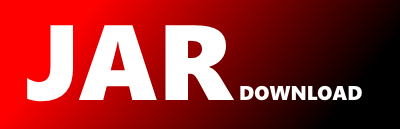
org.ow2.petals.bc.quartz.job.EmitSignalJob Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of petals-bc-quartz Show documentation
Show all versions of petals-bc-quartz Show documentation
A component to schedule service invocations.
/**
* Copyright (c) 2009-2012 EBM WebSourcing, 2012-2017 Linagora
*
* This program/library is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 2.1 of the License, or (at your
* option) any later version.
*
* This program/library is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License
* for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program/library; If not, see http://www.gnu.org/licenses/
* for the GNU Lesser General Public License version 2.1.
*/
package org.ow2.petals.bc.quartz.job;
import java.util.logging.Logger;
import javax.jbi.messaging.MessagingException;
import org.ow2.petals.bc.quartz.QuartzConsumeExtFlowStepBeginLogData;
import org.ow2.petals.bc.quartz.QuartzExternalListener;
import org.ow2.petals.bc.quartz.utils.QuartzConstants;
import org.ow2.petals.bc.quartz.utils.QuartzUtils;
import org.ow2.petals.commons.log.FlowAttributes;
import org.ow2.petals.commons.log.Level;
import org.ow2.petals.commons.log.PetalsExecutionContext;
import org.ow2.petals.component.framework.api.Message.MEPConstants;
import org.ow2.petals.component.framework.api.configuration.ConfigurationExtensions;
import org.ow2.petals.component.framework.api.message.Exchange;
import org.ow2.petals.component.framework.jbidescriptor.generated.Consumes;
import org.ow2.petals.component.framework.logger.StepLogHelper;
import org.ow2.petals.component.framework.util.SourceUtil;
import org.quartz.Job;
import org.quartz.JobDataMap;
import org.quartz.JobExecutionContext;
import org.quartz.JobExecutionException;
/**
* @author Adrien LOUIS - EBM WebSourcing
*/
public class EmitSignalJob implements Job {
@Override
public void execute(final JobExecutionContext jobContext) throws JobExecutionException {
// Get the external listener and the information it contains
final JobDataMap dataMap = jobContext.getJobDetail().getJobDataMap();
final QuartzExternalListener quartzExternalListener = (QuartzExternalListener) dataMap
.get(QuartzConstants.SCHEDULER_MANAGER);
final Consumes consume = quartzExternalListener.getConsumes();
final ConfigurationExtensions extensions = new ConfigurationExtensions(consume.getAny());
final Logger logger = quartzExternalListener.getLogger();
// Invoke the service
final String serviceIdentifier = QuartzUtils.buildTargetServiceIdentifier(consume);
final FlowAttributes flowAttributes = PetalsExecutionContext.initFlowAttributes();
logger.log(Level.MONIT, "", new QuartzConsumeExtFlowStepBeginLogData(flowAttributes.getFlowInstanceId(),
flowAttributes.getFlowStepId(), String.valueOf(jobContext.getFireTime().getTime())));
try {
final Exchange exchange = quartzExternalListener.createConsumeExchange(consume,
MEPConstants.IN_ONLY_PATTERN);
String content = extensions.get(QuartzConstants.CONTENT_EXPRESSION);
if (content != null)
content = content.trim();
if (logger.isLoggable(Level.FINE)) {
logger.fine(String.format("Creating the scheduled message %s for %s.", exchange.getExchangeId(),
serviceIdentifier));
}
if (logger.isLoggable(Level.FINEST))
logger.finest(String.format("%s: the sent content is%n%s", exchange.getExchangeId(), content));
exchange.getInMessage().setContent(SourceUtil.createSource(content));
quartzExternalListener.send(exchange);
if (logger.isLoggable(Level.INFO)) {
logger.info(String.format("The scheduled message %s was successfully sent to %s.",
exchange.getExchangeId(), serviceIdentifier));
}
} catch (final MessagingException e) {
if (logger.isLoggable(Level.INFO))
logger.info(String.format("A scheduled message cound not be sent to %s", serviceIdentifier));
StepLogHelper.addMonitExtFailureTrace(logger, PetalsExecutionContext.getFlowAttributes(), e, true);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy