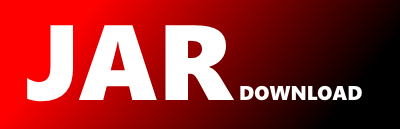
org.ow2.petals.binding.rest.junit.Assert Maven / Gradle / Ivy
Show all versions of petals-bc-rest-junit Show documentation
/**
* Copyright (c) 2017-2020 Linagora
*
* This program/library is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 2.1 of the License, or (at your
* option) any later version.
*
* This program/library is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License
* for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program/library; If not, see http://www.gnu.org/licenses/
* for the GNU Lesser General Public License version 2.1.
*/
package org.ow2.petals.binding.rest.junit;
import static org.junit.Assert.assertEquals;
import static org.junit.Assert.assertFalse;
import static org.junit.Assert.assertNotNull;
import static org.junit.Assert.assertTrue;
import static org.junit.Assert.fail;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.OutputStreamWriter;
import java.io.Reader;
import java.net.URI;
import java.net.URL;
import java.nio.charset.Charset;
import java.nio.charset.StandardCharsets;
import java.util.Map;
import java.util.Properties;
import java.util.logging.Logger;
import java.util.stream.Collectors;
import javax.jbi.messaging.ExchangeStatus;
import javax.jbi.messaging.MessagingException;
import javax.ws.rs.core.MediaType;
import javax.xml.namespace.QName;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.transform.TransformerException;
import org.apache.commons.io.IOUtils;
import org.apache.http.HttpEntity;
import org.apache.http.HttpEntityEnclosingRequest;
import org.apache.http.client.methods.HttpUriRequest;
import org.ow2.easywsdl.extensions.wsdl4complexwsdl.WSDL4ComplexWsdlFactory;
import org.ow2.easywsdl.extensions.wsdl4complexwsdl.api.WSDL4ComplexWsdlReader;
import org.ow2.easywsdl.wsdl.api.Description;
import org.ow2.easywsdl.wsdl.api.InterfaceType;
import org.ow2.easywsdl.wsdl.api.abstractItf.AbsItfOperation.MEPPatternConstants;
import org.ow2.petals.binding.rest.config.RESTProvidesConfiguration;
import org.ow2.petals.binding.rest.exchange.outgoing.reply.FaultReply;
import org.ow2.petals.binding.rest.exchange.outgoing.reply.JBIReply;
import org.ow2.petals.binding.rest.exchange.outgoing.reply.OutReply;
import org.ow2.petals.binding.rest.junit.rule.JUnitRestJBIExchange;
import org.ow2.petals.binding.rest.junit.rule.ServiceOperationUnderTest;
import org.ow2.petals.component.framework.api.Message.MEPConstants;
import org.ow2.petals.component.framework.jbidescriptor.CDKJBIDescriptorBuilder;
import org.ow2.petals.component.framework.jbidescriptor.generated.Jbi;
import org.ow2.petals.component.framework.jbidescriptor.generated.Provides;
import org.ow2.petals.component.framework.util.XMLUtil;
import org.w3c.dom.Document;
import org.xmlunit.builder.DiffBuilder;
import org.xmlunit.builder.Input;
import org.xmlunit.diff.Diff;
import com.ebmwebsourcing.easycommons.stream.EasyByteArrayOutputStream;
import com.ebmwebsourcing.easycommons.xml.DocumentBuilders;
import com.ebmwebsourcing.easycommons.xml.SourceHelper;
import com.ebmwebsourcing.easycommons.xml.XMLHelper;
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.flipkart.zjsonpatch.JsonDiff;
/**
* Assertions about BC Rest service units
*
* @author Christophe DENEUX - Linagora
*
*/
public class Assert {
private static final Logger LOG = Logger.getLogger(Assert.class.getName());
private Assert() {
// Utility class --> no constructor
}
/**
*
* Assertion checking the WSDL compliance of a service unit.
*
*
* This assertion must be used to check the WSDL against JBI descriptor declarations. So this assertion is expected
* to be used as unit test assertion of a service unit project. The JBI descriptor of the SU is expected to be the
* resource 'jbi/jbi.xml'.
*
*/
public static void assertWsdlCompliance() throws Exception {
assertWsdlCompliance(new Properties());
}
public static void assertWsdlCompliance(final Properties componentPlaceholders) throws Exception {
final URL jbiDescriptorUrl = Thread.currentThread().getContextClassLoader().getResource("jbi/jbi.xml");
assertNotNull("SU JBI descriptor not found", jbiDescriptorUrl);
final File jbiDescriptorFile = new File(jbiDescriptorUrl.toURI());
final DocumentBuilder docBuilder = DocumentBuilders.takeDocumentBuilder();
try (final FileInputStream isJbiDescr = new FileInputStream(jbiDescriptorFile)) {
final Jbi jbiDescriptor = CDKJBIDescriptorBuilder.getInstance().buildJavaJBIDescriptor(isJbiDescr);
assertNotNull("Invalid JBI descriptor", jbiDescriptor);
assertNotNull("Invalid JBI descriptor", jbiDescriptor.getServices());
assertNotNull("Invalid JBI descriptor", jbiDescriptor.getServices().getProvides());
assertEquals("Invalid JBI descriptor", 1, jbiDescriptor.getServices().getProvides().size());
final String installRoot = new File(jbiDescriptorUrl.toURI()).getParentFile().getAbsolutePath();
for (final Provides provides : jbiDescriptor.getServices().getProvides()) {
final File wsdlFile = new File(installRoot, provides.getWsdl());
final Document wsdlDoc = docBuilder.parse(wsdlFile);
final RESTProvidesConfiguration providesConfig = new RESTProvidesConfiguration(LOG, wsdlDoc,
"service-unit-under-test", installRoot, provides, componentPlaceholders);
// Check that all operations defined in JBI descriptor exist in the WSDL
final WSDL4ComplexWsdlReader wsdlReader = WSDL4ComplexWsdlFactory.newInstance().newWSDLReader();
final Description wsdlDescr = wsdlReader.read(wsdlFile.toURI().toURL());
assertNotNull(wsdlDescr);
final InterfaceType itf = wsdlDescr.getInterface(provides.getInterfaceName());
assertNotNull(String.format("Interface '%s' not found in WSDL '%s'",
provides.getInterfaceName().toString(), provides.getWsdl()), itf);
for (final QName opJbi : providesConfig.getRESTRequests().keySet()) {
assertNotNull(String.format("Operation '%s' not found in WSDL '%s'", opJbi.toString(),
provides.getWsdl()), itf.getOperation(opJbi));
}
}
} finally {
DocumentBuilders.releaseDocumentBuilder(docBuilder);
}
}
/**
*
* Assertion about an expected JBI OUT message payload generated from the given REST response:
*
*
* - HTTP status,
* - content-type,
* - HTTP body.
*
*
* @param expectedXMLPayloadResourceName
* Name of the resource containing the expected JBI OUT message payload
* @param opUnderTest
* The operation under test
* @param httpStatus
* The HTTP status of the REST response
* @param httpReason
* The HTTP reason of the REST response
* @param httpBodyResourceName
* Name of the resource containing the HTTP body of the REST response
* @param httpBodyContentType
* The content type of the REST response
* @param uriParameterValues
* Values of URI parameters. {@code null} if URI parameters are not use by OUT transformation.
*/
public static void assertJBIOutResponse(final String expectedXMLPayloadResourceName,
final ServiceOperationUnderTest opUnderTest, final int httpStatus, final String httpReason,
final String httpBodyResourceName, final MediaType httpBodyContentType,
final Map uriParameterValues) throws Exception {
// As a JBI OUT response is expected, the exchange pattern is InOut
assertJBIResponse(expectedXMLPayloadResourceName, ExchangeStatus.ACTIVE, opUnderTest,
MEPConstants.IN_OUT_PATTERN, httpStatus,
httpReason, httpBodyResourceName, httpBodyContentType, null, uriParameterValues);
}
/**
*
* Assertion about an expected JBI InOnly/RobustInOnly status message generated from the given REST response
*
*
* @param expectedStatus
* Expected JBI Status
* @param opUnderTest
* The operation under test
* @param mep
* MEP of the JBI exchange in which the assertion takes place. Only MEP 'InOnlyt' and 'RobustInOnly' are
* accepted.
* @param httpStatus
* The HTTP status of the REST response
* @param httpReason
* The HTTP reason of the REST response
* @param httpBodyContentType
* The content type of the REST response
* @param uriParameterValues
* Values of URI parameters. {@code null} if URI parameters are not use by OUT transformation.
*/
public static void assertJBIStatus(final ExchangeStatus expectedStatus, final ServiceOperationUnderTest opUnderTest,
final MEPPatternConstants mep, final int httpStatus, final String httpReason,
final MediaType httpBodyContentType, final Map uriParameterValues) throws Exception {
assertJBIStatus(expectedStatus, opUnderTest, mep, httpStatus, httpReason, null, httpBodyContentType, null,
uriParameterValues);
}
/**
*
* Assertion about an expected JBI InOnly/RobustInOnly status message generated from the given REST response
*
*
* @param opUnderTest
* The operation under test
* @param httpStatus
* The HTTP status of the REST response
* @param httpReason
* The HTTP reason of the REST response
* @param httpBodyResourceName
* Name of the resource containing the HTTP body of the REST response. {@code null} for no HTTP body.
* @param httpBodyContentType
* The content type of the REST response. {@code null} if no HTTP body.
* @param inXMLPayloadResourceName
* Name of the resource containing the JBI IN message payload from which the fault can be generated
* @param uriParameterValues
* Values of URI parameters. {@code null} if URI parameters are not use by fault transformation.
*/
public static void assertJBIStatus(final ExchangeStatus expectedStatus, final ServiceOperationUnderTest opUnderTest,
final MEPPatternConstants mep, final int httpStatus, final String httpReason,
final String httpBodyResourceName, final MediaType httpBodyContentType,
final String inXMLPayloadResourceName, final Map uriParameterValues) throws Exception {
assert mep == MEPConstants.IN_ONLY_PATTERN || mep == MEPConstants.ROBUST_IN_ONLY_PATTERN;
// As a JBI status is expected
assertJBIResponse(null, expectedStatus, opUnderTest, mep, httpStatus, httpReason, httpBodyResourceName,
httpBodyContentType, inXMLPayloadResourceName, uriParameterValues);
}
/**
*
* Assertion about an expected JBI fault payload generated from the given REST response:
*
*
* - HTTP status,
* - empty HTTP body.
*
*
* @param expectedXMLPayloadResourceName
* Name of the resource containing the expected JBI Fault message payload
* @param opUnderTest
* The operation under test
* @param httpStatus
* The HTTP status of the REST response
* @param httpReason
* The HTTP reason of the REST response
* @param httpBodyResourceName
* Name of the resource containing the HTTP body of the REST response. {@code null} for no HTTP body.
* @param httpBodyContentType
* The content type of the REST response. {@code null} if no HTTP body.
* @param inXMLPayloadResourceName
* Name of the resource containing the JBI IN message payload from which the fault can be generated
* @param uriParameterValues
* Values of URI parameters. {@code null} if URI parameters are not use by fault transformation.
*/
public static void assertJBIFault(final String expectedXMLPayloadResourceName,
final ServiceOperationUnderTest opUnderTest, final int httpStatus, final String httpReason,
final String httpBodyResourceName, final MediaType httpBodyContentType,
final String inXMLPayloadResourceName, final Map uriParameterValues) throws Exception {
assertJBIFault(expectedXMLPayloadResourceName, opUnderTest, MEPConstants.IN_OUT_PATTERN, httpStatus, httpReason,
httpBodyResourceName, httpBodyContentType, inXMLPayloadResourceName, uriParameterValues);
}
/**
*
* Assertion about an expected JBI fault payload generated from the given REST response:
*
*
* - HTTP status,
* - empty HTTP body.
*
*
* @param expectedXMLPayloadResourceName
* Name of the resource containing the expected JBI Fault message payload
* @param opUnderTest
* The operation under test
* @param mep
* MEP of the JBI exchange in which the assertion takes place. Only MEP 'InOut' and 'RobustInOnly' are
* accepted.
* @param httpStatus
* The HTTP status of the REST response
* @param httpReason
* The HTTP reason of the REST response
* @param httpBodyResourceName
* Name of the resource containing the HTTP body of the REST response. {@code null} for no HTTP body.
* @param httpBodyContentType
* The content type of the REST response. {@code null} if no HTTP body.
* @param inXMLPayloadResourceName
* Name of the resource containing the JBI IN message payload from which the fault can be generated
* @param uriParameterValues
* Values of URI parameters. {@code null} if URI parameters are not use by fault transformation.
*/
public static void assertJBIFault(final String expectedXMLPayloadResourceName,
final ServiceOperationUnderTest opUnderTest, final MEPPatternConstants mep, final int httpStatus,
final String httpReason, final String httpBodyResourceName, final MediaType httpBodyContentType,
final String inXMLPayloadResourceName, final Map uriParameterValues) throws Exception {
assert mep == MEPConstants.IN_OUT_PATTERN || mep == MEPConstants.ROBUST_IN_ONLY_PATTERN;
assertJBIResponse(expectedXMLPayloadResourceName, ExchangeStatus.ACTIVE, opUnderTest, mep, httpStatus,
httpReason, httpBodyResourceName, httpBodyContentType, inXMLPayloadResourceName, uriParameterValues);
}
/**
*
* Assertion about an expected JBI error generated from the given REST response:
*
*
* - HTTP status,
* - empty HTTP body.
*
*
* @param expectedErrorMessage
* The expected error message
* @param opUnderTest
* The operation under test
* @param mep
* MEP of the JBI exchange in which the assertion takes place. Only MEP 'InOut' and 'RobustInOnly' are
* accepted.
* @param httpStatus
* The HTTP status of the REST response
* @param httpReason
* The HTTP reason of the REST response
* @param httpBodyResourceName
* Name of the resource containing the HTTP body of the REST response. {@code null} for no HTTP body.
* @param httpBodyContentType
* The content type of the REST response. {@code null} if no HTTP body.
* @param inXMLPayloadResourceName
* Name of the resource containing the JBI IN message payload from which the fault can be generated
* @param uriParameterValues
* Values of URI parameters. {@code null} if URI parameters are not use by fault transformation.
*/
public static void assertJBIError(final String expectedErrorMessage, final ServiceOperationUnderTest opUnderTest,
final MEPPatternConstants mep, final int httpStatus, final String httpReason,
final String httpBodyResourceName, final MediaType httpBodyContentType,
final String inXMLPayloadResourceName, final Map uriParameterValues) throws Exception {
try {
assertJBIResponse(null, ExchangeStatus.ERROR, opUnderTest, mep, httpStatus, httpReason,
httpBodyResourceName, httpBodyContentType, inXMLPayloadResourceName, uriParameterValues);
} catch (final AssertionError e) {
throw e;
} catch (final Exception e) {
assertEquals("Unexpected error", expectedErrorMessage, e.getMessage());
}
}
/**
* Assertion to validate a HTTP request with HTTP body as XML.
*/
public static void assertJBIRequestXML(final String expectedXMLPayloadResourceName,
final ServiceOperationUnderTest opUnderTest, final MEPPatternConstants mep,
final String inXMLPayloadResourceName, final Map uriParameterValues) throws Exception {
assertJBIRequestXML(null, expectedXMLPayloadResourceName, opUnderTest, mep, inXMLPayloadResourceName,
uriParameterValues);
}
/**
* Assertion to validate a HTTP request with HTTP body as XML.
*/
public static void assertJBIRequestXML(final URI expectedURI, final String expectedXMLPayloadResourceName,
final ServiceOperationUnderTest opUnderTest, final MEPPatternConstants mep,
final String inXMLPayloadResourceName, final Map uriParameterValues) throws Exception {
assertJBIRequest(expectedURI, expectedXMLPayloadResourceName, MediaType.APPLICATION_XML_TYPE, opUnderTest, mep,
inXMLPayloadResourceName, uriParameterValues);
}
/**
* Assertion to validate a HTTP request with HTTP body as JSON.
*/
public static void assertJBIRequestJSON(final String expectedJSONPayloadResourceName,
final ServiceOperationUnderTest opUnderTest, final MEPPatternConstants mep,
final String inXMLPayloadResourceName, final Map uriParameterValues) throws Exception {
assertJBIRequestJSON(null, expectedJSONPayloadResourceName, opUnderTest, mep, inXMLPayloadResourceName,
uriParameterValues);
}
/**
* Assertion to validate a HTTP request with HTTP body as JSON.
*/
public static void assertJBIRequestJSON(final URI expectedURI, final String expectedJSONPayloadResourceName,
final ServiceOperationUnderTest opUnderTest, final MEPPatternConstants mep,
final String inXMLPayloadResourceName, final Map uriParameterValues) throws Exception {
assertJBIRequest(expectedURI, expectedJSONPayloadResourceName, MediaType.APPLICATION_JSON_TYPE, opUnderTest,
mep, inXMLPayloadResourceName, uriParameterValues);
}
/**
* Assertion to validate a HTTP request with no HTTP body.
*/
public static void assertJBIRequestEmpty(final ServiceOperationUnderTest opUnderTest, final MEPPatternConstants mep,
final String inXMLPayloadResourceName, final Map uriParameterValues) throws Exception {
assertJBIRequestEmpty(null, opUnderTest, mep, inXMLPayloadResourceName, uriParameterValues);
}
/**
* Assertion to validate a HTTP request with no HTTP body.
*/
public static void assertJBIRequestEmpty(final URI expectedURI, final ServiceOperationUnderTest opUnderTest,
final MEPPatternConstants mep, final String inXMLPayloadResourceName,
final Map uriParameterValues) throws Exception {
assertJBIRequest(expectedURI, null, null, opUnderTest, mep, inXMLPayloadResourceName, uriParameterValues);
}
/**
* Assertion to validate a HTTP request with HTTP body as URL-encoded form data.
*/
public static void assertJBIRequestForm(final String expectedHttpBodyResourceName,
final ServiceOperationUnderTest opUnderTest, final MEPPatternConstants mep,
final String inXMLPayloadResourceName, final Map uriParameterValues) throws Exception {
assertJBIRequestForm(null, expectedHttpBodyResourceName, opUnderTest, mep, inXMLPayloadResourceName,
uriParameterValues);
}
/**
* Assertion to validate a HTTP request with HTTP body as URL-encoded form data.
*/
public static void assertJBIRequestForm(final URI expectedURI, final String expectedHttpBodyResourceName,
final ServiceOperationUnderTest opUnderTest, final MEPPatternConstants mep,
final String inXMLPayloadResourceName, final Map uriParameterValues) throws Exception {
assertJBIRequest(expectedURI, expectedHttpBodyResourceName, MediaType.APPLICATION_FORM_URLENCODED_TYPE,
opUnderTest, mep, inXMLPayloadResourceName, uriParameterValues);
}
// TODO: URI parameter values should be build from the operation definition and the incoming XML payload
private static void assertJBIRequest(final URI expectedURI, final String expectedHttpBodyResourceName,
final MediaType expectedContentType, final ServiceOperationUnderTest opUnderTest,
final MEPPatternConstants mep, final String inXMLPayloadResourceName,
final Map uriParameterValues) throws Exception {
assertNotNull("Operation under test is null", opUnderTest);
assertTrue("'expectedHttpBodyResourceName' and 'expectedContentType' must both be null or not null",
!(expectedHttpBodyResourceName == null ^ expectedContentType == null));
final JUnitRestJBIExchange jbiExchange;
try (final InputStream inXMLPayloadResourceStream = Thread.currentThread().getContextClassLoader()
.getResourceAsStream(inXMLPayloadResourceName)) {
assertNotNull("XML resource file '" + inXMLPayloadResourceName
+ "' containing the XML input message is not found", inXMLPayloadResourceStream);
jbiExchange = new JUnitRestJBIExchange(XMLUtil.loadDocument(inXMLPayloadResourceStream), mep.value(),
uriParameterValues);
}
final HttpUriRequest request = opUnderTest.transformRESTRequest(jbiExchange);
if (expectedURI != null) {
assertEquals(expectedURI, request.getURI());
}
if (expectedHttpBodyResourceName != null) {
assertTrue("The built request should have a body", request instanceof HttpEntityEnclosingRequest
&& ((HttpEntityEnclosingRequest) request).getEntity() != null);
final HttpEntity entity = ((HttpEntityEnclosingRequest) request).getEntity();
final String body;
if (entity.isStreaming()) {
final EasyByteArrayOutputStream ebaos = new EasyByteArrayOutputStream();
entity.writeTo(ebaos);
body = ebaos.toString();
} else {
body = IOUtils.toString(entity.getContent(), Charset.defaultCharset());
}
try (final InputStream expectedResultStream = Thread.currentThread().getContextClassLoader()
.getResourceAsStream(expectedHttpBodyResourceName)) {
assertNotNull("HTTP body resource file '" + expectedHttpBodyResourceName
+ "' containing the transformation result is not found", expectedResultStream);
if (MediaType.APPLICATION_XML_TYPE.isCompatible(expectedContentType)) {
final Diff diff = DiffBuilder.compare(Input.fromStream(expectedResultStream))
.withTest(Input.fromString(body)).checkForSimilar().ignoreComments().ignoreWhitespace()
.build();
assertFalse(String.format("Unexpected XML payload: %n%s%nDifferences:%n%s", body, diff.toString()),
diff.hasDifferences());
} else if (MediaType.APPLICATION_JSON_TYPE.isCompatible(expectedContentType)) {
// We remove potential comments that can be included in the resource name but are forbidden in JSON
final EasyByteArrayOutputStream baos = new EasyByteArrayOutputStream();
try (final OutputStreamWriter osw = new OutputStreamWriter(baos);
final BufferedReader jsonFileBufferedReader = new BufferedReader(
new InputStreamReader(expectedResultStream));) {
String line;
while ((line = jsonFileBufferedReader.readLine()) != null) {
if (!line.startsWith("//")) {
osw.write(line);
osw.write('\n');
}
}
}
final ObjectMapper mapper = new ObjectMapper();
final JsonNode control = mapper.readTree(baos.toByteArrayInputStream());
final JsonNode test = mapper.readTree(body);
final JsonNode diff = JsonDiff.asJson(control, test);
assertTrue(String.format("Unexpected JSON payload: %n%s%nDifferences:%n%s", body, diff.toString()),
diff.equals(mapper.createArrayNode()));
} else if (MediaType.APPLICATION_FORM_URLENCODED_TYPE.isCompatible(expectedContentType)) {
try (final Reader expectedResultReader = new InputStreamReader(expectedResultStream,
StandardCharsets.UTF_8)) {
final String expectedBody = new BufferedReader(expectedResultReader).lines()
.collect(Collectors.joining("\n"));
assertEquals(String.format("Unexpected Text payload: %n%s%nExpected:%n%s", body, expectedBody),
expectedBody, body);
}
} else {
fail("Unsupported expected content type: " + expectedContentType);
}
}
} else {
assertTrue("The built request should not have a body",
(request instanceof HttpEntityEnclosingRequest
&& (((HttpEntityEnclosingRequest) request).getEntity() == null
|| ((HttpEntityEnclosingRequest) request).getEntity().getContentLength() == 0))
|| !(request instanceof HttpEntityEnclosingRequest));
}
}
/**
*
* Assertion about an expected JBI response generated from the given REST response:
*
*
* - HTTP status,
* - content-type,
* - HTTP body.
*
*
* @param expectedXMLPayloadResourceName
* Name of the resource containing the expected JBI XML payload (OUT or fault). Can be {@code null}.
* @param expectedStatus
* An expected status. Not {@code null}.
* @param opUnderTest
* The operation under test
* @param mep
* The JBI exchange pattern
* @param httpStatus
* The HTTP status of the REST response
* @param httpBodyResourceName
* Name of the resource containing the HTTP body of the REST response. Use {@code null} for no HTTP body.
* @param httpBodyContentType
* The content type of the REST response. {@code null} if no HTTP body.
* @param inXMLPayloadResourceName
* Name of the resource containing the JBI IN message payload from which XML response can be generated
* @param uriParameterValues
* Values of URI parameters. Can be {@code null} if not used in transformation.
*/
// TODO: URI parameter values should be build from the operation definition and the incoming XML payload
private static void assertJBIResponse(final String expectedXMLPayloadResourceName,
final ExchangeStatus expectedStatus, final ServiceOperationUnderTest opUnderTest,
final MEPPatternConstants mep, final int httpStatus, final String httpReason,
final String httpBodyResourceName, final MediaType httpBodyContentType,
final String inXMLPayloadResourceName, final Map uriParameterValues) throws Exception {
assertNotNull("Operation under test is null", opUnderTest);
assertTrue("'expectedStatus' is an expected parameter", expectedStatus != null);
final JUnitRestJBIExchange jbiExchange;
if (inXMLPayloadResourceName != null) {
try (final InputStream inXMLPayloadResourceStream = Thread.currentThread().getContextClassLoader()
.getResourceAsStream(inXMLPayloadResourceName)) {
assertNotNull("XML resource file '" + inXMLPayloadResourceName
+ "' containing the XML input message is not found", inXMLPayloadResourceStream);
jbiExchange = new JUnitRestJBIExchange(XMLUtil.loadDocument(inXMLPayloadResourceStream), mep.value(),
uriParameterValues);
}
} else {
jbiExchange = new JUnitRestJBIExchange(mep.value(), uriParameterValues);
}
final JBIReply jbiReply = opUnderTest.transformRESTResponse(jbiExchange, httpStatus, httpReason,
httpBodyResourceName, httpBodyContentType);
assertNotNull("Actual JBI response is null", jbiReply);
jbiReply.setReplyInJBIExchange(jbiExchange);
if (jbiExchange.isInOnlyPattern()) {
// Here: We check the status returned of a MEP InOnly
assertNotNull("No expected status given for a MEP InOnly", expectedStatus);
assertTrue(expectedStatus == ExchangeStatus.DONE && jbiExchange.isDoneStatus());
} else if (jbiExchange.isRobustInOnlyPattern()) {
assertTrue((expectedStatus == ExchangeStatus.DONE && jbiExchange.isDoneStatus())
|| (expectedStatus == ExchangeStatus.ERROR && jbiExchange.isErrorStatus())
|| (expectedStatus == ExchangeStatus.ACTIVE && jbiExchange.isActiveStatus()));
if (jbiExchange.isErrorStatus()) {
assertTrue(!jbiExchange.isFaultMessage() && !jbiExchange.isOutMessage());
final Exception error = jbiExchange.getError();
assertNotNull("No error returned", error);
throw error;
} else if (jbiExchange.isActiveStatus()) {
// Fault returned
assertTrue(jbiExchange.isFaultMessage() && !jbiExchange.isOutMessage());
assertOutOrFault(jbiExchange, jbiReply, expectedXMLPayloadResourceName);
} else {
// Status DONE returned
assertTrue(expectedStatus == ExchangeStatus.DONE);
assertTrue(!jbiExchange.isFaultMessage() && !jbiExchange.isOutMessage());
}
} else {
assertTrue((expectedStatus == ExchangeStatus.ACTIVE && jbiExchange.isActiveStatus())
|| (expectedStatus == ExchangeStatus.ERROR && jbiExchange.isErrorStatus()));
if (jbiExchange.isErrorStatus()) {
assertTrue(!jbiExchange.isFaultMessage() && !jbiExchange.isOutMessage());
final Exception error = jbiExchange.getError();
assertNotNull("No error returned", error);
throw error;
} else {
// Here: We check the OUT message or fault of a MEP InOut
assertTrue(jbiExchange.isActiveStatus());
assertTrue(jbiExchange.isFaultMessage() ^ jbiExchange.isOutMessage());
assertOutOrFault(jbiExchange, jbiReply, expectedXMLPayloadResourceName);
}
}
}
private static void assertOutOrFault(final JUnitRestJBIExchange jbiExchange, final JBIReply jbiReply,
final String expectedXMLPayloadResourceName) throws MessagingException, TransformerException, IOException {
assertFalse(jbiExchange.isDoneStatus());
final Document payload;
if (jbiExchange.getOutMessageContentAsDocument() != null) {
assertTrue("No OUT payload returned", jbiReply instanceof OutReply);
payload = jbiExchange.getOutMessageContentAsDocument();
} else {
assertTrue("No Fault payload returned", jbiReply instanceof FaultReply);
payload = SourceHelper.toDocument(jbiExchange.getFault().getContent());
}
assertNotNull(payload);
assertNotNull("A resource is required for the expected XML payload", expectedXMLPayloadResourceName);
try (final InputStream expectedResultStream = Thread.currentThread().getContextClassLoader()
.getResourceAsStream(expectedXMLPayloadResourceName)) {
assertNotNull("XML resource file '" + expectedXMLPayloadResourceName
+ "' containing the XML transformation result is not found", expectedResultStream);
// TODO: Add an assertion about the compliance of the expected result against the WSDL
final Diff diff = DiffBuilder.compare(Input.fromStream(expectedResultStream))
.withTest(Input.fromDocument(payload)).checkForSimilar().ignoreComments().ignoreWhitespace()
.build();
assertFalse(String.format("Unexpected XML payload: %n%s%nDifferences:%n%s",
XMLHelper.createStringFromDOMDocument(payload), diff.toString()), diff.hasDifferences());
}
}
}