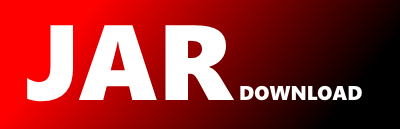
org.ow2.petals.bc.gateway.BcGatewaySUManager Maven / Gradle / Ivy
/**
* Copyright (c) 2015-2016 Linagora
*
* This program/library is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 2.1 of the License, or (at your
* option) any later version.
*
* This program/library is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License
* for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program/library; If not, see http://www.gnu.org/licenses/
* for the GNU Lesser General Public License version 2.1.
*/
package org.ow2.petals.bc.gateway;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Set;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.ConcurrentMap;
import java.util.logging.Level;
import org.eclipse.jdt.annotation.Nullable;
import org.ow2.petals.bc.gateway.inbound.ConsumerDomain;
import org.ow2.petals.bc.gateway.jbidescriptor.generated.JbiConsumerDomain;
import org.ow2.petals.bc.gateway.jbidescriptor.generated.JbiProviderDomain;
import org.ow2.petals.bc.gateway.jbidescriptor.generated.JbiProvidesConfig;
import org.ow2.petals.bc.gateway.outbound.ProviderDomain;
import org.ow2.petals.bc.gateway.utils.BcGatewayJbiHelper;
import org.ow2.petals.bc.gateway.utils.BcGatewayJbiHelper.Pair;
import org.ow2.petals.component.framework.AbstractComponent;
import org.ow2.petals.component.framework.api.exception.PEtALSCDKException;
import org.ow2.petals.component.framework.jbidescriptor.generated.Consumes;
import org.ow2.petals.component.framework.jbidescriptor.generated.Provides;
import org.ow2.petals.component.framework.su.AbstractServiceUnitManager;
import org.ow2.petals.component.framework.su.ServiceUnitDataHandler;
/**
* There is one instance of this class for the whole component.
*
* @author vnoel
*
*/
public class BcGatewaySUManager extends AbstractServiceUnitManager {
private final ConcurrentMap suDatas = new ConcurrentHashMap<>();
public BcGatewaySUManager(final BcGatewayComponent component) {
// let's disable endpoint activation, we will do it ourselves!
super(component, false);
}
/**
* No need for synchronization, only accessed during lifecycles
*/
private static class SUData {
private final Map providerDomains = new HashMap<>();
private final Map consumerDomains = new HashMap<>();
}
public Collection getProviderDomains() {
final List pds = new ArrayList<>();
for (final SUData data : suDatas.values()) {
pds.addAll(data.providerDomains.values());
}
return pds;
}
public @Nullable Map getProviderDomains(final String suName) {
final SUData data = suDatas.get(suName);
if (data != null) {
return Collections.unmodifiableMap(data.providerDomains);
} else {
return null;
}
}
public Collection getConsumerDomains() {
final List cds = new ArrayList<>();
for (final SUData data : suDatas.values()) {
cds.addAll(data.consumerDomains.values());
}
return cds;
}
public @Nullable Map getConsumerDomains(final String suName) {
final SUData data = suDatas.get(suName);
if (data != null) {
return Collections.unmodifiableMap(data.consumerDomains);
} else {
return null;
}
}
/**
* The {@link Provides} must be working after deploy!
*/
@Override
protected void doDeploy(final @Nullable ServiceUnitDataHandler suDH) throws PEtALSCDKException {
assert suDH != null;
final Map>> pd2provides = this.getJPDs(suDH);
final Map> cd2consumes = this.getJCDs(suDH);
final String ownerSU = suDH.getName();
assert ownerSU != null;
final SUData data = new SUData();
this.suDatas.put(ownerSU, data);
for (final Entry> entry : cd2consumes.entrySet()) {
final JbiConsumerDomain jcd = entry.getKey();
assert jcd != null;
final Collection consumes = entry.getValue();
assert consumes != null;
final ConsumerDomain cd = getComponent().createConsumerDomain(suDH, jcd, consumes);
data.consumerDomains.put(jcd.getId(), cd);
}
for (final Entry>> entry : pd2provides
.entrySet()) {
final JbiProviderDomain jpd = entry.getKey();
assert jpd != null;
final Collection> provides = entry.getValue();
assert provides != null;
final ProviderDomain pd = getComponent().createProviderDomain(suDH, jpd, provides);
data.providerDomains.put(jpd.getId(), pd);
}
}
@Override
protected void doInit(final @Nullable ServiceUnitDataHandler suDH) throws PEtALSCDKException {
assert suDH != null;
final SUData data = suDatas.get(suDH.getName());
for (final ProviderDomain pd : data.providerDomains.values()) {
pd.register();
}
}
@Override
protected void doStart(final @Nullable ServiceUnitDataHandler suDH) throws PEtALSCDKException {
assert suDH != null;
final String ownerSU = suDH.getName();
assert ownerSU != null;
final SUData data = suDatas.get(ownerSU);
for (final ConsumerDomain cd : data.consumerDomains.values()) {
cd.open();
}
}
@Override
protected void doStop(final @Nullable ServiceUnitDataHandler suDH) throws PEtALSCDKException {
assert suDH != null;
final String ownerSU = suDH.getName();
assert ownerSU != null;
final SUData data = suDatas.get(ownerSU);
final PEtALSCDKException ex = new PEtALSCDKException("Errors during SU stop");
for (final ConsumerDomain cd : data.consumerDomains.values()) {
try {
cd.close();
} catch (final Exception e) {
ex.addSuppressed(e);
}
}
ex.throwIfNeeded();
}
@Override
protected void doShutdown(final @Nullable ServiceUnitDataHandler suDH) throws PEtALSCDKException {
assert suDH != null;
final SUData data = suDatas.get(suDH.getName());
final PEtALSCDKException ex = new PEtALSCDKException("Errors during SU shutdown");
for (final ProviderDomain pd : data.providerDomains.values()) {
try {
// connection will stay open until undeploy so that previous exchanges are finished
pd.deregister();
} catch (final Exception e) {
ex.addSuppressed(e);
}
}
ex.throwIfNeeded();
}
@Override
protected void doUndeploy(final @Nullable ServiceUnitDataHandler suDH) throws PEtALSCDKException {
assert suDH != null;
final String ownerSU = suDH.getName();
assert ownerSU != null;
final SUData data = suDatas.remove(ownerSU);
if (data == null) {
// can happen in case deploy failed early
return;
}
final PEtALSCDKException ex = new PEtALSCDKException("Errors during SU undeploy");
for (final ProviderDomain pd : data.providerDomains.values()) {
try {
pd.disconnect();
} catch (final Exception e) {
ex.addSuppressed(e);
}
}
for (final ConsumerDomain cd : data.consumerDomains.values()) {
try {
cd.disconnect();
} catch (final Exception e) {
ex.addSuppressed(e);
}
}
ex.throwIfNeeded();
}
@Override
public void onPlaceHolderValuesReloaded() {
for (final ServiceUnitDataHandler suDH : getServiceUnitDataHandlers()) {
assert suDH != null;
try {
// let's first gather data to ensure it is valid
final Set jpds = getJPDs(suDH).keySet();
final Set jcds = getJCDs(suDH).keySet();
final SUData data = suDatas.get(suDH.getName());
for (final JbiProviderDomain jpd : jpds) {
data.providerDomains.get(jpd.getId()).reload(jpd);
}
for (final JbiConsumerDomain jcd : jcds) {
data.consumerDomains.get(jcd.getId()).reload(jcd);
}
} catch (final PEtALSCDKException e) {
logger.log(Level.WARNING, "Can't reload placeholders for SU " + suDH.getName(), e);
}
}
}
private Map> getJCDs(final ServiceUnitDataHandler suDH)
throws PEtALSCDKException {
return BcGatewayJbiHelper.getConsumesPerDomain(suDH, getComponent().getPlaceHolders(), logger);
}
private Map>> getJPDs(
final ServiceUnitDataHandler suDH) throws PEtALSCDKException {
return BcGatewayJbiHelper.getProvidesPerDomain(suDH, getComponent().getPlaceHolders(), logger);
}
@Override
public BcGatewayComponent getComponent() {
final AbstractComponent component = super.getComponent();
assert component != null;
return (BcGatewayComponent) component;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy