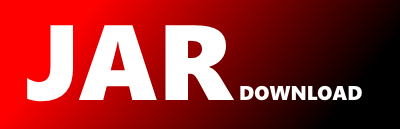
org.ow2.petals.bc.sftp.connection.SFTPConnectionInfo Maven / Gradle / Ivy
/**
* Copyright (c) 2009-2012 EBM WebSourcing, 2012-2016 Linagora
*
* This program/library is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 2.1 of the License, or (at your
* option) any later version.
*
* This program/library is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License
* for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program/library; If not, see http://www.gnu.org/licenses/
* for the GNU Lesser General Public License version 2.1.
*/
package org.ow2.petals.bc.sftp.connection;
import org.ow2.petals.bc.sftp.authentication.SshAuthentication;
import org.ow2.petals.bc.sftp.connection.exception.AuthenticationMissingException;
import org.ow2.petals.bc.sftp.connection.exception.ConnectionException;
import org.ow2.petals.bc.sftp.connection.exception.InvalidPortException;
import org.ow2.petals.bc.sftp.connection.exception.ServerMissingException;
import com.ebmwebsourcing.easycommons.lang.StringHelper;
/**
* Holds the SFTP connection parameters given in the service unit / message exchange.
* @author Charles Casadei - EBM WebSourcing
*/
public class SFTPConnectionInfo {
/**
* Default SSH port.
*/
public static final int DEFAULT_SSH_PORT = 22;
/**
* Working directory for the current session.
*/
private final String directory;
/**
* Specified the maximum number of simultaneous connection.
*/
private int maxConnection;
/**
* Max idle time of the connection
*/
private long maxIdleTime;
/**
* Define if an existing file on remote server have to be overwritten
*/
private final boolean overwrite;
/**
* Port.
*/
private final int port;
/**
* Address of the SFTP server.
*/
private final String server;
/**
* Holds the authentication informations.
*/
private final SshAuthentication sshAuthentication;
public SFTPConnectionInfo(final String server, final String port,
final SshAuthentication sshAuthentication, final String directory,
final boolean overwrite) throws ConnectionException {
this.server = server;
// For the SSH port
try {
this.port = (!StringHelper.isNullOrEmpty(port)) ? Integer.parseInt(port)
: DEFAULT_SSH_PORT;
} catch (final NumberFormatException ex) {
throw new InvalidPortException();
}
this.sshAuthentication = sshAuthentication;
this.directory = directory;
this.overwrite = overwrite;
// Validates the current configuration
this.validate();
}
/**
* Accessor on the current working directory.
*
* @return the current working directory.
*/
public final String getDirectory() {
return directory;
}
/**
* Builds a key identifying this connection.
*
* @return a key identifying this connection.
*/
public final String getKey() {
return this.server + this.port + this.sshAuthentication.getKey() + this.directory;
}
public int getMaxConnection() {
return maxConnection;
}
public long getMaxIdleTime() {
return maxIdleTime;
}
/**
* Accessor on the port used by the SFTP server.
*
* @return the port used by the SFTP server
*/
public final int getPort() {
return port;
}
/**
* Accessor on the host of the SFTP server.
*
* @return the host of the SFTP server
*/
public final String getServer() {
return server;
}
/**
* Accessor on the authentication used for the connection on the SSH server.
*
* @return the authentication used by the SSH server
*/
public final SshAuthentication getSshAuthentication() {
return this.sshAuthentication;
}
public void setMaxConnection(int maxConnection) {
this.maxConnection = maxConnection;
}
public void setMaxIdleTime(long maxIdleTime) {
this.maxIdleTime = maxIdleTime;
}
/**
* Returns a String with containing the values stored within an instance of
* this class.
*
* @return a String with containing the values stored within an instance of
* this class
*/
@Override
public final String toString() {
return "SFTP Connection Info : server=" + this.server + "; port=" + this.port + "; user="
+ this.getSshAuthentication().getUser() + "; directory=" + this.directory;
}
protected boolean isOverwrite() {
return overwrite;
}
/**
* Checks if the SU configuration seems to be valid or not.
*/
private final void validate() throws ConnectionException {
// Checks if the host is properly defined
if (StringHelper.isNullOrEmpty(server)) {
throw new ServerMissingException();
}
// Checks if the port seems to be valid
if (this.port < 0) {
throw new ConnectionException(
"The specified port is not valid. Port value range is 0-65535");
}
// Checks if there is any authentication defined
if (this.sshAuthentication == null) {
throw new AuthenticationMissingException();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy