSteps.next();
if (!flowStepCriteria.getValue()
.equals(flowStep.getBeginProperties().get(flowStepCriteria.getName()))) {
itAllFlowSteps.remove();
}
}
}
// Here 'allFlowSteps' should contain only flow steps matching criterias
return allFlowSteps;
}
/**
* Select a flow steps
*
* @param flowSteps
* A flow step list containing at least one flow step.
* @return the flow step matching selection criterias.
*/
private FlowStep selectFlowStep(final List flowSteps) {
if (flowSteps.size() == 1) {
return flowSteps.get(0);
} else if (this.row == null && !this.selectRandomly) {
throw new BuildCriteriaSelectionTooLargeException(this.getLocation());
} else if (this.row != null && this.row > flowSteps.size() - 1) {
throw new BuildRowInvalidException(this.getLocation());
} else if (this.selectRandomly) {
// We retrieve the flow step randomly (ie. according to parameter 'selectRandomly')
return flowSteps.get(RANDOM_GENERATOR.nextInt(flowSteps.size()));
} else {
// We retrieve the flow step according to parameter 'row'
return flowSteps.get(this.row);
}
}
/**
* Extract flow step information from the given flow step
*
* @param flowStep
* The flow step from which information must be extracted
*/
private void extractFlowStepInfos(final FlowStep flowStep) {
for (final FlowStepInfo flowStepInfo : this.flowStepInfos) {
final String property = flowStepInfo.getProperty();
if (property == null) {
throw new BuildPropertyMissingException(this.getLocation());
} else {
this.getProject().setProperty(property, flowStep.getBeginProperties().get(flowStepInfo.getAttribute()));
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy