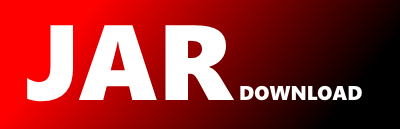
org.ow2.petals.bc.ftp.FTPUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of petals-bc-ftp Show documentation
Show all versions of petals-bc-ftp Show documentation
A binding component used to transfer files using ftp
/**
* Copyright (c) 2007-2012 EBM WebSourcing, 2012-2016 Linagora
*
* This program/library is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 2.1 of the License, or (at your
* option) any later version.
*
* This program/library is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License
* for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program/library; If not, see http://www.gnu.org/licenses/
* for the GNU Lesser General Public License version 2.1.
*/
package org.ow2.petals.bc.ftp;
import java.util.Arrays;
import java.util.List;
import javax.jbi.messaging.Fault;
import javax.jbi.messaging.MessagingException;
import javax.xml.namespace.QName;
import org.ow2.easywsdl.wsdl.api.abstractItf.AbsItfOperation.MEPPatternConstants;
import org.ow2.petals.component.framework.api.message.Exchange;
import org.ow2.petals.component.framework.util.MtomUtil;
import org.ow2.petals.component.framework.util.SourceUtil;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import com.ebmwebsourcing.easycommons.xml.DocumentBuilders;
/**
* @author Adrien LOUIS - EBM WebSourcing
* @author Mathieu CARROLLE - EBM WebSourcing
*/
public class FTPUtil {
/***
* Build a message to indicate the mep available for this operation. TODO :
* Move it in the cdk api.
*
* @param operation
* operation name
* @param mep
* allowed MEP
* @return
*/
public static String getOperationValidMep(String operation, MEPPatternConstants... mep) {
StringBuilder builder = new StringBuilder();
builder.append("Exchange pattern for the " + operation.toUpperCase()
+ " operation must be ");
for (int i = 0; i < mep.length; i++) {
if (i > 0)
builder.append(" or ");
builder.append(mep[i]);
}
return builder.toString();
}
/**
* generates the XML representation of the file name list
*
* @param names
* @return
*/
public static String generateFileNameList(final List names, final QName operation) {
final StringBuffer result = new StringBuffer();
result.append("");
for (final String string : names) {
result.append("");
result.append(string);
result.append(" ");
}
result.append(" ");
return result.toString();
}
/**
* Generate a MTOM response for a list of files.
*
* @param names
* @param operation
* @return
*/
public static Document generateMTOMListResponse(final List names, final QName operation) {
Document doc = DocumentBuilders.newDocument();
Element root = doc.createElementNS(operation.getNamespaceURI(),
"tns:" + operation.getLocalPart() + "Response");
root.appendChild(MtomUtil.generateMtomStructure(doc, names,
new QName(operation.getNamespaceURI(), "attachments", "tns"),
new QName(operation.getNamespaceURI(), "filename", "tns")));
doc.appendChild(root);
return doc;
}
/***
* Generate a MTOM response for a single file.
*
* @param names
* @param operation
* @return
*/
public static Document generateMTOMResponse(String names, QName operation) {
Document doc = DocumentBuilders.newDocument();
Element root = doc.createElementNS(operation.getNamespaceURI(),
"tns:" + operation.getLocalPart() + "Response");
root.appendChild(MtomUtil.generateMtomStructure(doc, Arrays.asList(names), new QName(
operation.getNamespaceURI(), "attachment", "tns"),
new QName(operation.getNamespaceURI(), "filename", "tns")));
doc.appendChild(root);
return doc;
}
public static void setIOFaultOnExchange(Exchange exchange, String ioMessage)
throws MessagingException {
final StringBuffer faultMessage = new StringBuffer();
faultMessage.append("");
faultMessage.append(ioMessage);
faultMessage.append(" ");
Fault fault = exchange.createFault();
fault.setContent(SourceUtil.createSource(faultMessage.toString()));
exchange.setFault(fault);
}
public static void setMissingElementFaultOnExchange(Exchange exchange, String missingElement)
throws MessagingException {
final StringBuffer faultMessage = new StringBuffer();
faultMessage.append("");
faultMessage.append(missingElement);
faultMessage.append(" ");
Fault fault = exchange.createFault();
fault.setContent(SourceUtil.createSource(faultMessage.toString()));
exchange.setFault(fault);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy