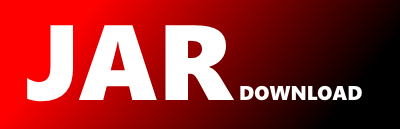
org.ow2.petals.binding.rest.RESTSUManager Maven / Gradle / Ivy
/**
* Copyright (c) 2007-2012 EBM WebSourcing, 2012-2020 Linagora
*
* This program/library is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 2.1 of the License, or (at your
* option) any later version.
*
* This program/library is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License
* for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program/library; If not, see http://www.gnu.org/licenses/
* for the GNU Lesser General Public License version 2.1.
*/
package org.ow2.petals.binding.rest;
import java.util.logging.Level;
import javax.xml.namespace.QName;
import javax.xml.xpath.XPath;
import javax.xml.xpath.XPathFactory;
import org.ow2.petals.binding.rest.config.RESTConsumesConfiguration;
import org.ow2.petals.binding.rest.config.RESTProvidesConfiguration;
import org.ow2.petals.binding.rest.exchange.incoming.RESTExternalListener;
import org.ow2.petals.component.framework.api.exception.PEtALSCDKException;
import org.ow2.petals.component.framework.bc.BindingComponentServiceUnitManager;
import org.ow2.petals.component.framework.jbidescriptor.generated.Consumes;
import org.ow2.petals.component.framework.jbidescriptor.generated.Jbi;
import org.ow2.petals.component.framework.jbidescriptor.generated.Provides;
import org.ow2.petals.component.framework.jbidescriptor.generated.Services;
import org.ow2.petals.component.framework.listener.AbstractExternalListener;
import org.ow2.petals.component.framework.su.ServiceUnitDataHandler;
public class RESTSUManager extends BindingComponentServiceUnitManager {
private RESTComponent restComponent;
public RESTSUManager(final RESTComponent restComponent) {
super(restComponent);
this.restComponent = restComponent;
}
@Override
protected AbstractExternalListener createExternalListener() {
return new RESTExternalListener();
}
@Override
protected void doDeploy(final ServiceUnitDataHandler suDH) throws PEtALSCDKException {
if (this.logger.isLoggable(Level.FINEST)) {
this.logger.log(Level.FINEST, String.format("Deploy '%s'", suDH.getName()));
}
final Services services = suDH.getDescriptor().getServices();
for (final Consumes consumes : services.getConsumes()) {
final String endpointName = consumes.getEndpointName();
final QName interfaceName = consumes.getInterfaceName();
final QName serviceName = consumes.getServiceName();
if (this.logger.isLoggable(Level.FINEST)) {
this.logger.log(Level.FINEST, String.format(
"Consumer:%n\tEndpoint Name '%s'%n\tInterface Name '%s'%n\tService Name '%s'",
endpointName, interfaceName, serviceName));
}
final XPathFactory xpathFactory = XPathFactory.newInstance();
final XPath xPathEvaluator = xpathFactory.newXPath();
final RESTConsumesConfiguration consumesConfig = new RESTConsumesConfiguration(suDH, consumes,
xPathEvaluator, this.getComponent().getPlaceHolders(), this.logger);
this.restComponent.addConsumesConfig(consumes, consumesConfig);
this.restComponent.addRESTService(consumes);
}
for (final Provides provides : services.getProvides()) {
final String endpointName = provides.getEndpointName();
final QName interfaceName = provides.getInterfaceName();
final QName serviceName = provides.getServiceName();
if (this.logger.isLoggable(Level.FINEST)) {
this.logger.log(Level.FINEST, String.format(
"Provider:%n\tEndpoint Name '%s'%n\tInterface Name '%s'%n\tService Name '%s'",
endpointName, interfaceName, serviceName));
}
final RESTProvidesConfiguration providesConfig = new RESTProvidesConfiguration(this.logger,
suDH.getEndpointDescription(provides), suDH.getName(), suDH.getInstallRoot(), provides,
this.getComponent().getPlaceHolders());
this.restComponent.addProvidesConfig(provides, providesConfig);
}
}
@Override
protected void doUndeploy(final ServiceUnitDataHandler suDH) throws PEtALSCDKException {
if (this.logger.isLoggable(Level.FINEST)) {
this.logger.log(Level.FINEST, String.format("Undeploy '%s'", suDH.getName()));
}
final Jbi jbiDescriptor = suDH.getDescriptor();
final Services services = jbiDescriptor.getServices();
final PEtALSCDKException ex = new PEtALSCDKException("Error during undeploy");
for (final Consumes consumes : services.getConsumes()) {
if (this.restComponent.getConsumesConfig(consumes) != null) {
try {
this.restComponent.removeRESTService(consumes);
this.restComponent.removeConsumesConfig(consumes);
} catch (final PEtALSCDKException e) {
ex.addSuppressed(e);
}
}
}
for (final Provides provides : services.getProvides()) {
if (this.restComponent.getProvidesConfig(provides) != null) {
this.restComponent.removeProvidesConfig(provides);
}
}
ex.throwIfNeeded();
}
@Override
protected void onPlaceHolderValuesReloaded() {
super.onPlaceHolderValuesReloaded();
for (final RESTProvidesConfiguration provides : this.restComponent.getProvidesConfigs().values()) {
provides.onPlaceHolderValuesReloaded();
}
for (final RESTConsumesConfiguration consumes : this.restComponent.getConsumesConfigs().values()) {
consumes.onPlaceHolderValuesReloaded();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy