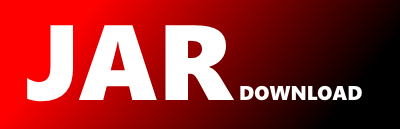
org.ow2.petals.bc.ftp.connection.FTPConnectionInfoBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of petals-bc-ftp Show documentation
Show all versions of petals-bc-ftp Show documentation
A binding component used to transfer files using ftp
/**
* Copyright (c) 2007-2012 EBM WebSourcing, 2012-2016 Linagora
*
* This program/library is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 2.1 of the License, or (at your
* option) any later version.
*
* This program/library is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License
* for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program/library; If not, see http://www.gnu.org/licenses/
* for the GNU Lesser General Public License version 2.1.
*/
package org.ow2.petals.bc.ftp.connection;
import org.ow2.petals.bc.ftp.FTPConstants;
import org.ow2.petals.bc.ftp.MissingElementException;
import org.ow2.petals.component.framework.api.configuration.SuConfigurationParameters;
import org.ow2.petals.component.framework.jbidescriptor.generated.Retrypolicy;
import org.w3c.dom.Node;
import com.ebmwebsourcing.easycommons.xml.XMLHelper;
/**
* @author Mathieu CARROLLE - EBM WebSourcing
* @author Adrien Louis - EBM WebSourcing
*/
public class FTPConnectionInfoBuilder {
public static FTPConnectionInfo buildFTPConnectionInfo(Node node)
throws MissingElementException {
Node server = null;
Node user = null;
Node password = null;
Node directory = null;
Node port = null;
Node connectionMode = null;
Node transferType = null;
Node deleteProcessedFile = null;
Node encoding = null;
Node overwrite = null;
server = XMLHelper.findChild(node, null, FTPConstants.FTP_SERVER, false);
port = XMLHelper.findChild(node, null, FTPConstants.FTP_PORT, false);
user = XMLHelper.findChild(node, null, FTPConstants.FTP_USER, false);
password = XMLHelper.findChild(node, null, FTPConstants.FTP_PASSWORD, false);
directory = XMLHelper.findChild(node, null, FTPConstants.FTP_FOLDER, false);
connectionMode = XMLHelper.findChild(node, null, FTPConstants.CONNECTION_MODE, false);
transferType = XMLHelper.findChild(node, null, FTPConstants.TRANSFER_TYPE, false);
deleteProcessedFile = XMLHelper.findChild(node, null, FTPConstants.FTP_DELETE_PROCESSED_FILES,
false);
encoding = XMLHelper.findChild(node, null, FTPConstants.FTP_ENCODING, false);
overwrite = XMLHelper.findChild(node, null, FTPConstants.FTP_OVERWRITE, false);
int portValue = 21;
if (port != null) {
try {
portValue = Integer.parseInt(port.getTextContent());
} catch (NumberFormatException e) {
portValue = 0;
}
}
FTPConnectionInfo result = new FTPConnectionInfo(server != null ? server.getTextContent()
: null, portValue, user != null ? user.getTextContent() : null,
password != null ? password.getTextContent() : null,
directory != null ? directory.getTextContent() : null,
connectionMode != null ? connectionMode.getTextContent() : null,
transferType != null ? transferType.getTextContent() : null, 1, 0,
deleteProcessedFile != null ? Boolean.parseBoolean(deleteProcessedFile
.getTextContent()) : false, encoding != null ? encoding.getTextContent()
: null,
overwrite != null ? Boolean.parseBoolean(overwrite.getTextContent()) : false);
result.validate();
return result;
}
public static FTPConnectionInfo buildFTPConnectionInfo(final SuConfigurationParameters extensions,
final Retrypolicy retryPolicy) throws MissingElementException {
int attempt = 1;
long delay = 0;
// Get the retry policy elements from the SU descriptor
if (retryPolicy != null) {
attempt += retryPolicy.getAttempts();
delay = retryPolicy.getDelay();
}
String server = null;
String user = null;
String password = null;
String directory = null;
int port = 21;
String connectionMode = null;
String transferType = null;
boolean deleteProcessedFile = false;
String encoding = null;
boolean overwrite = false;
int maxConnection = FTPConstants.DEFAULT_MAX_CONNECTION;
long maxIdleTime = FTPConstants.DEFAULT_MAX_IDLE_TIME;
try {
port = Integer.parseInt(extensions.get(FTPConstants.FTP_PORT));
} catch (NumberFormatException e) {
// managed by validate()
}
try {
maxIdleTime = Long.parseLong(extensions.get(FTPConstants.FTP_MAX_IDLE_TIME));
} catch (NumberFormatException e) {
}
try {
maxConnection = Integer.parseInt(extensions.get(FTPConstants.FTP_MAX_CONNECTION));
} catch (NumberFormatException e) {
}
server = extensions.get(FTPConstants.FTP_SERVER);
user = extensions.get(FTPConstants.FTP_USER);
password = extensions.get(FTPConstants.FTP_PASSWORD);
directory = extensions.get(FTPConstants.FTP_FOLDER);
connectionMode = extensions.get(FTPConstants.CONNECTION_MODE);
transferType = extensions.get(FTPConstants.TRANSFER_TYPE);
deleteProcessedFile = Boolean.valueOf(extensions
.get(FTPConstants.FTP_DELETE_PROCESSED_FILES));
encoding = extensions.get(FTPConstants.FTP_ENCODING);
overwrite = Boolean.parseBoolean(extensions.get(FTPConstants.FTP_OVERWRITE));
FTPConnectionInfo result = new FTPConnectionInfo(server, port, user, password, directory,
connectionMode, transferType, attempt, delay, deleteProcessedFile, encoding,
overwrite);
result.validate();
result.setMaxConnection(maxConnection);
result.setMaxIdleTime(maxIdleTime);
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy