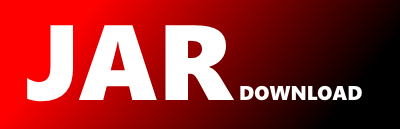
org.ow2.petals.binding.rest.utils.HttpHeadersBuilder Maven / Gradle / Ivy
/**
* Copyright (c) 2018-2020 Linagora
*
* This program/library is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 2.1 of the License, or (at your
* option) any later version.
*
* This program/library is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License
* for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program/library; If not, see http://www.gnu.org/licenses/
* for the GNU Lesser General Public License version 2.1.
*/
package org.ow2.petals.binding.rest.utils;
import java.util.HashMap;
import java.util.Map;
import java.util.Properties;
import java.util.logging.Level;
import java.util.logging.Logger;
import javax.xml.xpath.XPath;
import org.ow2.petals.binding.rest.utils.extractor.value.ValueExtractorBuilder;
import org.ow2.petals.binding.rest.utils.extractor.value.XMLPayloadValueExtractor;
import org.ow2.petals.binding.rest.utils.extractor.value.exception.OnlyOneValueExtractorException;
import org.ow2.petals.binding.rest.utils.extractor.value.exception.UnsupportedValueExtractorException;
import org.ow2.petals.binding.rest.utils.extractor.value.exception.ValueExtractorConfigException;
import org.ow2.petals.binding.rest.utils.extractor.value.exception.ValueExtractorRequiredException;
import org.w3c.dom.Element;
import org.w3c.dom.NodeList;
public class HttpHeadersBuilder {
public static final String HEADER_XML_TAG_NAME = "header";
public static final String HEADER_NAME_XML_ATTR_NAME = "name";
private HttpHeadersBuilder() {
// Utility class --> No constructor
}
/**
* Build HTTP headers from the given XML parent element
*
* @param parentElt
* The XML parent element containing HTTP header definitions
* @param xPathEvaluator
* @param componentPlaceholders
* @param logger
* @return List of HTTP headers. Not {@code null}. Can be empty.
*/
public static Map build(final Element parentElt, final XPath xPathEvaluator,
final Properties componentPlaceholders, final Logger logger) {
final Map headers = new HashMap<>();
final String namespaceURI = parentElt.getNamespaceURI();
final NodeList headerElements = parentElt.getElementsByTagNameNS(namespaceURI, HEADER_XML_TAG_NAME);
int headerElementsNb = headerElements.getLength();
for (int i = 0; i < headerElementsNb; i++) {
final Element headerElt = (Element) headerElements.item(i);
final String headerName = headerElt.getAttribute(HEADER_NAME_XML_ATTR_NAME);
try {
final XMLPayloadValueExtractor valueExtractor = ValueExtractorBuilder.build(headerElt, xPathEvaluator,
componentPlaceholders, logger);
headers.put(headerName, valueExtractor);
} catch (final OnlyOneValueExtractorException e) {
logger.warning(
String.format("Only one value extractor for header '%s'. Header skipped !!", headerName));
} catch (final ValueExtractorRequiredException e) {
logger.warning(
String.format("A value extractor is required for header '%s'. Header skipped !!", headerName));
} catch (final UnsupportedValueExtractorException e) {
logger.warning(String.format("Unsupport value extractor '%s' for header '%s'. Header skipped !!",
e.getUnsupportedValueExtractor(), headerName));
} catch (final ValueExtractorConfigException e) {
logger.log(Level.WARNING,
String.format(
"Error checking configuration of the value extractor of header '%s'. Header skipped !!",
headerName),
e);
}
}
return headers;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy