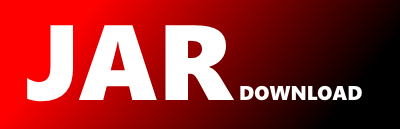
org.ow2.petals.extension.autoloader.ExtensionController Maven / Gradle / Ivy
/**
* Copyright (c) 2013-2016 Linagora
*
* This program/library is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 2.1 of the License, or (at your
* option) any later version.
*
* This program/library is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License
* for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program/library; If not, see http://www.gnu.org/licenses/
* for the GNU Lesser General Public License version 2.1.
*/
package org.ow2.petals.extension.autoloader;
import org.objectweb.fractal.api.Component;
import org.objectweb.fractal.api.Interface;
import org.objectweb.fractal.api.NoSuchInterfaceException;
import org.objectweb.fractal.api.control.BindingController;
import org.objectweb.fractal.api.control.ContentController;
import org.objectweb.fractal.api.control.IllegalBindingException;
import org.objectweb.fractal.api.control.IllegalContentException;
import org.objectweb.fractal.api.control.IllegalLifeCycleException;
import org.objectweb.fractal.api.factory.InstantiationException;
import org.objectweb.fractal.util.Fractal;
import org.ow2.petals.microkernel.api.configuration.ConfigurationService;
import org.ow2.petals.microkernel.api.extension.AbstractPetalsExtensionController;
import org.ow2.petals.microkernel.api.extension.InstallationExtension;
import org.ow2.petals.microkernel.api.extension.PetalsExtensionController;
import org.ow2.petals.microkernel.api.extension.PetalsPostExtensionController;
import org.ow2.petals.microkernel.api.extension.exception.CreationExtensionException;
import org.ow2.petals.microkernel.api.extension.exception.PetalsExtensionException;
import org.ow2.petals.microkernel.api.server.FractalHelper;
/**
* Controller of the Petals post-extension 'Autoloader'
*
* @author Christophe DENEUX - Linagora
*
*/
public class ExtensionController extends AbstractPetalsExtensionController implements PetalsPostExtensionController {
/**
* Name of the property enabling/disabling the auto-loader.
*/
private static final String AUTOLOADER = "petals.autoloader";
/**
* Default value of the property {@value #AUTOLOADER}.
*/
private static final boolean DEFAULT_AUTOLOADER = true;
/**
* The name of the resources containing the Fractal composite definition
* associated to the Petals ESB Autoloader
*/
private static final String AUTOLOADER_COMPOSITE = "Autoloader";
/**
* The name set to the Fractal composite associated to the Petals ESB
* Autoloader
*/
private static final String AUTOLOADER_COMPONENT_NAME = "Autoloader";
/**
* The name of the extension for logging purposes.
*/
private final static String EXTENSION_NAME = "Petals ESB Autoloader";
/**
* The name of the client interface of the configuration service of the
* Petals ESB autoloader composite
*/
private final static String CONFIGURATION_FRACTAL_ITF_NAME = "configuration";
/**
* The name of the client interface of the deployment service of the Petals
* ESB autoloader composite
*/
private final static String DEPLOYMENT_FRACTAL_ITF_NAME = "deployment";
/**
* The name of the client interface of the installation service of the
* Petals ESB autoloader composite
*/
private final static String INSTALLATION_FRACTAL_ITF_NAME = "installation";
/**
* The name of the client interface of the system state service of the
* Petals ESB autoloader composite
*/
private final static String SYSTEMSTATE_FRACTAL_ITF_NAME = "systemstate";
/**
* The name of the client interface of the JMX service of the Petals ESB
* autoloader composite
*/
private final static String JMX_FRACTAL_ITF_NAME = "jmx";
@Override
public Component createFractalComponent(final Component extensionComposite)
throws CreationExtensionException {
try {
// Create the Fractal component associated to the Petals ESB
// Autoloader
final Component extensionImpl = FractalHelper.createCompositeComponent(AUTOLOADER_COMPOSITE);
// Add the Petals ESB Autoloader component in the Extension
// composite
FractalHelper.addComponent(extensionImpl, extensionComposite, null, AUTOLOADER_COMPONENT_NAME);
// Next we bind client interfaces of the Petals ESB Autoloader to
// the right interfaces
final ContentController extensionCC = Fractal.getContentController(extensionComposite);
final BindingController extensionBC = Fractal.getBindingController(extensionImpl);
// As the configuration component is not a sub-component of the
// extension composite, we link the client interface "configuration"
// of the autoloader to the client interface "configuration" of the
// extension composite.
final Interface configurationServiceItf = (Interface) extensionCC
.getFcInternalInterface(PetalsExtensionController.CONFIGURATION_FRACTAL_ITF_NAME);
extensionBC.bindFc(ExtensionController.CONFIGURATION_FRACTAL_ITF_NAME,
configurationServiceItf);
// As the deployment component is not a sub-component of the
// extension composite, we link the client interface "deployment"
// of the autoloader to the client interface "deployment" of the
// extension composite.
final Interface deploymentServiceItf = (Interface) extensionCC
.getFcInternalInterface(PetalsExtensionController.DEPLOYMENT_FRACTAL_ITF_NAME);
extensionBC.bindFc(ExtensionController.DEPLOYMENT_FRACTAL_ITF_NAME,
deploymentServiceItf);
// As the installation component is not a sub-component of the
// extension composite, we link the client interface "installation"
// of the autoloader to the client interface "installation" of the
// extension composite.
final Interface installationServiceItf = (Interface) extensionCC
.getFcInternalInterface(PetalsExtensionController.INSTALLATION_FRACTAL_ITF_NAME);
extensionBC.bindFc(ExtensionController.INSTALLATION_FRACTAL_ITF_NAME,
installationServiceItf);
// As the system state component is not a sub-component of the
// extension composite, we link the client interface "installation"
// of the autoloader to the client interface "installation" of the
// extension composite.
final Interface systemstateServiceItf = (Interface) extensionCC
.getFcInternalInterface(PetalsExtensionController.SYSTEMSTATE_FRACTAL_ITF_NAME);
extensionBC.bindFc(ExtensionController.SYSTEMSTATE_FRACTAL_ITF_NAME,
systemstateServiceItf);
// As the JMX component is not a sub-component of the
// extension composite, we link the client interface "installation"
// of the autoloader to the client interface "installation" of the
// extension composite.
final Interface jmxServiceItf = (Interface) extensionCC
.getFcInternalInterface(PetalsExtensionController.JMX_FRACTAL_ITF_NAME);
extensionBC.bindFc(ExtensionController.JMX_FRACTAL_ITF_NAME, jmxServiceItf);
return extensionImpl;
} catch (final InstantiationException e) {
throw new CreationExtensionException(EXTENSION_NAME, e);
} catch (final NoSuchInterfaceException e) {
throw new CreationExtensionException(EXTENSION_NAME, e);
} catch (final IllegalContentException e) {
throw new CreationExtensionException(EXTENSION_NAME, e);
} catch (final IllegalLifeCycleException e) {
throw new CreationExtensionException(EXTENSION_NAME, e);
} catch (final IllegalBindingException e) {
throw new CreationExtensionException(EXTENSION_NAME, e);
}
}
@Override
public void removeFractalComponent() {
// TODO Auto-generated method stub
}
@Override
public String getExtensionName() {
return ExtensionController.EXTENSION_NAME;
}
@Override
public boolean isActivated(final ConfigurationService configurationService) throws PetalsExtensionException {
final String propertyValue = configurationService.getServerProperties().getProperty(AUTOLOADER);
final boolean isActivated;
if (propertyValue != null) {
isActivated = Boolean.parseBoolean(propertyValue);
} else {
isActivated = DEFAULT_AUTOLOADER;
}
return isActivated;
}
@Override
public String[] getDependencies() {
return null;
}
@Override
public boolean failsPetalsStartup() {
return false;
}
/**
* {@link AutoLoaderServiceImpl} implements {@link InstallationExtension}.
*/
@Override
public boolean isInstallationExtension() {
return true;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy