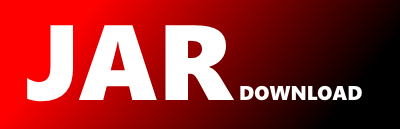
org.ow2.petals.bc.ftp.connection.FTPConnectionInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of petals-bc-ftp Show documentation
Show all versions of petals-bc-ftp Show documentation
A binding component used to transfer files using ftp
/**
* Copyright (c) 2007-2012 EBM WebSourcing, 2012-2016 Linagora
*
* This program/library is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 2.1 of the License, or (at your
* option) any later version.
*
* This program/library is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License
* for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program/library; If not, see http://www.gnu.org/licenses/
* for the GNU Lesser General Public License version 2.1.
*/
package org.ow2.petals.bc.ftp.connection;
import java.nio.charset.Charset;
import org.ow2.petals.bc.ftp.FTPConstants;
import org.ow2.petals.bc.ftp.MissingElementException;
import com.ebmwebsourcing.easycommons.lang.StringHelper;
/**
* @author Mathieu CARROLLE - EBM WebSourcing
* @author Adrien Louis - EBM WebSourcing
*/
public class FTPConnectionInfo {
private int attempt;
private String connectionMode;
private long delay;
private boolean deleteProcessedFile;
private String encoding;
private String folder;
private int maxConnection;
private long maxIdleTime;
private boolean overwrite;
private String password;
private int port;
private String server;
private String transferType;
private String user;
/**
*
* @param server
* @param port
* @param user
* @param password
* @param directory
* can be null
* @param connectionMode
* can be null
* @param transferType
* can be null
* @param attempt
* number of attempts to connect to FTP server
* @param delay
* waiting time before trying to reconnect
* @param deleteProcessedFile
* if true delete each processed file
* @param encoding
* Character encoding used by the FTP control connection.
*
*/
public FTPConnectionInfo(String server, int port, String user, String password,
String directory, String connectionMode, String transferType, int attempt, long delay,
boolean deleteProcessedFile, String encoding, boolean overwrite) {
super();
this.connectionMode = connectionMode;
this.folder = directory;
this.password = password;
this.port = port;
this.server = server;
this.transferType = transferType;
this.user = user;
this.attempt = attempt;
this.delay = delay;
this.deleteProcessedFile = deleteProcessedFile;
this.encoding = encoding;
this.overwrite = overwrite;
}
public int getAttempt() {
return attempt;
}
public String getConnectionMode() {
return connectionMode;
}
public long getDelay() {
return delay;
}
public boolean getDeleteProcessedFile() {
return deleteProcessedFile;
}
public String getDirectory() {
return folder;
}
public String getEncoding() {
return encoding;
}
public int getMaxConnection() {
return maxConnection;
}
public long getMaxIdleTime() {
return maxIdleTime;
}
public String getPassword() {
return password;
}
public int getPort() {
return port;
}
public String getServer() {
return server;
}
public String getTransferType() {
return transferType;
}
public String getUser() {
return user;
}
public boolean isActiveMode() {
return !isPassiveMode();
}
public boolean isAsciiTransferType() {
return FTPConstants.TRANSFER_TYPE_ASCII.equalsIgnoreCase(transferType);
}
public boolean isBinaryTransferType() {
return FTPConstants.TRANSFER_TYPE_BINARY.equalsIgnoreCase(transferType);
}
public boolean isOverwrite() {
return overwrite;
}
public boolean isPassiveMode() {
return FTPConstants.CONNECTION_MODE_PASSIVE.equalsIgnoreCase(connectionMode);
}
public void setAttempt(int attempt) {
this.attempt = attempt;
}
public void setDelay(long delay) {
this.delay = delay;
}
public void setDeleteProcessedFile(boolean deleteProcessedFile) {
this.deleteProcessedFile = deleteProcessedFile;
}
public void setEncoding(String encoding) {
this.encoding = encoding;
}
public void setMaxConnection(int maxConnection) {
this.maxConnection = maxConnection;
}
public void setMaxIdleTime(long maxIdleTime) {
this.maxIdleTime = maxIdleTime;
}
public void setOverwrite(boolean overwrite) {
this.overwrite = overwrite;
}
@Override
public String toString() {
return "FTP Connection Info :server=" + server + "; port=" + port + "; user=" + user
+ "; password=" + password + "; directory=" + folder + "; transfer-type="
+ transferType + "; connection-mode :" + connectionMode + "; encoding :" + encoding
+ "; overwrite :" + overwrite + "; delete processed file :" + deleteProcessedFile;
}
/**
* check attributes
*
* @throws MissingElementException
*/
public void validate() throws MissingElementException {
if (StringHelper.isNullOrEmpty(server))
throw new MissingElementException(FTPConstants.FTP_SERVER);
if (StringHelper.isNullOrEmpty(user))
throw new MissingElementException(FTPConstants.FTP_USER);
if (StringHelper.isNullOrEmpty(password))
throw new MissingElementException(FTPConstants.FTP_PASSWORD);
if (port == 0)
throw new MissingElementException(FTPConstants.FTP_PORT);
if (connectionMode != null
&& !connectionMode.equalsIgnoreCase(FTPConstants.CONNECTION_MODE_ACTIVE)
&& !connectionMode.equalsIgnoreCase(FTPConstants.CONNECTION_MODE_PASSIVE))
throw new MissingElementException(FTPConstants.CONNECTION_MODE);
if (transferType != null
&& !transferType.equalsIgnoreCase(FTPConstants.TRANSFER_TYPE_ASCII)
&& !transferType.equalsIgnoreCase(FTPConstants.TRANSFER_TYPE_BINARY))
throw new MissingElementException(FTPConstants.TRANSFER_TYPE);
if (encoding == null || !Charset.isSupported(encoding)) {
encoding = Charset.defaultCharset().toString();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy