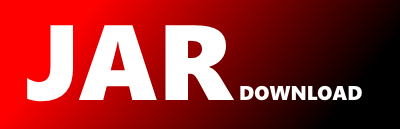
org.ow2.petals.ant.task.monit.ReadLogFilesTask Maven / Gradle / Ivy
/**
* Copyright (c) 2015-2016 Linagora
*
* This program/library is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 2.1 of the License, or (at your
* option) any later version.
*
* This program/library is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License
* for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program/library; If not, see http://www.gnu.org/licenses/
* for the GNU Lesser General Public License version 2.1.
*/
package org.ow2.petals.ant.task.monit;
import java.io.File;
import java.util.ArrayList;
import java.util.List;
import java.util.Set;
import java.util.TreeSet;
import org.apache.tools.ant.BuildException;
import org.apache.tools.ant.DirectoryScanner;
import org.apache.tools.ant.types.DirSet;
import org.ow2.petals.ant.task.monit.exception.BuildDirChooseDirSpecificationException;
import org.ow2.petals.ant.task.monit.exception.BuildDirEmptyException;
import org.ow2.petals.ant.task.monit.exception.BuildDirMissingException;
import org.ow2.petals.ant.task.monit.exception.BuildIdEmptyException;
import org.ow2.petals.ant.task.monit.exception.BuildIdMissingException;
import org.ow2.petals.log.parser.api.FlowBuilder;
import org.ow2.petals.log.parser.api.model.Flow;
import org.ow2.petals.log.parser.api.model.FlowId;
/**
* The Ant task to read MONIT log files
*
* @author Christophe DENEUX - Linagora
*
*/
public class ReadLogFilesTask extends AbstractMonitAntTask {
/**
* Root directory of the log files containing MONIT traces
*/
private String dir;
/**
* Root directories of the log files containing MONIT traces
*/
private final List dirSets = new ArrayList();
/**
* Name of the object reference in which flows will be put
*/
private String id;
/**
* @param dir
*/
public void setDir(final String dir) {
this.dir = dir;
}
public void addDirSet(final DirSet dirSet) {
this.dirSets.add(dirSet);
}
/**
* @param id
*/
public void setId(final String id) {
this.id = id;
}
@Override
public final void doTask() throws Exception {
this.validateIdParameter();
final File dirFile = this.validateDirParameter();
// Parse log files to retrieve flow instances
final FlowBuilder flowBuilder = new FlowBuilder();
final File directories[];
if (this.dirSets.isEmpty()) {
directories = new File[] { dirFile };
} else {
final List dirList = new ArrayList();
for (final DirSet dirSet : this.dirSets) {
final DirectoryScanner scanner = dirSet.getDirectoryScanner(this.getProject());
for (final String includedDir : scanner.getIncludedDirectories()) {
dirList.add(new File(dirSet.getDir(this.getProject()), includedDir));
}
}
directories = dirList.toArray(new File[dirList.size()]);
}
flowBuilder.setLogDirectories(directories);
final Set flows = new TreeSet();
final Set flowIds = flowBuilder.findFlowIds();
this.log(String.format("%d flow(s) found in log files", flowIds.size()));
for (final FlowId flowId : flowIds) {
final Flow flow = flowBuilder.parseFlow(flowId.getName(), this.failOnError);
if (flow != null) {
flows.add(flow);
}
}
this.getProject().addReference(this.id, flows);
}
/**
*
* Validate the parameter 'id
'.
*
*
* The parameter should not be null or empty.
*
*
* @throws BuildException
* The parameter has an invalid value
*/
private void validateIdParameter() throws BuildException {
if (this.id == null) {
throw new BuildIdMissingException(this.getLocation());
}
if (this.id.isEmpty()) {
throw new BuildIdEmptyException(this.getLocation());
}
}
/**
*
* Validate the parameter 'dir
'.
*
*
* The parameter should not be null and must be an existing directory.
*
*
* @throws BuildException
* The parameter has an invalid value
*/
private File validateDirParameter() throws BuildException {
if (this.dir == null && this.dirSets.isEmpty()) {
throw new BuildDirMissingException(this.getLocation());
}
if (this.dir != null && !this.dirSets.isEmpty()) {
throw new BuildDirChooseDirSpecificationException(this.getLocation());
}
if (this.dir != null) {
if (this.dir.isEmpty()) {
throw new BuildDirEmptyException(this.getLocation());
}
final File dirFile = new File(this.dir);
if (!dirFile.exists()) {
throw new BuildException("Invalid value for attribute 'dir': '" + this.dir + "' does not exist.");
}
if (!dirFile.isDirectory()) {
throw new BuildException("Invalid value for attribute 'dir': '" + this.dir + "' is not a directory.");
}
return dirFile;
} else {
return null;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy