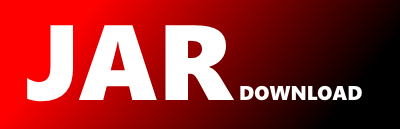
org.ow2.petals.bc.sftp.connection.WrappedSftpClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of petals-bc-sftp Show documentation
Show all versions of petals-bc-sftp Show documentation
A binding component used to transfer files using secure ftp
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy