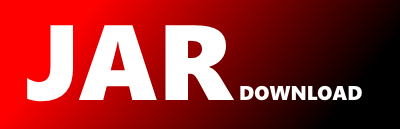
org.ow2.petals.ant.task.monit.FlowTreeNode Maven / Gradle / Ivy
/**
* Copyright (c) 2016 Linagora
*
* This program/library is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 2.1 of the License, or (at your
* option) any later version.
*
* This program/library is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License
* for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program/library; If not, see http://www.gnu.org/licenses/
* for the GNU Lesser General Public License version 2.1.
*/
package org.ow2.petals.ant.task.monit;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.Set;
import org.ow2.petals.log.parser.api.model.Flow;
/**
* A flow tree node
*
* @author Christophe DENEUX - Linagora
*
*/
public class FlowTreeNode {
private final List children = new ArrayList<>();
private final List parents = new ArrayList<>();
private Flow flow;
/**
* If {@code parent} is not {@code null}, this parent is added to the parent list of the current flow tree node, and
* the current flow tree node is automatically added as child of {@code parent}.
*
* @param flow
* @param parent
*/
public FlowTreeNode(final Flow flow, final FlowTreeNode parent) {
this.flow = flow;
if (parent != null) {
parent.addChildFlowTreeNode(this);
this.parents.add(parent);
}
}
public void addChildFlowTreeNode(final FlowTreeNode flowTreeNode) {
this.children.add(flowTreeNode);
}
public void addParentFlowTreeNode(final FlowTreeNode flowTreeNode) {
this.parents.add(flowTreeNode);
}
public void setFlow(final Flow flow) {
this.flow = flow;
}
public Flow getFlow() {
return this.flow;
}
/**
* @return Child flow tree nodes, as unmodifiable {@link Set}.
*/
public List getFlowTreeNodes() {
return Collections.unmodifiableList(this.children);
}
/**
* @return Parent flow tree nodes, as unmodifiable {@link Set}.
*/
public List getParents() {
return Collections.unmodifiableList(this.parents);
}
/**
* @return The child {@link FlowTreeNode} associated to the given flow instance identifier, or {@code null} if not
* found (ie. no flow with the given flow instance identifier exists in the children of the current tree.
*/
public FlowTreeNode getFlowTreeNode(final String flowInstanceId) {
return FlowTreeNode.getFlowTreeNode(this, flowInstanceId);
}
private static FlowTreeNode getFlowTreeNode(final FlowTreeNode flowTreeNode, final String flowInstanceId) {
if (flowTreeNode.getFlow().getFlowId().equals(flowInstanceId)) {
return flowTreeNode;
} else {
for (final FlowTreeNode child : flowTreeNode.getFlowTreeNodes()) {
final FlowTreeNode flowTreeNodeFound = FlowTreeNode.getFlowTreeNode(child, flowInstanceId);
if (flowTreeNodeFound != null) {
return flowTreeNodeFound;
}
}
return null;
}
}
@Override
public String toString() {
return this.toString(new StringBuilder(), 0);
}
private String toString(final StringBuilder buf, final int level) {
if (level > 0) {
buf.append('|');
}
for (int i = 0; i < level; i++) {
buf.append("---");
}
buf.append(String.format(" %s%n", this.flow.getFlowId()));
for (final FlowTreeNode child : this.children) {
child.toString(buf, level + 1);
}
return buf.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy